在Python中,可以通过字符串的替换方法来实现用逗号代替空格。具体方法是使用字符串的replace()函数、split()和join()方法。下面将详细介绍这些方法,并提供示例代码来说明如何实现这一操作。
一、使用replace()函数:
Python的replace()函数可以用来替换字符串中的子字符串。通过replace()函数,可以将字符串中的空格替换为逗号。这是最简单直接的方法。
original_string = "This is a sample string"
modified_string = original_string.replace(" ", ",")
print(modified_string) # Output: This,is,a,sample,string
replace()函数的语法是:str.replace(old, new, count),其中old是需要替换的子字符串,new是新的子字符串,count是可选参数,表示替换的次数。如果不指定count,则会替换所有出现的子字符串。
二、使用split()和join()方法:
split()方法可以将字符串按指定的分隔符进行分割,返回一个列表;join()方法可以将列表中的元素连接成一个字符串,使用指定的分隔符。结合这两个方法,可以实现用逗号代替空格。
original_string = "This is another example string"
split_string = original_string.split(" ") # 将字符串按空格分割成列表
modified_string = ",".join(split_string) # 用逗号连接列表中的元素
print(modified_string) # Output: This,is,another,example,string
split()方法的语法是:str.split(separator, maxsplit),其中separator是分隔符,maxsplit是可选参数,表示分割的次数。如果不指定separator,默认按空格分割;如果不指定maxsplit,则会分割所有出现的分隔符。
三、使用正则表达式:
在一些复杂的情况下,可以使用正则表达式来替换空格。Python的re模块提供了sub()函数,可以用来替换字符串中的子字符串。
import re
original_string = "This is a more complex example string"
modified_string = re.sub(r"\s+", ",", original_string) # 用逗号替换一个或多个空格
print(modified_string) # Output: This,is,a,more,complex,example,string
sub()函数的语法是:re.sub(pattern, repl, string, count=0, flags=0),其中pattern是正则表达式,repl是新的子字符串,string是要处理的字符串,count是可选参数,表示替换的次数,flags是可选参数,表示匹配模式。
四、不同方法的比较:
- replace()方法适用于简单的替换操作,代码简洁直观,但只能替换固定的子字符串。
- split()和join()方法可以灵活处理各种分隔符,适用于需要进一步处理字符串的情况。
- 正则表达式方法适用于复杂的替换操作,可以处理各种复杂的模式匹配,但代码相对复杂。
五、实际应用中的注意事项:
- 当处理包含多个空格的字符串时,使用正则表达式方法可以更有效地替换所有空格。
- 在处理大文本或文件时,考虑使用高效的字符串操作方法,以提升性能。
- 如果需要替换的内容包含特殊字符,注意转义字符的使用。
通过以上几种方法,可以在Python中灵活地实现用逗号代替空格的操作。根据具体需求选择合适的方法,能够提高代码的可读性和执行效率。
六、示例代码解析:
以下是一个综合示例,展示了如何在实际应用中使用上述方法来替换字符串中的空格。
import re
def replace_space_with_comma(input_string):
# 方法一:使用replace()函数
method1_result = input_string.replace(" ", ",")
# 方法二:使用split()和join()方法
split_string = input_string.split(" ")
method2_result = ",".join(split_string)
# 方法三:使用正则表达式
method3_result = re.sub(r"\s+", ",", input_string)
return method1_result, method2_result, method3_result
测试字符串
test_string = "This is a test string with multiple spaces"
调用函数,获取结果
result1, result2, result3 = replace_space_with_comma(test_string)
输出结果
print("Method 1 Result:", result1) # Output: This,is,a,test,string,with,multiple,spaces
print("Method 2 Result:", result2) # Output: This,is,a,test,string,with,multiple,spaces
print("Method 3 Result:", result3) # Output: This,is,a,test,string,with,multiple,spaces
通过这个示例代码,可以看到不同方法的应用效果。根据具体需求选择合适的方法,可以提高代码的可读性和执行效率。希望通过本文的介绍,能够帮助读者更好地理解和掌握在Python中用逗号代替空格的操作。
相关问答FAQs:
如何在Python中将字符串中的空格替换为逗号?
在Python中,可以使用字符串的replace()
方法轻松地将空格替换为逗号。例如,my_string = "这是 一个 示例"; new_string = my_string.replace(" ", ",")
将会得到"这是,一个,示例"
。
使用正则表达式是否可以更灵活地替换空格?
是的,正则表达式提供了更强大的匹配功能。可以使用re
模块来实现更复杂的替换。例如,import re; new_string = re.sub(r'\s+', ',', my_string)
可以将多个空格或制表符替换为一个逗号。
如何处理字符串开头和结尾的空格?
在替换空格时,可以使用strip()
方法去除字符串开头和结尾的空格。结合使用replace()
方法,代码示例为new_string = my_string.strip().replace(" ", ",")
,这样可以确保处理后的字符串不会在开头或结尾留下多余的逗号。
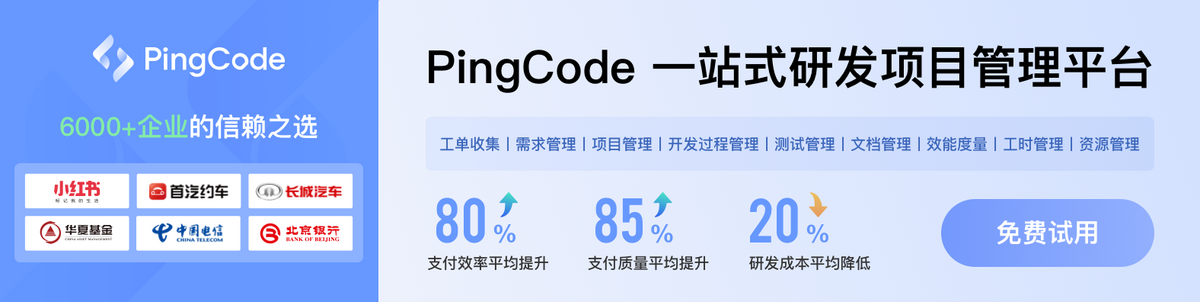