Python获取信息中的文本的主要方法有:使用正则表达式、使用字符串方法、使用BeautifulSoup库、使用NLTK库。 在这篇文章中,我们将详细讨论这四种方法,尤其是如何使用正则表达式来提取文本信息。
一、正则表达式
正则表达式是一种强大的文本处理工具,适用于查找、匹配和提取字符串中的特定模式。Python的re
模块提供了对正则表达式的支持。
1、基本语法与方法
正则表达式的基本语法包括字符集、量词、边界、分组等。re
模块中的常用方法有:
re.match(pattern, string)
: 从字符串的起始位置开始匹配,匹配成功返回Match对象,否则返回None。re.search(pattern, string)
: 搜索整个字符串,匹配成功返回Match对象,否则返回None。re.findall(pattern, string)
: 返回字符串中所有非重叠的匹配。re.sub(pattern, repl, string)
: 使用一个不同的字符串替换匹配到的模式。
2、示例代码
import re
示例文本
text = "My phone number is 123-456-7890 and my email is example@test.com"
提取电话号码
phone_pattern = r'\d{3}-\d{3}-\d{4}'
phone_number = re.search(phone_pattern, text)
if phone_number:
print("Phone number found:", phone_number.group())
提取邮箱地址
email_pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
email_address = re.search(email_pattern, text)
if email_address:
print("Email address found:", email_address.group())
二、使用字符串方法
Python内置的字符串方法也可以用于提取文本信息,如split()
, find()
, index()
, replace()
等。
1、基本方法
split()
: 将字符串按照指定的分隔符拆分为一个列表。find()
: 返回子字符串在字符串中的最低索引,如果没有找到返回-1。index()
: 与find()
类似,但如果没有找到会引发ValueError。replace()
: 替换字符串中的旧子字符串为新的子字符串。
2、示例代码
# 示例文本
text = "Hello, my name is John Doe. I live in New York."
提取姓名
start = text.find("name is") + len("name is ")
end = text.find(".", start)
name = text[start:end].strip()
print("Name found:", name)
提取城市
start = text.find("in") + len("in ")
city = text[start:].strip(".")
print("City found:", city)
三、使用BeautifulSoup库
BeautifulSoup是一个用于解析HTML和XML文档的库,常用于从网页中提取数据。
1、安装与导入
首先需要安装BeautifulSoup库,可以使用以下命令:
pip install beautifulsoup4
导入BeautifulSoup库:
from bs4 import BeautifulSoup
import requests
2、示例代码
# 示例网页
url = "https://example.com"
获取网页内容
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
提取标题
title = soup.title.string
print("Title found:", title)
提取所有段落文本
paragraphs = soup.find_all('p')
for p in paragraphs:
print("Paragraph found:", p.get_text())
四、使用NLTK库
NLTK(Natural Language Toolkit)是一个用于处理自然语言文本的库,适用于更复杂的文本分析任务。
1、安装与导入
首先需要安装NLTK库,可以使用以下命令:
pip install nltk
导入NLTK库:
import nltk
from nltk.tokenize import word_tokenize, sent_tokenize
from nltk.corpus import stopwords
2、示例代码
# 示例文本
text = "Hello, my name is John Doe. I live in New York. I love programming in Python."
分词
words = word_tokenize(text)
print("Words:", words)
句子分割
sentences = sent_tokenize(text)
print("Sentences:", sentences)
去除停用词
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word.lower() not in stop_words]
print("Filtered Words:", filtered_words)
结论
Python提供了多种方法来获取信息中的文本,包括正则表达式、字符串方法、BeautifulSoup库和NLTK库。每种方法都有其独特的优点和适用场景。正则表达式适用于复杂模式匹配、字符串方法适用于简单的文本操作、BeautifulSoup库适用于从网页中提取数据、NLTK库适用于自然语言处理。 根据具体需求选择适当的方法,可以更高效地进行文本信息的提取和处理。
相关问答FAQs:
如何在Python中提取特定文本信息?
在Python中提取特定文本信息可以通过多种方式实现,例如使用字符串方法、正则表达式或文本处理库。对于简单的文本提取,可以使用str.find()
或str.split()
方法。对于复杂的模式匹配和提取,re
模块(正则表达式)将非常有用。使用这些工具,你可以根据需求定位和提取所需的文本片段。
Python有哪些库可以帮助我处理文本信息?
处理文本信息的库有很多,比较常用的包括re
(正则表达式),BeautifulSoup
(用于解析HTML和XML文档),pandas
(用于数据分析和处理),以及nltk
和spaCy
(用于自然语言处理)。这些库各自有不同的功能,可以帮助你以不同的方式提取和分析文本。
如何处理包含多种格式的文本信息?
在处理包含多种格式的文本信息时,可以使用Python的str
方法和正则表达式的组合。对于结构化数据,如JSON或CSV格式,可以使用json
或pandas
库轻松解析。对于非结构化文本,使用nltk
或spaCy
等自然语言处理库可以帮助你提取有用的信息并进行分析。确保在提取时考虑到文本的格式,以获得最佳结果。
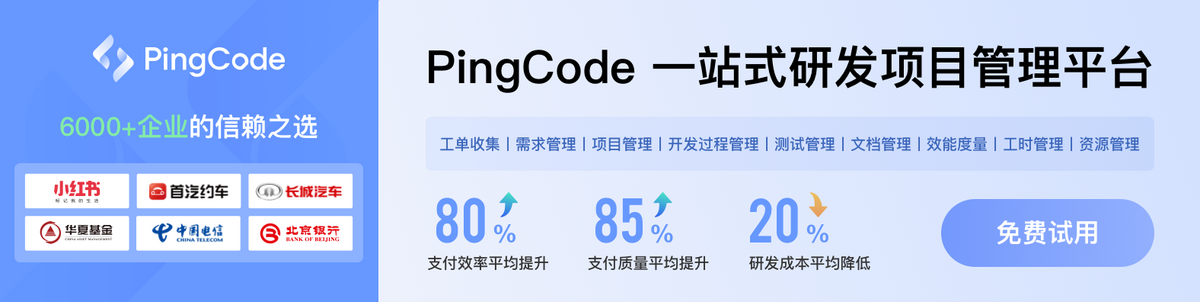