在Python中,使用Matplotlib库可以很方便地绘制散点图并添加标签。要给散点图中的数据点添加标签,可以使用annotate
函数。最常用的方法是通过循环遍历数据点,并为每个点添加标签。
下面详细讲解如何在Python中绘制散点图并添加标签:
一、导入所需的库
首先,需要导入绘制散点图所需的库。Matplotlib
是Python中最常用的绘图库,而NumPy
则用于生成随机数据。
import matplotlib.pyplot as plt
import numpy as np
二、生成数据
接下来,生成一些示例数据。可以使用NumPy
生成随机数据点,这些数据点将被用来绘制散点图。
# 生成随机数据
np.random.seed(0)
x = np.random.rand(10)
y = np.random.rand(10)
labels = [f'Point {i}' for i in range(10)] # 数据点的标签
三、绘制散点图
使用plt.scatter
函数绘制散点图。
# 绘制散点图
plt.scatter(x, y)
四、添加标签
通过循环遍历每个数据点,并使用annotate
函数为每个点添加标签。
# 添加标签
for i, label in enumerate(labels):
plt.annotate(label, (x[i], y[i]))
五、显示图形
最后,使用plt.show()
函数显示图形。
# 显示图形
plt.show()
完整的代码如下:
import matplotlib.pyplot as plt
import numpy as np
生成随机数据
np.random.seed(0)
x = np.random.rand(10)
y = np.random.rand(10)
labels = [f'Point {i}' for i in range(10)]
绘制散点图
plt.scatter(x, y)
添加标签
for i, label in enumerate(labels):
plt.annotate(label, (x[i], y[i]))
显示图形
plt.show()
通过上述步骤,可以在Python中绘制带有标签的散点图。接下来,我们深入探讨散点图和标签的更多细节和技巧。
一、使用不同的标签样式
在添加标签时,可以自定义标签的样式,使其更具可读性。例如,可以设置字体大小、颜色、对齐方式等。
for i, label in enumerate(labels):
plt.annotate(label, (x[i], y[i]), fontsize=10, color='red', ha='right')
在上述代码中,fontsize
设置标签的字体大小,color
设置标签的颜色,ha
设置标签的水平对齐方式。
二、避免标签重叠
在实际应用中,数据点可能会非常接近,导致标签重叠。可以使用offset
参数来稍微移动标签的位置,以避免重叠。
for i, label in enumerate(labels):
plt.annotate(label, (x[i], y[i]), fontsize=10, color='red', ha='right',
xytext=(5, 5), textcoords='offset points')
其中,xytext
设置标签相对于数据点的位置偏移量,textcoords
设置偏移量的坐标系。
三、使用其他库(如Seaborn)绘制散点图并添加标签
除了Matplotlib
,Seaborn
也是一个常用的绘图库,基于Matplotlib
,提供更高级的接口和美观的默认样式。
import seaborn as sns
生成随机数据
np.random.seed(0)
x = np.random.rand(10)
y = np.random.rand(10)
labels = [f'Point {i}' for i in range(10)]
创建DataFrame
import pandas as pd
df = pd.DataFrame({'x': x, 'y': y, 'label': labels})
绘制散点图
sns.scatterplot(data=df, x='x', y='y')
添加标签
for line in range(0, df.shape[0]):
plt.text(df.x[line], df.y[line], df.label[line], horizontalalignment='left', size='medium', color='black', weight='semibold')
显示图形
plt.show()
四、与其他图表结合
有时候,散点图可能需要与其他类型的图表结合使用,以便更好地展示数据。例如,可以将散点图与折线图结合,或者在散点图上叠加密度图。
# 生成随机数据
np.random.seed(0)
x = np.random.rand(10)
y = np.random.rand(10)
labels = [f'Point {i}' for i in range(10)]
绘制散点图
plt.scatter(x, y, label='Data Points')
绘制折线图
plt.plot(x, y, linestyle='--', color='gray', label='Connecting Line')
添加标签
for i, label in enumerate(labels):
plt.annotate(label, (x[i], y[i]), fontsize=10, color='red', ha='right',
xytext=(5, 5), textcoords='offset points')
显示图例
plt.legend()
显示图形
plt.show()
五、绘制三维散点图并添加标签
有时,需要绘制三维散点图。Matplotlib
中的Axes3D
模块可以帮助实现三维散点图,并添加标签。
from mpl_toolkits.mplot3d import Axes3D
生成随机数据
np.random.seed(0)
x = np.random.rand(10)
y = np.random.rand(10)
z = np.random.rand(10)
labels = [f'Point {i}' for i in range(10)]
创建三维图形
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
绘制三维散点图
ax.scatter(x, y, z)
添加标签
for i, label in enumerate(labels):
ax.text(x[i], y[i], z[i], label, fontsize=10, color='red')
显示图形
plt.show()
综上所述,通过以上步骤,您可以在Python中使用Matplotlib和其他库绘制带有标签的散点图,并根据需要自定义标签的样式和位置。此外,您还可以将散点图与其他类型的图表结合使用,或者绘制三维散点图,以更好地展示数据。通过不断练习和探索,您将能够熟练掌握这些技巧,并在数据可视化中创造更具吸引力和信息丰富的图表。
相关问答FAQs:
如何在Python的散点图中添加个别点的标签?
在使用Matplotlib库绘制散点图时,可以通过plt.text()
或ax.text()
函数为每个数据点添加标签。您需要指定标签的位置和内容。例如,您可以在循环中为每个点添加标签,代码示例如下:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
labels = ['A', 'B', 'C', 'D', 'E']
plt.scatter(x, y)
for i in range(len(labels)):
plt.text(x[i], y[i], labels[i], fontsize=9, ha='right')
plt.show()
这样,每个点旁边都会显示相应的标签。
在散点图中如何自定义标签的样式和位置?
使用plt.text()
或ax.text()
时,可以通过参数调整标签的字体大小、颜色、对齐方式等。例如,可以使用fontsize
、color
、ha
(水平对齐)和va
(垂直对齐)等参数来个性化标签的样式。以下是一个示例:
plt.text(x[i], y[i], labels[i], fontsize=12, color='red', ha='center', va='bottom')
这将使标签更加醒目并且具有良好的视觉效果。
如何在散点图中添加总标题和坐标轴标签?
为了使散点图更具可读性,可以添加图表的总标题和坐标轴标签。使用plt.title()
、plt.xlabel()
和plt.ylabel()
函数可以轻松实现。例如:
plt.title('散点图示例')
plt.xlabel('X 轴标签')
plt.ylabel('Y 轴标签')
这些元素将帮助观众更好地理解图表内容和数据分布。
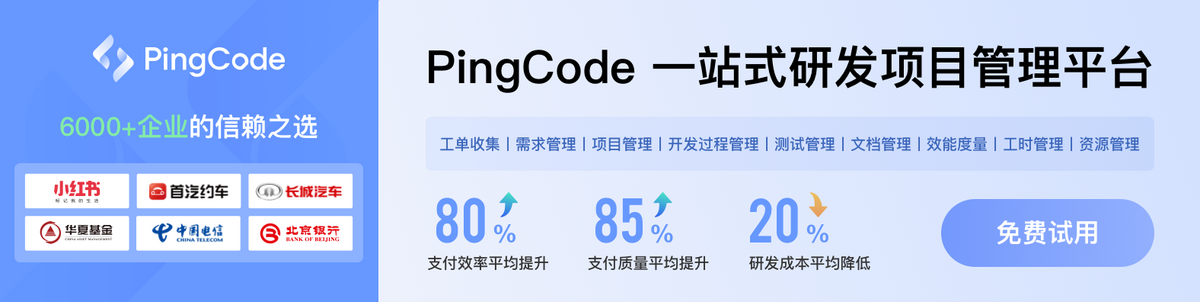