在Python中,访问父类的属性可以通过以下几种方法:使用super()
函数、直接通过类名访问、在子类的初始化方法中调用父类的初始化方法。其中,最推荐的方法是使用super()
函数,因为它不仅可以简化代码,还可以避免多重继承带来的潜在问题。下面我们将详细介绍这几种方法,并通过具体的代码示例来说明它们的使用方式。
一、使用super()
函数
使用super()
函数是访问父类属性和方法的推荐方法。super()
函数返回了一个父类的对象,可以方便地调用父类的方法和属性。
class Parent:
def __init__(self):
self.parent_attribute = "I am a parent attribute"
class Child(Parent):
def __init__(self):
super().__init__() # 调用父类的初始化方法
self.child_attribute = "I am a child attribute"
def get_parent_attribute(self):
return super().parent_attribute # 通过super()访问父类的属性
child = Child()
print(child.get_parent_attribute()) # 输出: I am a parent attribute
在上述代码中,子类Child
通过super().__init__()
调用了父类Parent
的初始化方法,从而继承了父类的属性parent_attribute
。随后,通过super().parent_attribute
访问了父类的属性。
二、直接通过类名访问
除了使用super()
函数外,还可以直接通过类名访问父类的属性。这种方法在某些特定场景下也非常有用。
class Parent:
def __init__(self):
self.parent_attribute = "I am a parent attribute"
class Child(Parent):
def __init__(self):
Parent.__init__(self) # 调用父类的初始化方法
self.child_attribute = "I am a child attribute"
def get_parent_attribute(self):
return Parent.parent_attribute
child = Child()
print(child.get_parent_attribute()) # 输出: I am a parent attribute
在这个例子中,子类Child
通过Parent.__init__(self)
调用了父类Parent
的初始化方法,并通过Parent.parent_attribute
访问了父类的属性。
三、在子类的初始化方法中调用父类的初始化方法
在子类的初始化方法中,显式调用父类的初始化方法也是一种常见的做法。这种方法可以确保子类在初始化时正确地继承父类的属性和方法。
class Parent:
def __init__(self):
self.parent_attribute = "I am a parent attribute"
class Child(Parent):
def __init__(self):
Parent.__init__(self) # 调用父类的初始化方法
self.child_attribute = "I am a child attribute"
def get_parent_attribute(self):
return self.parent_attribute # 直接访问父类的属性
child = Child()
print(child.get_parent_attribute()) # 输出: I am a parent attribute
在这个例子中,子类Child
通过Parent.__init__(self)
调用了父类Parent
的初始化方法,从而继承了父类的属性parent_attribute
。随后,通过self.parent_attribute
直接访问了父类的属性。
详细解析
一、使用super()
函数
1. 调用父类的初始化方法
在子类中使用super().__init__()
,可以确保父类的初始化方法被正确调用,从而继承父类的属性和方法。这在多重继承的情况下尤其重要,因为super()
函数可以按照继承链顺序调用所有父类的初始化方法。
class Parent1:
def __init__(self):
self.parent1_attribute = "I am Parent1 attribute"
class Parent2:
def __init__(self):
self.parent2_attribute = "I am Parent2 attribute"
class Child(Parent1, Parent2):
def __init__(self):
super().__init__()
self.child_attribute = "I am a child attribute"
child = Child()
print(child.parent1_attribute) # 输出: I am Parent1 attribute
print(child.parent2_attribute) # 输出: I am Parent2 attribute
在这个例子中,子类Child
通过super().__init__()
调用了父类Parent1
的初始化方法,从而继承了Parent1
的属性parent1_attribute
。由于super()
函数按照继承链顺序调用父类的初始化方法,因此Parent2
的初始化方法也被调用了,继承了Parent2
的属性parent2_attribute
。
2. 调用父类的方法
除了初始化方法外,super()
函数还可以用于调用父类的其他方法。这样可以简化代码,并避免多重继承中的潜在问题。
class Parent:
def __init__(self):
self.parent_attribute = "I am a parent attribute"
def parent_method(self):
return "This is a method from parent"
class Child(Parent):
def __init__(self):
super().__init__()
self.child_attribute = "I am a child attribute"
def child_method(self):
parent_method_result = super().parent_method()
return f"Child method calls: {parent_method_result}"
child = Child()
print(child.child_method()) # 输出: Child method calls: This is a method from parent
在这个例子中,子类Child
通过super().parent_method()
调用了父类Parent
的方法parent_method
,并在child_method
中返回了父类方法的结果。
二、直接通过类名访问
直接通过类名访问父类的属性和方法,可以在某些特定场景下非常有用,尤其是在多重继承的情况下,明确指定要调用的父类方法或属性。
1. 访问父类的属性
通过类名直接访问父类的属性,可以清晰地表达意图,但需要注意这种方式可能在多重继承中带来复杂性。
class Parent:
def __init__(self):
self.parent_attribute = "I am a parent attribute"
class Child(Parent):
def __init__(self):
Parent.__init__(self)
self.child_attribute = "I am a child attribute"
def get_parent_attribute(self):
return Parent.parent_attribute
child = Child()
print(child.get_parent_attribute()) # 输出: I am a parent attribute
2. 调用父类的方法
直接通过类名调用父类的方法,可以在多重继承中避免潜在的冲突和混淆。
class Parent1:
def parent1_method(self):
return "This is a method from Parent1"
class Parent2:
def parent2_method(self):
return "This is a method from Parent2"
class Child(Parent1, Parent2):
def child_method(self):
return Parent1.parent1_method(self)
child = Child()
print(child.child_method()) # 输出: This is a method from Parent1
在这个例子中,子类Child
通过Parent1.parent1_method(self)
明确指定调用Parent1
的方法,避免了多重继承中可能的混淆。
三、在子类的初始化方法中调用父类的初始化方法
在子类的初始化方法中显式调用父类的初始化方法,可以确保子类在初始化时正确继承父类的属性和方法。这种方法在某些特定场景下也非常有用。
1. 继承父类的属性
显式调用父类的初始化方法,可以确保子类继承父类的属性,并在子类中进行扩展。
class Parent:
def __init__(self):
self.parent_attribute = "I am a parent attribute"
class Child(Parent):
def __init__(self):
Parent.__init__(self)
self.child_attribute = "I am a child attribute"
def get_parent_attribute(self):
return self.parent_attribute
child = Child()
print(child.get_parent_attribute()) # 输出: I am a parent attribute
2. 调用父类的方法
显式调用父类的方法,可以在子类中重用父类的方法逻辑,并在子类中进行扩展。
class Parent:
def parent_method(self):
return "This is a method from parent"
class Child(Parent):
def child_method(self):
return self.parent_method()
child = Child()
print(child.child_method()) # 输出: This is a method from parent
在这个例子中,子类Child
通过self.parent_method()
调用了父类Parent
的方法parent_method
,并在子类方法中返回了父类方法的结果。
总结
在Python中,访问父类的属性和方法可以通过多种方法实现,其中最推荐的方法是使用super()
函数。super()
函数不仅可以简化代码,还可以避免多重继承中的潜在问题。此外,直接通过类名访问和显式调用父类的初始化方法也是常见的做法,但需要根据具体场景选择合适的方法。通过上述方法,可以在子类中方便地继承和扩展父类的属性和方法,提高代码的可维护性和可读性。
相关问答FAQs:
在Python中,如何访问父类的私有属性?
虽然私有属性不能直接通过子类访问,但可以通过父类的方法来间接获取。可以在父类中定义一个公共或受保护的方法,用于返回私有属性的值。例如:
class Parent:
def __init__(self):
self.__private_attr = "I'm private"
def get_private_attr(self):
return self.__private_attr
class Child(Parent):
def show_private_attr(self):
return self.get_private_attr()
child = Child()
print(child.show_private_attr()) # 输出: I'm private
通过这种方式,子类可以安全地访问父类的私有属性。
子类如何重写父类的属性?
在Python中,子类可以通过直接赋值的方式重写父类的属性。这意味着如果子类和父类有同名属性,子类的属性将会覆盖父类的属性。示例代码如下:
class Parent:
attr = "I am from Parent"
class Child(Parent):
attr = "I am from Child"
print(Child.attr) # 输出: I am from Child
print(Parent.attr) # 输出: I am from Parent
这种机制使得子类能够根据需要定义自己的属性。
如何在子类中调用父类的属性?
在子类中调用父类的属性,可以使用super()
函数或直接通过父类名来访问。以下是两种方法的示例:
class Parent:
attr = "I am from Parent"
class Child(Parent):
def show_attr(self):
return super().attr # 使用super()
# 或者直接通过父类名访问
class Child2(Parent):
def show_attr(self):
return Parent.attr # 使用父类名
child = Child()
print(child.show_attr()) # 输出: I am from Parent
child2 = Child2()
print(child2.show_attr()) # 输出: I am from Parent
通过这两种方式,子类可以轻松访问和使用父类的属性。
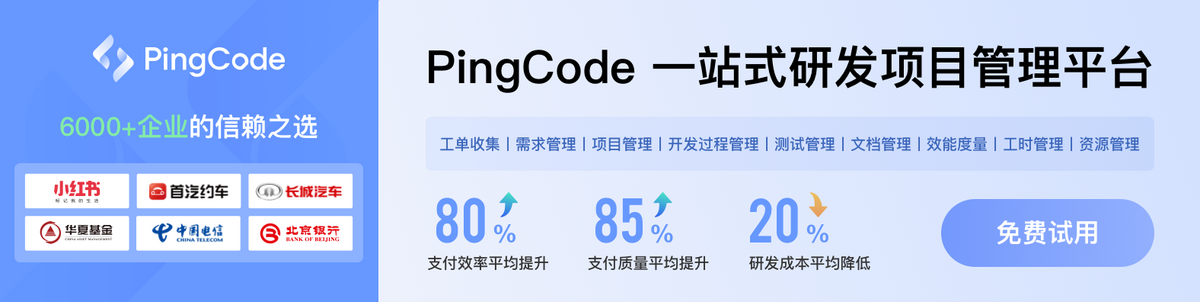