在Python中,使用GUI参数定义类的方法包括:使用Tkinter、PyQt、Kivy等库。其中,Tkinter是Python的标准GUI库,它提供了简单的接口来创建图形用户界面。通过定义类,我们可以将GUI组件和功能封装在一起,便于代码的组织和重用。本文将详细介绍如何使用Tkinter在Python中定义一个包含GUI参数的类。
一、Tkinter简介
Tkinter是Python的标准GUI库,提供了一组完整的接口来创建图形用户界面。它是基于Tcl/Tk的一个薄层封装,易于使用且跨平台。Tkinter的主要组件包括窗口、按钮、标签、文本框等,它们可以通过类和方法进行创建和管理。
二、创建基础GUI窗口
在开始定义类之前,我们需要了解如何创建一个基础的Tkinter窗口。以下是一个简单的示例代码:
import tkinter as tk
创建主窗口
root = tk.Tk()
root.title("基础窗口")
root.geometry("300x200")
运行主循环
root.mainloop()
以上代码创建了一个标题为“基础窗口”,大小为300×200像素的窗口,并运行主循环来保持窗口的打开状态。
三、定义包含GUI参数的类
通过定义类,我们可以将GUI组件和功能封装在一起,使代码更加模块化和易于维护。以下是一个示例,展示如何定义一个包含GUI参数的类:
import tkinter as tk
class MyApp:
def __init__(self, root):
self.root = root
self.root.title("My Application")
self.root.geometry("400x300")
self.label = tk.Label(self.root, text="Hello, Tkinter!")
self.label.pack(pady=20)
self.button = tk.Button(self.root, text="Click Me", command=self.on_button_click)
self.button.pack(pady=10)
self.entry = tk.Entry(self.root)
self.entry.pack(pady=10)
def on_button_click(self):
user_input = self.entry.get()
self.label.config(text=f"Hello, {user_input}!")
创建主窗口
root = tk.Tk()
创建应用实例
app = MyApp(root)
运行主循环
root.mainloop()
在上述代码中,我们定义了一个名为MyApp
的类,用于封装GUI组件和功能。__init__
方法用于初始化类实例,并创建标签、按钮和文本框等组件。on_button_click
方法则定义了按钮点击事件的处理逻辑。
四、添加更多GUI组件和功能
为了使我们的应用程序更加丰富,我们可以添加更多的GUI组件和功能。例如,我们可以添加一个复选框和一个单选按钮,以实现更多交互功能:
import tkinter as tk
class MyApp:
def __init__(self, root):
self.root = root
self.root.title("My Application")
self.root.geometry("500x400")
self.label = tk.Label(self.root, text="Hello, Tkinter!")
self.label.pack(pady=20)
self.button = tk.Button(self.root, text="Click Me", command=self.on_button_click)
self.button.pack(pady=10)
self.entry = tk.Entry(self.root)
self.entry.pack(pady=10)
self.checkbox_var = tk.IntVar()
self.checkbox = tk.Checkbutton(self.root, text="Check me", variable=self.checkbox_var)
self.checkbox.pack(pady=10)
self.radio_var = tk.StringVar()
self.radio1 = tk.Radiobutton(self.root, text="Option 1", value="1", variable=self.radio_var)
self.radio2 = tk.Radiobutton(self.root, text="Option 2", value="2", variable=self.radio_var)
self.radio1.pack(pady=5)
self.radio2.pack(pady=5)
def on_button_click(self):
user_input = self.entry.get()
checkbox_state = "checked" if self.checkbox_var.get() else "unchecked"
selected_option = self.radio_var.get()
self.label.config(text=f"Hello, {user_input}! Checkbox is {checkbox_state}. Selected option is {selected_option}.")
创建主窗口
root = tk.Tk()
创建应用实例
app = MyApp(root)
运行主循环
root.mainloop()
在这个示例中,我们添加了一个复选框和两个单选按钮,并在on_button_click
方法中处理它们的状态。
五、使用Grid布局管理器
除了使用pack
布局管理器,我们还可以使用grid
布局管理器来更灵活地管理组件的布局。以下是一个示例,展示如何使用grid
布局管理器:
import tkinter as tk
class MyApp:
def __init__(self, root):
self.root = root
self.root.title("My Application")
self.root.geometry("400x300")
self.label = tk.Label(self.root, text="Hello, Tkinter!")
self.label.grid(row=0, column=0, columnspan=2, pady=20)
self.entry = tk.Entry(self.root)
self.entry.grid(row=1, column=0, pady=10)
self.button = tk.Button(self.root, text="Click Me", command=self.on_button_click)
self.button.grid(row=1, column=1, pady=10)
self.checkbox_var = tk.IntVar()
self.checkbox = tk.Checkbutton(self.root, text="Check me", variable=self.checkbox_var)
self.checkbox.grid(row=2, column=0, columnspan=2, pady=10)
self.radio_var = tk.StringVar()
self.radio1 = tk.Radiobutton(self.root, text="Option 1", value="1", variable=self.radio_var)
self.radio2 = tk.Radiobutton(self.root, text="Option 2", value="2", variable=self.radio_var)
self.radio1.grid(row=3, column=0, pady=5)
self.radio2.grid(row=3, column=1, pady=5)
def on_button_click(self):
user_input = self.entry.get()
checkbox_state = "checked" if self.checkbox_var.get() else "unchecked"
selected_option = self.radio_var.get()
self.label.config(text=f"Hello, {user_input}! Checkbox is {checkbox_state}. Selected option is {selected_option}.")
创建主窗口
root = tk.Tk()
创建应用实例
app = MyApp(root)
运行主循环
root.mainloop()
在这个示例中,我们使用grid
布局管理器将组件放置在窗口的网格中,通过指定行和列的位置来控制组件的布局。
六、添加菜单栏和对话框
为了使应用程序更加完整,我们可以添加菜单栏和对话框。例如,我们可以添加一个文件菜单,并实现打开文件和保存文件的功能:
import tkinter as tk
from tkinter import filedialog, messagebox
class MyApp:
def __init__(self, root):
self.root = root
self.root.title("My Application")
self.root.geometry("500x400")
self.create_menu()
self.label = tk.Label(self.root, text="Hello, Tkinter!")
self.label.pack(pady=20)
self.entry = tk.Entry(self.root)
self.entry.pack(pady=10)
self.button = tk.Button(self.root, text="Click Me", command=self.on_button_click)
self.button.pack(pady=10)
self.checkbox_var = tk.IntVar()
self.checkbox = tk.Checkbutton(self.root, text="Check me", variable=self.checkbox_var)
self.checkbox.pack(pady=10)
self.radio_var = tk.StringVar()
self.radio1 = tk.Radiobutton(self.root, text="Option 1", value="1", variable=self.radio_var)
self.radio2 = tk.Radiobutton(self.root, text="Option 2", value="2", variable=self.radio_var)
self.radio1.pack(pady=5)
self.radio2.pack(pady=5)
def create_menu(self):
menubar = tk.Menu(self.root)
filemenu = tk.Menu(menubar, tearoff=0)
filemenu.add_command(label="Open", command=self.open_file)
filemenu.add_command(label="Save", command=self.save_file)
filemenu.add_separator()
filemenu.add_command(label="Exit", command=self.root.quit)
menubar.add_cascade(label="File", menu=filemenu)
self.root.config(menu=menubar)
def open_file(self):
file_path = filedialog.askopenfilename()
if file_path:
with open(file_path, 'r') as file:
content = file.read()
messagebox.showinfo("File Content", content)
def save_file(self):
file_path = filedialog.asksaveasfilename()
if file_path:
with open(file_path, 'w') as file:
file.write(self.entry.get())
messagebox.showinfo("Save File", "File saved successfully!")
def on_button_click(self):
user_input = self.entry.get()
checkbox_state = "checked" if self.checkbox_var.get() else "unchecked"
selected_option = self.radio_var.get()
self.label.config(text=f"Hello, {user_input}! Checkbox is {checkbox_state}. Selected option is {selected_option}.")
创建主窗口
root = tk.Tk()
创建应用实例
app = MyApp(root)
运行主循环
root.mainloop()
在这个示例中,我们添加了一个菜单栏,并实现了打开文件和保存文件的功能。通过filedialog
模块,我们可以弹出文件选择对话框,让用户选择文件进行打开或保存。
七、总结
通过本文的介绍,我们了解了如何在Python中使用Tkinter定义包含GUI参数的类。我们从基础窗口的创建开始,逐步添加了标签、按钮、文本框、复选框、单选按钮、菜单栏和对话框等组件,并通过定义类将这些组件和功能封装在一起。希望通过这篇文章,您能够掌握使用Tkinter创建图形用户界面的基本技巧,并能够创建出功能丰富的应用程序。
相关问答FAQs:
如何在Python GUI中使用类来管理用户输入的参数?
在Python的GUI应用程序中,使用类来管理用户输入的参数是一种有效的方式。首先,可以创建一个类来定义参数的属性和方法。然后,在GUI中创建相应的输入控件(如文本框、下拉菜单等),并将这些控件的值与类的属性绑定。这样,当用户输入数据时,可以通过类的实例来获取和处理这些参数。
在Python GUI中,我如何实现参数验证?
在实现参数输入时,确保数据的有效性至关重要。可以在类中定义验证方法,例如检查输入是否为空、格式是否正确、数值范围是否合理等。在用户提交数据时,调用这些验证方法,如果验证未通过,可以通过GUI显示错误信息,提示用户更正输入。
我可以使用哪些Python库来创建GUI并定义类?
有多种Python库可以用来创建GUI,如Tkinter、PyQt、wxPython等。Tkinter是内置库,适合简单应用;PyQt提供更丰富的控件和样式,适合复杂应用;wxPython则是另一个广受欢迎的选择,具有良好的平台兼容性。选择适合自己需求的库后,可以结合类的定义来管理参数输入和处理。
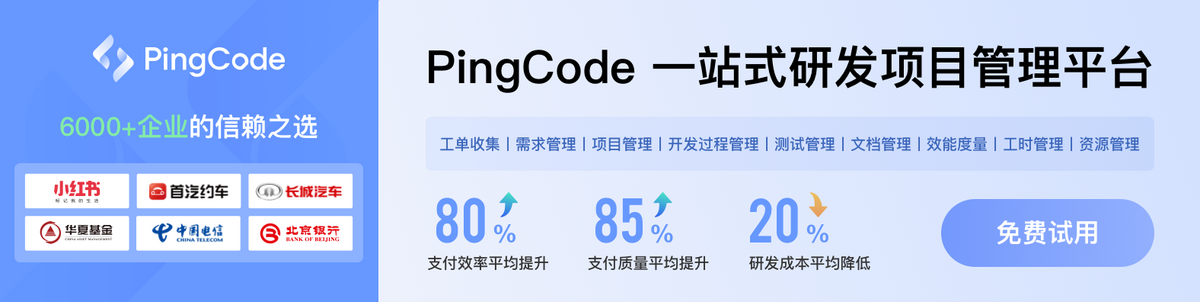