要对Python读取的txt文件进行统计,可以使用多种方法,包括读取文件内容、统计行数、单词数和字符数等。具体方法包括使用Python内置函数、正则表达式或第三方库。 其中,读取文件内容是最基础的一步,它为后续的统计操作提供了数据。接下来,我们会详细描述如何在Python中进行这些操作,以及一些高级的统计方法。
一、读取文件内容
在进行任何统计操作之前,首先需要读取文件内容。Python 提供了多种方式来读取文件内容,最常用的方法是使用 open()
函数。下面是一个示例代码,用来读取文本文件的内容:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
这段代码使用 with open
语句打开文件,并读取其内容。使用 with
语句可以确保文件在读取后自动关闭,避免资源泄露。
二、统计行数
统计行数是文本文件统计的基本操作之一。可以使用 readlines()
方法将文件内容读取为一个列表,每个元素表示文件中的一行,然后通过 len()
函数获取行数:
with open('example.txt', 'r', encoding='utf-8') as file:
lines = file.readlines()
line_count = len(lines)
print(f'Total number of lines: {line_count}')
这种方法非常简单明了,也可以直接通过迭代文件对象来统计行数:
line_count = 0
with open('example.txt', 'r', encoding='utf-8') as file:
for line in file:
line_count += 1
print(f'Total number of lines: {line_count}')
三、统计单词数
统计单词数是文本处理中的常见需求。可以使用 split()
方法将文本内容拆分为单词列表,然后获取其长度:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
words = content.split()
word_count = len(words)
print(f'Total number of words: {word_count}')
这种方法将文件内容按空白字符拆分为单词,对于大多数文本文件是足够的。如果需要更复杂的拆分规则,可以使用正则表达式:
import re
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
words = re.findall(r'\b\w+\b', content)
word_count = len(words)
print(f'Total number of words: {word_count}')
四、统计字符数
统计字符数相对简单,可以直接使用 len()
函数获取文件内容的长度:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
char_count = len(content)
print(f'Total number of characters: {char_count}')
如果需要统计不包括空白字符的字符数,可以使用 replace()
方法去除空白字符后再统计:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
content_no_space = content.replace(' ', '').replace('\n', '')
char_count = len(content_no_space)
print(f'Total number of characters (excluding spaces): {char_count}')
五、高级统计方法
除了基本的行数、单词数和字符数统计,还可以进行一些高级统计操作。例如,统计某个特定单词的出现次数,或者统计每个单词的频率。
- 统计特定单词的出现次数:
word_to_count = 'python'
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
word_count = content.lower().split().count(word_to_count)
print(f'Total number of occurrences of "{word_to_count}": {word_count}')
- 统计每个单词的频率:
from collections import Counter
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
words = content.lower().split()
word_freq = Counter(words)
print(f'Word frequencies: {word_freq}')
- 统计每行的字符数:
line_char_counts = []
with open('example.txt', 'r', encoding='utf-8') as file:
for line in file:
line_char_counts.append(len(line.strip()))
print(f'Character count per line: {line_char_counts}')
- 统计每段落的行数:
paragraph_line_counts = []
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
paragraphs = content.split('\n\n')
for paragraph in paragraphs:
paragraph_line_counts.append(paragraph.count('\n') + 1)
print(f'Line count per paragraph: {paragraph_line_counts}')
六、使用第三方库
除了Python的内置函数,还可以使用第三方库如 pandas
和 nltk
来进行更复杂的统计操作。
- 使用
pandas
进行统计:
import pandas as pd
data = pd.read_csv('example.txt', delimiter='\n', header=None, names=['line'])
data['word_count'] = data['line'].apply(lambda x: len(x.split()))
data['char_count'] = data['line'].apply(lambda x: len(x))
total_word_count = data['word_count'].sum()
total_char_count = data['char_count'].sum()
print(f'Total number of words: {total_word_count}')
print(f'Total number of characters: {total_char_count}')
- 使用
nltk
进行统计:
import nltk
from nltk.tokenize import word_tokenize
nltk.download('punkt')
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
words = word_tokenize(content)
word_count = len(words)
print(f'Total number of words: {word_count}')
七、总结
对Python读取的txt文件进行统计是文本处理中的基本操作。本文介绍了如何使用Python内置函数来统计行数、单词数和字符数,以及一些高级统计操作。还介绍了使用第三方库如 pandas
和 nltk
进行更复杂的统计操作。通过学习这些方法,可以根据具体需求选择合适的统计方法,从而提高文本处理的效率和准确性。
相关问答FAQs:
如何用Python读取txt文件并进行数据统计?
要使用Python读取txt文件并进行统计,您可以使用内置的open()
函数来打开文件,并利用字符串方法和数据结构(如字典和列表)来存储和统计数据。例如,可以读取文件内容并统计每行的单词数量,或计算特定单词的出现次数。
可以使用哪些Python库来简化txt文件的统计过程?
除了Python内置的功能外,您还可以使用一些第三方库来简化文件读取和统计的过程。例如,pandas
库可以方便地处理文件数据,并进行复杂的统计分析。collections
模块中的Counter
类也非常适合用于统计单词频率或其他元素的出现次数。
如何处理大文件以提高性能和效率?
当处理大文件时,建议逐行读取文件而不是一次性加载整个文件内容。这可以通过使用with open()
语句来实现,以确保文件在读取后被正确关闭。此外,可以考虑使用生成器来处理数据,以减少内存消耗,从而提高性能和效率。
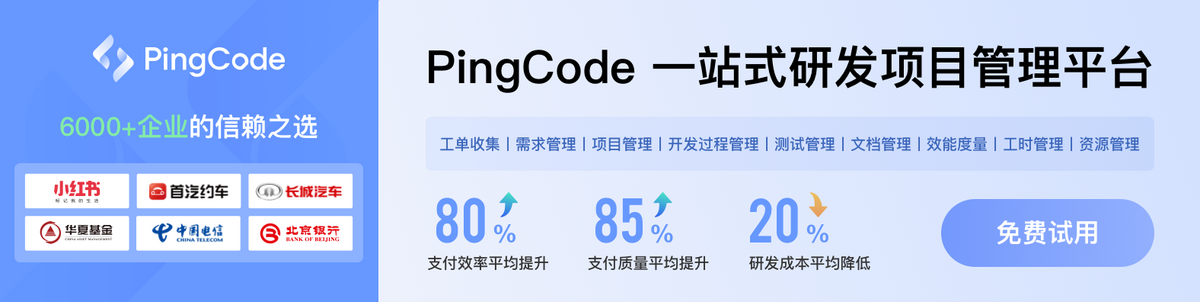