Python函数定义及注意事项
Python函数定义:
Python函数定义非常简单,可以通过使用关键字 def
来定义函数。函数定义使用 def 关键字、函数名要具有描述性、函数可以包含多个参数。例如,定义一个简单的函数如下:
def my_function(parameter1, parameter2):
# Function body
result = parameter1 + parameter2
return result
在这段代码中,my_function
是函数名称,parameter1
和 parameter2
是函数的参数,函数体包含要执行的代码,return
语句用于返回结果。
函数定义要点:
- 函数名要具有描述性:函数名应该清晰描述其用途。比如,一个计算加法的函数可以命名为
add
。 - 使用合适的参数:函数应该使用适当的参数来接收输入。参数可以有默认值,也可以是可选的。
- 函数文档字符串:函数定义的首行可以使用文档字符串(docstring)来描述函数的用途和用法。
- 函数返回值:确保函数返回适当的值,或者在不需要返回值时明确使用
return
。 - 错误处理:在函数中进行适当的错误处理,以提高函数的鲁棒性。
一、函数定义
1、定义简单函数
在 Python 中,函数的定义非常灵活,可以根据需要定义各种类型的函数。一个简单的函数可以如下定义:
def greet(name):
"""This function greets the person passed in as a parameter"""
print(f"Hello, {name}!")
这个函数接受一个参数 name
,并打印出问候语。函数的文档字符串用于描述该函数的用途。
2、定义带有默认参数的函数
有时候,我们希望某些参数有默认值,这样调用函数时可以不传递这些参数。例如:
def greet(name, greeting="Hello"):
"""This function greets the person with the given greeting"""
print(f"{greeting}, {name}!")
在这个函数中,greeting
参数有一个默认值 "Hello"
。调用时可以只传递 name
参数:
greet("Alice") # 输出: Hello, Alice!
greet("Bob", "Hi") # 输出: Hi, Bob!
3、定义带有可变参数的函数
有时候我们不确定传递给函数的参数个数,可以使用可变参数。可变参数包括 *args 和 kwargs。
def my_function(*args, kwargs):
"""This function demonstrates the use of *args and kwargs"""
print(f"Positional arguments: {args}")
print(f"Keyword arguments: {kwargs}")
调用该函数可以传递任意数量的参数:
my_function(1, 2, 3, name="Alice", age=30)
输出:
Positional arguments: (1, 2, 3)
Keyword arguments: {'name': 'Alice', 'age': 30}
二、函数返回值
1、返回单个值
函数可以返回一个值,使用 return
关键字:
def add(a, b):
"""This function returns the sum of two numbers"""
return a + b
调用该函数会返回两个参数的和:
result = add(2, 3) # result 为 5
2、返回多个值
Python 函数可以返回多个值,返回值可以是一个元组:
def divide_and_remainder(a, b):
"""This function returns the quotient and remainder of division"""
quotient = a // b
remainder = a % b
return quotient, remainder
调用该函数可以同时接收商和余数:
q, r = divide_and_remainder(10, 3)
q 为 3, r 为 1
三、函数文档字符串
文档字符串(docstring)用于描述函数的用途和用法,通常放在函数定义的首行。文档字符串可以通过 help()
函数查看:
def multiply(a, b):
"""This function returns the product of two numbers"""
return a * b
help(multiply)
输出:
Help on function multiply in module __main__:
multiply(a, b)
This function returns the product of two numbers
四、错误处理
1、使用 try-except 块
在函数中进行错误处理可以提高函数的鲁棒性,避免程序崩溃。例如:
def safe_divide(a, b):
"""This function divides two numbers and handles division by zero"""
try:
result = a / b
except ZeroDivisionError:
return "Cannot divide by zero"
return result
调用该函数时,如果 b
为 0,不会引发异常,而是返回一条错误信息:
print(safe_divide(10, 2)) # 输出: 5.0
print(safe_divide(10, 0)) # 输出: Cannot divide by zero
2、使用 assert 进行参数检查
在函数中使用 assert
语句可以对参数进行检查,确保参数满足预期条件:
def positive_sum(a, b):
"""This function returns the sum of two positive numbers"""
assert a > 0 and b > 0, "Both numbers must be positive"
return a + b
调用该函数时,如果参数不是正数,会引发 AssertionError:
print(positive_sum(5, 3)) # 输出: 8
print(positive_sum(-5, 3)) # 引发 AssertionError: Both numbers must be positive
五、函数的高级用法
1、匿名函数
Python 支持定义匿名函数,使用 lambda
关键字。匿名函数通常用于简单的操作,例如:
double = lambda x: x * 2
print(double(5)) # 输出: 10
匿名函数可以作为参数传递给其他函数,例如:
numbers = [1, 2, 3, 4, 5]
doubled_numbers = list(map(lambda x: x * 2, numbers))
print(doubled_numbers) # 输出: [2, 4, 6, 8, 10]
2、递归函数
递归函数是指在函数内部调用自身的函数。递归函数通常用于解决分治问题,例如计算阶乘:
def factorial(n):
"""This function returns the factorial of a number"""
if n == 1:
return 1
else:
return n * factorial(n - 1)
调用该函数可以计算阶乘:
print(factorial(5)) # 输出: 120
3、函数的闭包
闭包是指在函数内部定义的函数可以访问外部函数的变量。例如:
def outer_function(msg):
"""This function demonstrates closures"""
def inner_function():
print(msg)
return inner_function
closure = outer_function("Hello, World!")
closure() # 输出: Hello, World!
在这个例子中,inner_function
可以访问 outer_function
的变量 msg
,即使 outer_function
已经返回。
4、装饰器
装饰器是用于修改其他函数的功能的函数。使用装饰器可以在不修改原函数的情况下扩展其功能。例如:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
输出:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
在这个例子中,my_decorator
是一个装饰器,它在 say_hello
函数的前后打印消息。
5、生成器函数
生成器函数是使用 yield
关键字返回一个迭代器,逐个生成值而不是一次性返回所有值。例如:
def count_up_to(max):
"""This function generates numbers from 1 to max"""
count = 1
while count <= max:
yield count
count += 1
counter = count_up_to(5)
for num in counter:
print(num)
输出:
1
2
3
4
5
在这个例子中,count_up_to
是一个生成器函数,每次调用 yield
会暂停函数执行并返回一个值,下次调用时继续执行。
六、总结
Python 函数的定义和使用非常灵活,可以满足各种编程需求。定义函数时应注意函数名的描述性、参数的使用、文档字符串和错误处理。函数的高级用法包括匿名函数、递归函数、闭包、装饰器和生成器函数,这些特性使得 Python 函数更加强大和灵活。通过合理地使用这些特性,可以编写出高效、可读性强、易于维护的代码。
相关问答FAQs:
Python函数的定义格式是什么?
在Python中,函数的定义通常使用def
关键字,后接函数名称和括号中的参数列表。函数体需要缩进,示例如下:
def function_name(parameters):
# 函数体
return result
这种结构使得函数易于理解和使用,确保代码的可读性。
定义函数时应该考虑哪些参数类型?
在定义函数时,参数可以是位置参数、关键字参数、默认参数以及可变参数。位置参数是最常用的,而关键字参数则允许在调用时不按照顺序传递参数。默认参数提供了在未传入特定值时的默认行为。可变参数(如*args
和**kwargs
)允许函数接收不定数量的参数。这些设计使得函数更加灵活和通用。
在编写Python函数时需要注意哪些常见错误?
常见错误包括函数名重复、参数数量不匹配、缩进错误以及缺少return
语句。确保函数名具有描述性且不与内置函数冲突,可以提高代码的可维护性。参数数量不匹配通常发生在函数调用时,因此在调用函数之前,仔细检查参数个数和类型是很重要的。此外,保持一致的缩进风格和适当使用返回值可以避免逻辑错误。
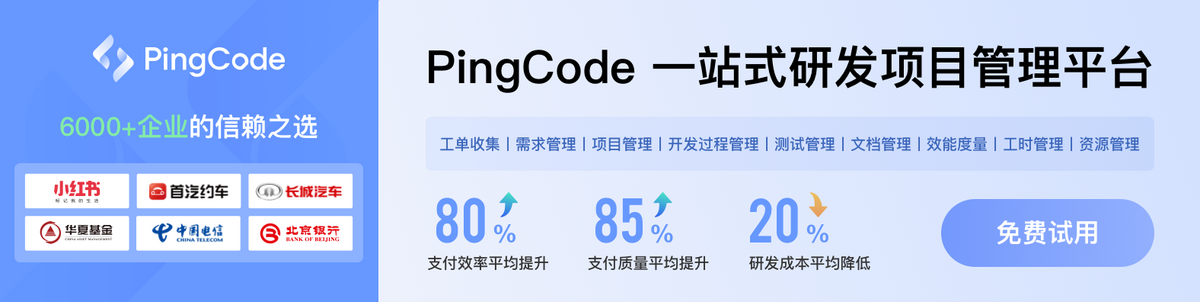