Python多线程间进行通信的几种方法有:使用线程安全的队列、使用事件对象、使用条件变量、使用全局变量或共享数据结构。 其中,使用线程安全的队列是最常用且推荐的方法。
使用线程安全的队列:Python的queue
模块提供了一个线程安全的队列,可以用于在线程间传递数据。队列可以确保数据的完整性,并且提供了简洁的接口来添加和获取数据。通过使用队列,生产者线程可以将数据放入队列,而消费者线程可以从队列中读取数据,从而实现了线程间的通信。
以下是关于如何在Python中使用这些方法来实现多线程通信的详细介绍。
一、使用线程安全的队列
Python的queue
模块提供了Queue
类,该类实现了一个线程安全的先进先出队列。通过使用Queue
,我们可以很容易地在多个线程之间传递数据。Queue
类提供了以下几个方法:
put(item)
: 将一个元素放入队列。get()
: 从队列中取出一个元素。task_done()
: 表示一个队列任务完成。join()
: 阻塞直到所有队列任务都被处理。
示例代码:
import threading
import queue
import time
def producer(queue, n):
for i in range(n):
item = f"item-{i}"
queue.put(item)
print(f"Produced {item}")
time.sleep(1)
def consumer(queue):
while True:
item = queue.get()
if item is None:
break
print(f"Consumed {item}")
queue.task_done()
q = queue.Queue()
n = 10
t1 = threading.Thread(target=producer, args=(q, n))
t2 = threading.Thread(target=consumer, args=(q,))
t1.start()
t2.start()
t1.join()
q.put(None) # Sentinel to stop the consumer
t2.join()
在以上代码中,producer
线程将生产的项目放入队列,而consumer
线程从队列中获取项目并处理它们。生产者线程生产10个项目,并将一个None
作为哨兵值放入队列,以通知消费者线程停止。
二、使用事件对象
threading.Event
对象可以用于实现线程间的信号通信。事件对象管理一个内部标志,线程可以等待该标志被设置,或者设置/清除该标志以通知其他线程。
示例代码:
import threading
import time
def worker(event):
print("Worker waiting for event...")
event.wait()
print("Event received! Worker proceeding...")
event = threading.Event()
t = threading.Thread(target=worker, args=(event,))
t.start()
time.sleep(3)
print("Main thread setting event.")
event.set()
t.join()
在这个例子中,worker
线程等待事件对象被设置,而主线程在3秒后设置事件对象,从而通知worker
线程继续执行。
三、使用条件变量
条件变量通过threading.Condition
类来实现,允许线程等待某个条件变为真。条件变量通常与锁结合使用,以确保线程安全。
示例代码:
import threading
import time
class SharedResource:
def __init__(self):
self.condition = threading.Condition()
self.data_ready = False
def produce(self):
with self.condition:
print("Producing data...")
time.sleep(2)
self.data_ready = True
self.condition.notify_all()
print("Data produced and notification sent.")
def consume(self):
with self.condition:
while not self.data_ready:
print("Waiting for data...")
self.condition.wait()
print("Data consumed.")
shared_resource = SharedResource()
producer_thread = threading.Thread(target=shared_resource.produce)
consumer_thread = threading.Thread(target=shared_resource.consume)
consumer_thread.start()
producer_thread.start()
producer_thread.join()
consumer_thread.join()
在这个示例中,SharedResource
类使用条件变量来协调生产者和消费者线程。消费者线程等待数据准备好,而生产者线程在数据准备好后通知所有等待的线程。
四、使用全局变量或共享数据结构
虽然不推荐,但在某些情况下可以使用全局变量或共享数据结构来实现线程间通信。需要注意的是,这种方法需要手动处理线程同步,以避免数据竞争和不一致性。
示例代码:
import threading
import time
shared_data = None
lock = threading.Lock()
def producer():
global shared_data
with lock:
print("Producing data...")
time.sleep(2)
shared_data = "Data"
print("Data produced.")
def consumer():
global shared_data
while True:
with lock:
if shared_data is not None:
print(f"Consumed {shared_data}")
shared_data = None
break
time.sleep(1)
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
producer_thread.join()
consumer_thread.join()
在这个示例中,使用全局变量shared_data
来共享数据,并使用锁来确保线程同步。生产者线程生产数据并更新全局变量,而消费者线程等待数据可用后进行消费。
五、总结
在Python中,有多种方法可以实现多线程间的通信,每种方法都有其适用场景和优缺点。使用线程安全的队列是最常用且推荐的方法,因为它提供了简单且安全的接口来传递数据。使用事件对象和条件变量可以实现更复杂的同步和通知机制,而使用全局变量或共享数据结构虽然不推荐,但在某些特定情况下也可以使用。根据具体需求选择合适的方法,可以有效地实现多线程间的通信和协作。
相关问答FAQs:
在Python中,如何实现多线程之间的安全通信?
在Python中,可以使用queue.Queue
类来实现线程之间的安全通信。这个类提供了线程安全的队列,允许一个线程将数据放入队列中,而另一个线程可以从队列中取出数据。使用队列可以避免线程间的竞争条件,从而保证数据的一致性。
Python多线程通信中有哪些常用的同步机制?
在Python中,常用的同步机制包括threading.Lock
、threading.Event
、threading.Condition
和threading.Semaphore
。这些机制可以帮助管理线程对共享资源的访问,确保数据的完整性和一致性。使用这些工具,开发者可以更好地控制线程的执行顺序和状态。
如何在Python多线程中处理异常?
在多线程环境中,处理异常需要特别小心。可以在每个线程中使用try-except
块来捕获异常,并在捕获到异常时进行适当的处理,比如记录日志或发送通知。此外,使用threading.Thread
类的setDaemon()
方法,可以确保主线程在所有子线程完成之前不会退出,从而避免未处理的异常导致程序提前终止。
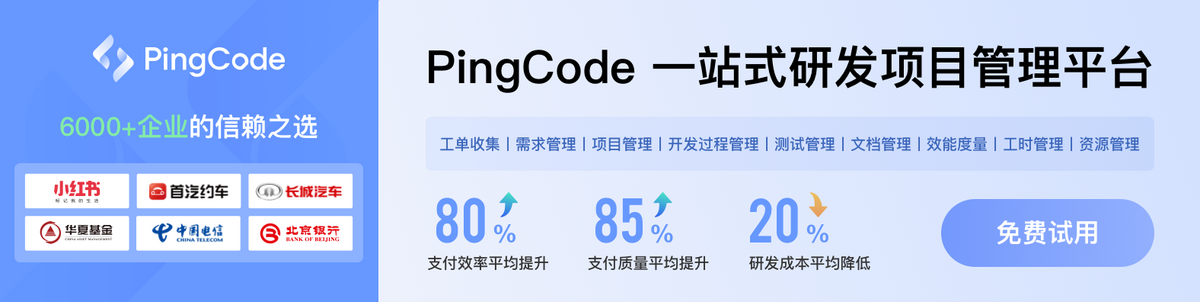