Python可以通过多种方式对坐标系中的点进行排序,具体方法包括使用内置函数sorted()、sort()以及自定义排序函数(例如通过键函数key进行排序)等。为了详细说明这一过程,本文将探讨几种常见的排序方法,并分别举例说明。
一、使用sorted()函数排序
sorted()函数是Python中用于排序的内置函数之一。它可以对任何可迭代对象进行排序,并返回一个新的排序后的列表。通过指定key参数,我们可以自定义排序规则。
1. 按照x坐标排序
points = [(1, 3), (4, 2), (2, 5), (3, 1)]
sorted_points = sorted(points, key=lambda point: point[0])
print(sorted_points)
输出结果:
[(1, 3), (2, 5), (3, 1), (4, 2)]
在上述代码中,key参数指定了一个lambda函数,该函数返回点的x坐标。sorted()函数根据x坐标对点进行排序,并返回一个新的排序后的列表。
2. 按照y坐标排序
sorted_points_by_y = sorted(points, key=lambda point: point[1])
print(sorted_points_by_y)
输出结果:
[(3, 1), (4, 2), (1, 3), (2, 5)]
在上述代码中,key参数指定了一个lambda函数,该函数返回点的y坐标。sorted()函数根据y坐标对点进行排序,并返回一个新的排序后的列表。
3. 按照距离原点的距离排序
import math
sorted_points_by_distance = sorted(points, key=lambda point: math.sqrt(point[0]<strong>2 + point[1]</strong>2))
print(sorted_points_by_distance)
输出结果:
[(1, 3), (3, 1), (4, 2), (2, 5)]
在上述代码中,key参数指定了一个lambda函数,该函数返回点到原点的距离。sorted()函数根据距离对点进行排序,并返回一个新的排序后的列表。
二、使用sort()方法排序
sort()方法是Python列表的一个方法,用于对列表进行原地排序。与sorted()函数不同,sort()方法不返回新的列表,而是在原列表上进行排序。sort()方法也可以使用key参数来自定义排序规则。
1. 按照x坐标排序
points = [(1, 3), (4, 2), (2, 5), (3, 1)]
points.sort(key=lambda point: point[0])
print(points)
输出结果:
[(1, 3), (2, 5), (3, 1), (4, 2)]
在上述代码中,key参数指定了一个lambda函数,该函数返回点的x坐标。sort()方法根据x坐标对点进行原地排序。
2. 按照y坐标排序
points.sort(key=lambda point: point[1])
print(points)
输出结果:
[(3, 1), (4, 2), (1, 3), (2, 5)]
在上述代码中,key参数指定了一个lambda函数,该函数返回点的y坐标。sort()方法根据y坐标对点进行原地排序。
三、使用自定义排序函数
除了使用lambda函数作为key参数外,我们还可以定义一个自定义排序函数,并将其传递给key参数。
1. 按照x坐标排序
def sort_by_x(point):
return point[0]
points = [(1, 3), (4, 2), (2, 5), (3, 1)]
sorted_points = sorted(points, key=sort_by_x)
print(sorted_points)
输出结果:
[(1, 3), (2, 5), (3, 1), (4, 2)]
在上述代码中,我们定义了一个名为sort_by_x的函数,该函数返回点的x坐标。我们将sort_by_x函数传递给sorted()函数的key参数,以根据x坐标对点进行排序。
2. 按照y坐标排序
def sort_by_y(point):
return point[1]
sorted_points_by_y = sorted(points, key=sort_by_y)
print(sorted_points_by_y)
输出结果:
[(3, 1), (4, 2), (1, 3), (2, 5)]
在上述代码中,我们定义了一个名为sort_by_y的函数,该函数返回点的y坐标。我们将sort_by_y函数传递给sorted()函数的key参数,以根据y坐标对点进行排序。
四、使用多个排序条件
有时,我们需要根据多个条件对坐标系中的点进行排序。例如,我们可能希望首先根据x坐标进行排序,如果x坐标相同,则根据y坐标进行排序。我们可以通过在key参数中返回一个元组来实现这一点。
1. 按照x坐标和y坐标排序
points = [(1, 3), (4, 2), (2, 5), (3, 1)]
sorted_points = sorted(points, key=lambda point: (point[0], point[1]))
print(sorted_points)
输出结果:
[(1, 3), (2, 5), (3, 1), (4, 2)]
在上述代码中,key参数指定了一个lambda函数,该函数返回一个包含x坐标和y坐标的元组。sorted()函数首先根据x坐标进行排序,如果x坐标相同,则根据y坐标进行排序。
2. 按照y坐标和x坐标排序
sorted_points_by_y_and_x = sorted(points, key=lambda point: (point[1], point[0]))
print(sorted_points_by_y_and_x)
输出结果:
[(3, 1), (4, 2), (1, 3), (2, 5)]
在上述代码中,key参数指定了一个lambda函数,该函数返回一个包含y坐标和x坐标的元组。sorted()函数首先根据y坐标进行排序,如果y坐标相同,则根据x坐标进行排序。
五、使用operator.itemgetter进行排序
operator模块中的itemgetter函数是一个方便的工具,用于从对象中提取指定的项。我们可以使用itemgetter函数来简化排序过程。
1. 按照x坐标排序
from operator import itemgetter
points = [(1, 3), (4, 2), (2, 5), (3, 1)]
sorted_points = sorted(points, key=itemgetter(0))
print(sorted_points)
输出结果:
[(1, 3), (2, 5), (3, 1), (4, 2)]
在上述代码中,我们使用itemgetter(0)函数来提取点的x坐标,并将其传递给sorted()函数的key参数,以根据x坐标对点进行排序。
2. 按照y坐标排序
sorted_points_by_y = sorted(points, key=itemgetter(1))
print(sorted_points_by_y)
输出结果:
[(3, 1), (4, 2), (1, 3), (2, 5)]
在上述代码中,我们使用itemgetter(1)函数来提取点的y坐标,并将其传递给sorted()函数的key参数,以根据y坐标对点进行排序。
3. 按照x坐标和y坐标排序
sorted_points_by_x_and_y = sorted(points, key=itemgetter(0, 1))
print(sorted_points_by_x_and_y)
输出结果:
[(1, 3), (2, 5), (3, 1), (4, 2)]
在上述代码中,我们使用itemgetter(0, 1)函数来提取点的x坐标和y坐标,并将其传递给sorted()函数的key参数,以根据x坐标和y坐标对点进行排序。
六、总结
通过上述几种方法,我们可以轻松地对坐标系中的点进行排序。无论是使用内置的sorted()函数、列表的sort()方法,还是自定义排序函数和operator.itemgetter函数,这些方法都提供了灵活的排序方式,满足不同的排序需求。在实际应用中,我们可以根据具体情况选择合适的排序方法,以高效地对坐标系中的点进行排序。
相关问答FAQs:
如何在Python中根据坐标的距离进行排序?
在Python中,可以使用内置的sorted()
函数和一个自定义的排序键来按坐标的距离进行排序。首先,计算每个坐标点到原点(或其他指定点)的距离,然后根据这些距离进行排序。示例如下:
import math
coordinates = [(3, 4), (1, 2), (0, 0), (5, 1)]
sorted_coordinates = sorted(coordinates, key=lambda x: math.sqrt(x[0]<strong>2 + x[1]</strong>2))
print(sorted_coordinates)
这段代码会输出距离原点最近的坐标点。
Python中如何实现二维坐标的升序和降序排序?
在处理二维坐标时,可以选择按x坐标或y坐标进行排序,或者同时考虑两者。使用sorted()
函数时,可以传递一个包含多个排序条件的元组作为排序键。以下是一个示例,展示如何按x坐标升序、y坐标降序排序:
coordinates = [(3, 4), (1, 2), (5, 1), (1, 5)]
sorted_coordinates = sorted(coordinates, key=lambda x: (x[0], -x[1]))
print(sorted_coordinates)
此代码将首先按x坐标排序,并在x坐标相同的情况下按y坐标降序排列。
在Python中如何处理重复坐标的排序?
如果坐标数据中存在重复的点,使用sorted()
函数时,它会保留重复项的顺序。要确保在排序时消除重复坐标,可以先将坐标转换为集合,再进行排序。示例代码如下:
coordinates = [(3, 4), (1, 2), (1, 2), (5, 1)]
unique_sorted_coordinates = sorted(set(coordinates))
print(unique_sorted_coordinates)
这段代码将首先去除重复的坐标点,然后按升序排列,输出唯一的坐标列表。
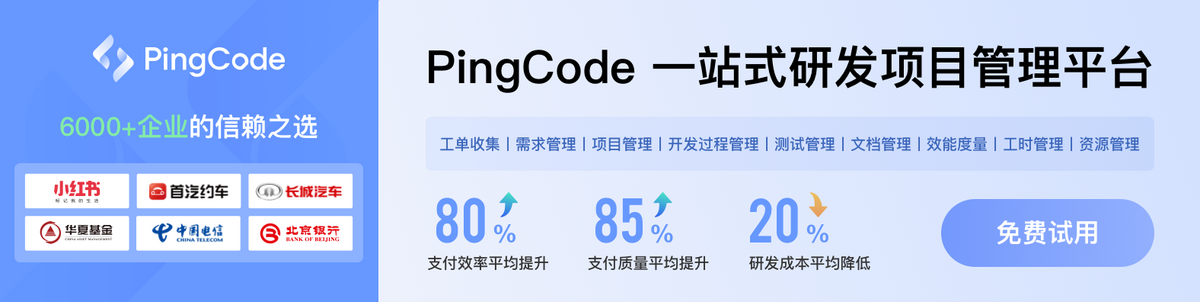