Python将图片存入数据库的方法有多种,包括使用二进制格式存储、Base64编码等。 常见的方法有将图片转换为二进制数据(BLOB)并存储在数据库中,或者将图片转换为Base64编码后存储在数据库中。在详细描述之前,首先需要了解数据库的选择和Python的相关库。
一、选择数据库
数据库的选择对于如何存储和读取图片至关重要。常见的数据库包括MySQL、PostgreSQL、SQLite等。以下将以MySQL为例详细介绍如何将图片存入数据库。
二、准备工作
在进行图片存储操作之前,需要确保以下工具已经安装和配置好:
- MySQL数据库
- Python编程环境
pymysql
库(用于Python与MySQL数据库的连接)
三、将图片转换为二进制数据并存储
1、连接数据库
首先,需要连接到数据库,下面是使用pymysql
库连接到MySQL数据库的示例代码:
import pymysql
连接到数据库
connection = pymysql.connect(
host='localhost',
user='yourusername',
password='yourpassword',
database='yourdatabase'
)
cursor = connection.cursor()
2、创建存储图片的表
在数据库中创建一个表,用于存储图片数据:
CREATE TABLE images (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
data LONGBLOB NOT NULL
);
3、将图片读取为二进制数据
使用Python读取图片文件并将其转换为二进制数据:
def read_image(file_path):
with open(file_path, 'rb') as file:
binary_data = file.read()
return binary_data
4、将二进制数据存储到数据库
编写代码将二进制数据存储到数据库中:
def store_image(name, file_path):
binary_data = read_image(file_path)
cursor.execute("INSERT INTO images (name, data) VALUES (%s, %s)", (name, binary_data))
connection.commit()
四、从数据库中读取图片
1、从数据库中读取二进制数据
编写代码从数据库中读取二进制数据:
def read_image_from_db(image_id):
cursor.execute("SELECT data FROM images WHERE id = %s", (image_id,))
result = cursor.fetchone()
return result[0] if result else None
2、将二进制数据写入文件
将读取到的二进制数据写入图片文件:
def write_image(file_path, binary_data):
with open(file_path, 'wb') as file:
file.write(binary_data)
五、完整示例代码
将上述步骤整合到一个完整的示例代码中:
import pymysql
def connect_to_db():
return pymysql.connect(
host='localhost',
user='yourusername',
password='yourpassword',
database='yourdatabase'
)
def create_table(cursor):
cursor.execute("""
CREATE TABLE IF NOT EXISTS images (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
data LONGBLOB NOT NULL
);
""")
def read_image(file_path):
with open(file_path, 'rb') as file:
binary_data = file.read()
return binary_data
def store_image(cursor, name, file_path):
binary_data = read_image(file_path)
cursor.execute("INSERT INTO images (name, data) VALUES (%s, %s)", (name, binary_data))
def read_image_from_db(cursor, image_id):
cursor.execute("SELECT data FROM images WHERE id = %s", (image_id,))
result = cursor.fetchone()
return result[0] if result else None
def write_image(file_path, binary_data):
with open(file_path, 'wb') as file:
file.write(binary_data)
def mAIn():
connection = connect_to_db()
cursor = connection.cursor()
create_table(cursor)
# Store image
store_image(cursor, 'example_image', 'path_to_your_image.jpg')
connection.commit()
# Read image from database and save to file
binary_data = read_image_from_db(cursor, 1)
if binary_data:
write_image('output_image.jpg', binary_data)
cursor.close()
connection.close()
if __name__ == '__main__':
main()
六、其他数据库的处理
1、PostgreSQL
对于PostgreSQL数据库,可以使用psycopg2
库进行连接和操作。代码逻辑与MySQL类似,只需要更改连接部分和库的导入:
import psycopg2
def connect_to_db():
return psycopg2.connect(
host='localhost',
user='yourusername',
password='yourpassword',
dbname='yourdatabase'
)
其余部分代码逻辑基本相同,只需适当调整。
2、SQLite
对于SQLite数据库,可以使用sqlite3
库进行连接和操作。代码逻辑与MySQL类似,只需要更改连接部分和库的导入:
import sqlite3
def connect_to_db():
return sqlite3.connect('yourdatabase.db')
其余部分代码逻辑基本相同,只需适当调整。
七、注意事项
- 数据量与性能:存储大量图片数据会导致数据库文件变得非常大,影响性能。可以考虑将图片存储在文件系统中,仅将图片路径存储在数据库中。
- 安全性:确保数据库连接信息的安全性,避免将敏感信息暴露在代码中。
- 备份与恢复:定期备份数据库,确保数据安全。
总结
本文详细介绍了如何使用Python将图片存入数据库的方法,包括选择数据库、准备工作、将图片转换为二进制数据并存储、从数据库中读取图片、完整示例代码、其他数据库的处理以及注意事项。通过这些步骤,您可以轻松实现将图片存入数据库的功能。
相关问答FAQs:
如何使用Python将图片存储到数据库中?
在Python中,可以使用多种库将图片存储到数据库。常用的库包括PIL
(Pillow)用于处理图片,sqlite3
或SQLAlchemy
用于与数据库交互。首先,您需要将图片读取为二进制数据,然后通过SQL语句将其插入到数据库中。确保数据库表中有适合存储二进制数据的字段类型,如BLOB。
存储图片到数据库时需要注意哪些事项?
在将图片存入数据库时,有几个关键因素需要考虑。首先,数据库的容量和性能可能会受到影响,因此需要评估是否存储图片是最佳选择。其次,确保使用适当的图片格式,以避免数据损坏。此外,合理地设计数据库表结构也很重要,比如为图片添加描述字段,以便后续检索和管理。
如何从数据库中提取并显示图片?
提取存储在数据库中的图片可以通过Python的数据库连接库实现。首先,使用SQL查询获取图片的二进制数据。接下来,可以使用PIL
(Pillow)库将二进制数据转换为图片格式,并在应用程序中进行显示。确保在提取和显示图片时,处理好异常情况,比如连接失败或数据损坏。
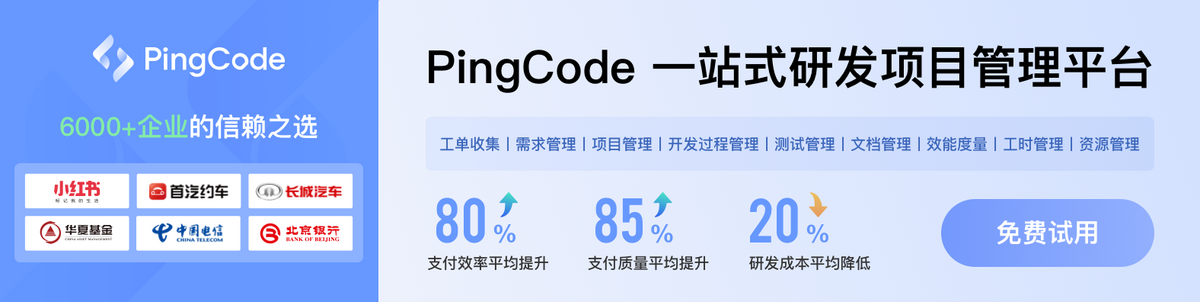