Python同时调用两个函数的常用方法有多线程、多进程、异步编程等。使用多线程能够让两个函数并行执行,从而提高程序运行效率。下面将详细介绍如何使用多线程来同时调用两个函数。
一、导入所需模块
在Python中,要使用多线程功能,需要导入threading
模块。多线程允许你在一个程序中并发地运行多个操作。
import threading
二、定义要并行执行的函数
首先,我们需要定义两个函数,这两个函数将在多线程中并行运行。这里我们使用简单的示例函数来演示。
def function1():
for i in range(5):
print("Function 1 - Iteration", i)
def function2():
for i in range(5):
print("Function 2 - Iteration", i)
三、创建并启动线程
接下来,我们需要创建线程对象,并将函数作为参数传递给这些线程对象。然后,使用start()
方法启动线程。
# 创建线程
thread1 = threading.Thread(target=function1)
thread2 = threading.Thread(target=function2)
启动线程
thread1.start()
thread2.start()
等待所有线程完成
thread1.join()
thread2.join()
四、完整示例
结合上面的步骤,我们可以创建一个完整的示例程序:
import threading
定义第一个函数
def function1():
for i in range(5):
print("Function 1 - Iteration", i)
定义第二个函数
def function2():
for i in range(5):
print("Function 2 - Iteration", i)
创建线程
thread1 = threading.Thread(target=function1)
thread2 = threading.Thread(target=function2)
启动线程
thread1.start()
thread2.start()
等待所有线程完成
thread1.join()
thread2.join()
print("Both functions have completed execution.")
五、多线程的注意事项
-
线程安全:多线程程序需要注意线程安全问题。如果多个线程同时访问共享资源,如全局变量或文件,可能会导致数据竞争和不一致的问题。使用线程锁(
threading.Lock
)可以帮助解决这些问题。 -
性能:虽然多线程可以提高程序的并发性,但创建和管理线程也会带来一定的开销。因此,在某些情况下,线程数量过多可能会导致性能下降。
-
GIL:Python的全局解释器锁(GIL)可能会影响多线程程序的性能,尤其是在CPU密集型任务中。对于这些情况,可以考虑使用多进程(
multiprocessing
模块)来代替多线程。
六、多进程示例
如果你需要更高的并发性或处理CPU密集型任务,可以使用multiprocessing
模块来创建多进程程序。多进程允许在多个CPU核心上并行运行,避免了GIL的限制。
import multiprocessing
定义第一个函数
def function1():
for i in range(5):
print("Function 1 - Iteration", i)
定义第二个函数
def function2():
for i in range(5):
print("Function 2 - Iteration", i)
创建进程
process1 = multiprocessing.Process(target=function1)
process2 = multiprocessing.Process(target=function2)
启动进程
process1.start()
process2.start()
等待所有进程完成
process1.join()
process2.join()
print("Both functions have completed execution.")
七、异步编程示例
异步编程是另一种并发执行方法,特别适用于I/O密集型任务。可以使用asyncio
模块来实现异步函数的并发执行。
import asyncio
定义第一个异步函数
async def function1():
for i in range(5):
print("Function 1 - Iteration", i)
await asyncio.sleep(1) # 模拟异步操作
定义第二个异步函数
async def function2():
for i in range(5):
print("Function 2 - Iteration", i)
await asyncio.sleep(1) # 模拟异步操作
主函数
async def main():
# 创建任务
task1 = asyncio.create_task(function1())
task2 = asyncio.create_task(function2())
# 等待所有任务完成
await task1
await task2
运行异步程序
asyncio.run(main())
结论
在Python中同时调用两个函数的方法有多种,包括多线程、多进程和异步编程。选择哪种方法取决于具体的应用场景和需求。多线程适用于I/O密集型任务,多进程适用于CPU密集型任务,异步编程适用于需要处理大量并发I/O操作的任务。通过合理选择和使用这些并发编程技术,可以显著提高程序的性能和效率。
相关问答FAQs:
如何在Python中实现多线程来同时调用两个函数?
在Python中,可以使用threading
模块来实现多线程,从而同时调用两个函数。首先,导入threading
模块,然后创建两个线程,每个线程对应一个函数。使用start()
方法启动线程,最后使用join()
方法确保主程序等待线程执行完毕。代码示例如下:
import threading
def function_one():
print("Function One is running")
def function_two():
print("Function Two is running")
# 创建线程
thread1 = threading.Thread(target=function_one)
thread2 = threading.Thread(target=function_two)
# 启动线程
thread1.start()
thread2.start()
# 等待线程结束
thread1.join()
thread2.join()
在Python中使用异步编程来调用两个函数的好处是什么?
使用异步编程(asyncio
模块)可以更高效地管理I/O密集型操作,如网络请求或文件读写。通过async
和await
关键字,可以同时调用多个函数,而不会阻塞其他操作。这种方式特别适合需要同时处理多个任务的场景,能够提高程序的响应速度和性能。
是否可以使用进程来同时调用两个函数?
当然可以。Python的multiprocessing
模块允许你创建多个进程,每个进程可以运行不同的函数,这样就能充分利用多核CPU的能力。与线程相比,进程之间的数据共享较少,因此在处理CPU密集型任务时,使用进程通常会更高效。代码示例:
from multiprocessing import Process
def function_one():
print("Function One is running in a separate process")
def function_two():
print("Function Two is running in a separate process")
if __name__ == "__main__":
process1 = Process(target=function_one)
process2 = Process(target=function_two)
process1.start()
process2.start()
process1.join()
process2.join()
通过以上方法,您可以根据具体需求选择最适合的方式来同时调用多个函数。
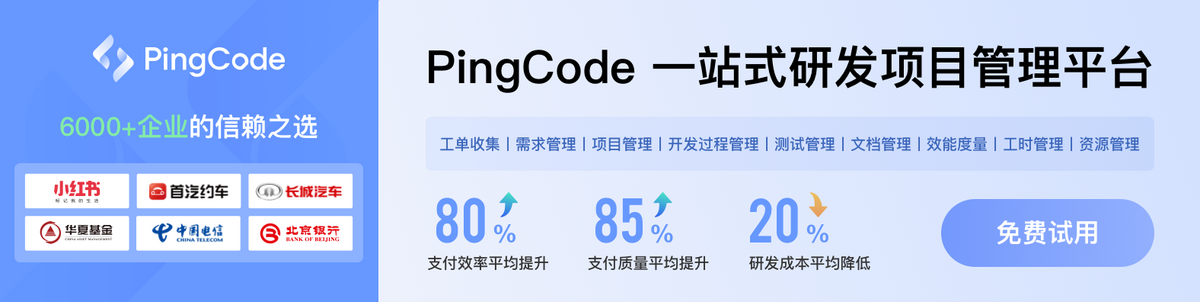