在Python中,自定义类的使用是编程中的一个重要部分。创建类、定义类属性和方法、实例化类对象、调用类方法等都是使用自定义类的关键步骤。下面将对其中的“创建类”展开详细描述。
创建类是定义类的最基础步骤,它为类提供了一个模板。我们通过class
关键字来创建一个类,并在类中定义属性和方法。例如:
class MyClass:
def __init__(self, attribute1, attribute2):
self.attribute1 = attribute1
self.attribute2 = attribute2
def method1(self):
return f'Attribute1 is {self.attribute1}'
def method2(self):
return f'Attribute2 is {self.attribute2}'
在这个例子中,MyClass
是一个自定义类,它有两个属性attribute1
和attribute2
,以及两个方法method1
和method2
。
一、创建类
在Python中,创建类是使用自定义类的第一步。创建类时需要使用class
关键字,并且通常会定义一个特殊的初始化方法__init__
来初始化对象的属性。
class MyClass:
def __init__(self, attribute1, attribute2):
self.attribute1 = attribute1
self.attribute2 = attribute2
在上面的例子中,MyClass
是类名,__init__
方法是一个特殊的初始化方法,self
参数是对实例本身的引用。通过self
可以访问类的属性和方法。
二、定义类属性和方法
类属性是类的变量,它们存储了类的状态。类方法是类的函数,它们定义了类的行为。类属性和方法可以在类定义中设置。
class MyClass:
def __init__(self, attribute1, attribute2):
self.attribute1 = attribute1
self.attribute2 = attribute2
def method1(self):
return f'Attribute1 is {self.attribute1}'
def method2(self):
return f'Attribute2 is {self.attribute2}'
在上面的例子中,attribute1
和attribute2
是类的属性,method1
和method2
是类的方法。方法可以访问和修改类的属性。
三、实例化类对象
实例化类对象是使用类的一个重要步骤。通过实例化,可以创建类的具体对象,并且可以访问和修改对象的属性和方法。
my_object = MyClass('value1', 'value2')
print(my_object.attribute1) # Output: value1
print(my_object.method1()) # Output: Attribute1 is value1
在上面的例子中,my_object
是MyClass
类的一个实例,通过实例可以访问类的属性和方法。
四、调用类方法
调用类方法是使用类的关键步骤之一。通过调用类方法,可以执行类的行为,并且可以访问和修改类的属性。
my_object = MyClass('value1', 'value2')
print(my_object.method1()) # Output: Attribute1 is value1
在上面的例子中,通过调用method1
方法,可以访问attribute1
的值,并且可以执行类的方法。
五、继承和多态
继承是面向对象编程中的一个重要概念,通过继承,可以创建一个新的类,该类具有现有类的属性和方法。多态是指在不同的类中使用相同的方法名称,但具有不同的行为。
class MyBaseClass:
def method(self):
return 'Base method'
class MyDerivedClass(MyBaseClass):
def method(self):
return 'Derived method'
base_object = MyBaseClass()
derived_object = MyDerivedClass()
print(base_object.method()) # Output: Base method
print(derived_object.method()) # Output: Derived method
在上面的例子中,MyDerivedClass
继承了MyBaseClass
,并且重写了method
方法。这是多态的一种体现。
六、类的封装
封装是面向对象编程中的一个重要概念,通过封装,可以隐藏类的内部实现细节,只暴露类的公共接口。
class MyClass:
def __init__(self, attribute1, attribute2):
self.__attribute1 = attribute1
self.__attribute2 = attribute2
def get_attribute1(self):
return self.__attribute1
def set_attribute1(self, value):
self.__attribute1 = value
在上面的例子中,__attribute1
和__attribute2
是私有属性,通过get_attribute1
和set_attribute1
方法,可以访问和修改私有属性。
七、类的多继承
多继承是指一个类可以继承多个父类的属性和方法。在Python中,可以通过在类定义中列出多个父类来实现多继承。
class BaseClass1:
def method1(self):
return 'Method from BaseClass1'
class BaseClass2:
def method2(self):
return 'Method from BaseClass2'
class DerivedClass(BaseClass1, BaseClass2):
pass
derived_object = DerivedClass()
print(derived_object.method1()) # Output: Method from BaseClass1
print(derived_object.method2()) # Output: Method from BaseClass2
在上面的例子中,DerivedClass
继承了BaseClass1
和BaseClass2
,因此可以访问这两个类的属性和方法。
八、类的组合
组合是指在一个类中使用另一个类的实例作为属性。通过组合,可以构建复杂的类结构。
class Engine:
def start(self):
return 'Engine started'
class Car:
def __init__(self):
self.engine = Engine()
def start(self):
return self.engine.start()
car = Car()
print(car.start()) # Output: Engine started
在上面的例子中,Car
类包含一个Engine
类的实例,通过组合,可以使用Engine
类的属性和方法。
九、类的静态方法和类方法
静态方法和类方法是类的特殊方法,它们可以在不实例化类的情况下调用。静态方法使用@staticmethod
装饰器,类方法使用@classmethod
装饰器。
class MyClass:
@staticmethod
def static_method():
return 'Static method called'
@classmethod
def class_method(cls):
return 'Class method called'
print(MyClass.static_method()) # Output: Static method called
print(MyClass.class_method()) # Output: Class method called
在上面的例子中,通过@staticmethod
和@classmethod
装饰器,可以定义静态方法和类方法,并且可以在不实例化类的情况下调用这些方法。
十、类的特殊方法
特殊方法是类的特殊函数,它们以双下划线开头和结尾,例如__init__
、__str__
、__repr__
等。特殊方法可以定义类的特殊行为。
class MyClass:
def __init__(self, attribute):
self.attribute = attribute
def __str__(self):
return f'MyClass with attribute: {self.attribute}'
def __repr__(self):
return f'MyClass(attribute={self.attribute})'
my_object = MyClass('value')
print(str(my_object)) # Output: MyClass with attribute: value
print(repr(my_object)) # Output: MyClass(attribute=value)
在上面的例子中,__str__
和__repr__
是特殊方法,它们定义了类的字符串表示形式。
通过以上内容,我们详细介绍了Python中自定义类的使用方法,包括创建类、定义类属性和方法、实例化类对象、调用类方法、继承和多态、封装、多继承、组合、静态方法和类方法、特殊方法等。这些内容涵盖了自定义类的主要使用方法和技巧,希望对您在Python编程中使用自定义类有所帮助。
相关问答FAQs:
如何在Python中创建自定义类?
在Python中,创建自定义类非常简单。您可以使用class
关键字来定义一个类。类的基本结构包括类名、属性和方法。例如:
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
return f"Hello, {self.name}!"
这个示例展示了如何定义一个简单的类MyClass
,它有一个构造方法__init__
和一个实例方法greet
。
自定义类的实例如何访问属性和方法?
一旦定义了自定义类,您可以创建它的实例并通过点符号访问其属性和方法。例如:
obj = MyClass("Alice")
print(obj.greet()) # 输出: Hello, Alice!
通过obj.greet()
调用方法,您可以获取相应的返回值,同时obj.name
可以访问到name
属性。
如何在自定义类中实现继承?
在Python中,您可以通过继承来创建一个新类,使其继承自现有的类。这可以通过在类定义中指定父类来实现。例如:
class Animal:
def speak(self):
return "Animal speaks"
class Dog(Animal):
def bark(self):
return "Woof!"
在这个例子中,Dog
类继承了Animal
类的方法 speak
,同时定义了自己的bark
方法。您可以通过创建Dog
类的实例来调用这些方法。
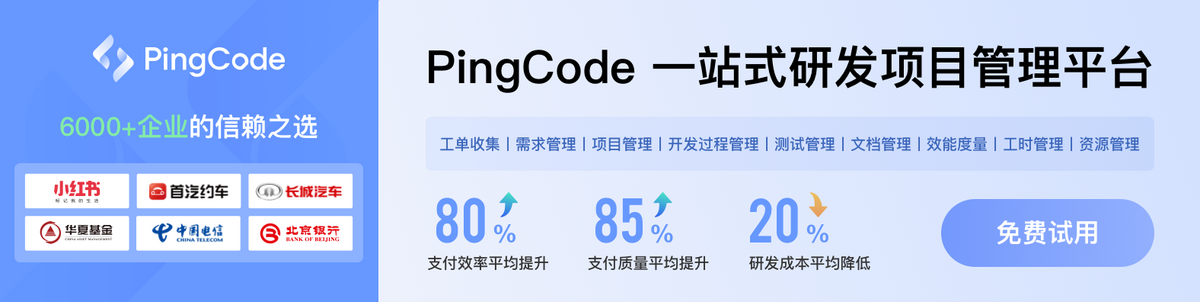