Python字符串匹配的方法有多种,包括使用find()方法、正则表达式、in关键字、startswith()方法、endswith()方法。这些方法各有优缺点,适用于不同的场景。下面将详细介绍其中一种方法,并进一步展开介绍其他方法。
正则表达式 是一种强大的工具,用于在字符串中搜索特定模式。Python的re
模块提供了对正则表达式的支持。使用正则表达式可以进行复杂的字符串匹配,包括匹配单个字符、多字符、分组匹配等。以下是一个使用正则表达式匹配字符串的例子:
import re
pattern = r'\bhello\b'
text = 'hello world, hello Python'
matches = re.findall(pattern, text)
print(matches)
在这个例子中,\b
表示单词边界,hello
是我们要匹配的字符串,re.findall()
函数返回一个列表,包含所有匹配的子串。
一、使用find()方法
find()方法用于在字符串中查找子字符串。如果找到子字符串,返回子字符串的起始索引;否则,返回-1。
text = "hello world"
index = text.find("world")
if index != -1:
print(f"Found 'world' at index {index}")
else:
print("Did not find 'world'")
在这个例子中,find()
方法找到了"world"子字符串,并返回了它的起始索引。
二、使用in关键字
in关键字可以用于检查一个字符串是否包含另一个字符串。它返回一个布尔值。
text = "hello world"
if "world" in text:
print("Found 'world'")
else:
print("Did not find 'world'")
使用in关键字非常直观和易于理解,适用于简单的字符串匹配。
三、使用startswith()和endswith()方法
startswith()方法用于检查字符串是否以特定子字符串开头,endswith()方法用于检查字符串是否以特定子字符串结尾。
text = "hello world"
if text.startswith("hello"):
print("String starts with 'hello'")
if text.endswith("world"):
print("String ends with 'world'")
这两个方法非常适合用于检查字符串的特定部分。
四、使用正则表达式
正则表达式非常适合用于复杂的字符串匹配。Python的re
模块提供了对正则表达式的支持。
import re
pattern = r'\bworld\b'
text = 'hello world, hello Python'
matches = re.findall(pattern, text)
print(matches)
在这个例子中,re.findall()
函数返回一个列表,包含所有匹配的子串。
五、正则表达式详细介绍
正则表达式是一种强大的字符串匹配工具。下面将详细介绍一些常用的正则表达式模式和函数。
1、基本模式
.
匹配任意单个字符^
匹配字符串的开头$
匹配字符串的结尾*
匹配前面的字符零次或多次+
匹配前面的字符一次或多次?
匹配前面的字符零次或一次{n}
匹配前面的字符n次{n,}
匹配前面的字符至少n次{n,m}
匹配前面的字符n到m次[]
匹配字符集中的任意一个字符|
表示或操作()
用于分组
2、常用函数
re.match(pattern, string)
从字符串的开头匹配模式re.search(pattern, string)
搜索字符串中第一次出现的模式re.findall(pattern, string)
查找字符串中所有非重叠的模式re.finditer(pattern, string)
返回一个迭代器,包含字符串中所有非重叠的模式re.sub(pattern, repl, string)
替换字符串中所有匹配的模式
六、使用re模块的示例
以下是一些使用re模块的示例代码:
import re
匹配字符串开头
pattern = r'^hello'
text = 'hello world'
if re.match(pattern, text):
print("String starts with 'hello'")
搜索字符串中第一次出现的模式
pattern = r'world'
text = 'hello world, hello Python'
match = re.search(pattern, text)
if match:
print(f"Found 'world' at index {match.start()}")
查找字符串中所有非重叠的模式
pattern = r'\bhello\b'
text = 'hello world, hello Python'
matches = re.findall(pattern, text)
print(matches)
替换字符串中所有匹配的模式
pattern = r'hello'
repl = 'hi'
text = 'hello world, hello Python'
new_text = re.sub(pattern, repl, text)
print(new_text)
在这些示例中,我们展示了如何使用re模块的不同函数来进行字符串匹配和替换。
七、总结
Python提供了多种字符串匹配的方法,包括find()方法、in关键字、startswith()方法、endswith()方法和正则表达式。每种方法都有其优缺点,适用于不同的场景。正则表达式 是一种强大的工具,适用于复杂的字符串匹配。通过学习和掌握这些方法,可以有效地解决各种字符串匹配的问题。
相关问答FAQs:
如何在Python中检查一个字符串是否包含另一个字符串?
在Python中,可以使用in
关键字来检查一个字符串是否包含另一个字符串。示例如下:
main_string = "Hello, welcome to Python programming."
substring = "Python"
if substring in main_string:
print("Substring found!")
else:
print("Substring not found.")
这种方法简洁明了,适合快速检查。
在Python中,使用正则表达式如何进行复杂的字符串匹配?
Python的re
模块提供了强大的正则表达式支持,可以用于复杂的字符串匹配。以下是一个示例:
import re
text = "There are many programming languages, including Python and Java."
pattern = r'Python|Java'
matches = re.findall(pattern, text)
print(matches) # 输出: ['Python', 'Java']
使用正则表达式,可以实现更灵活的匹配规则。
如何在Python中替换字符串中的特定字符或子字符串?
可以使用字符串对象的replace()
方法轻松替换字符串中的特定字符或子字符串。示例如下:
original_string = "I love Python programming."
new_string = original_string.replace("Python", "Java")
print(new_string) # 输出: I love Java programming.
这个方法非常适合需要进行简单替换的场景,返回一个新的字符串。
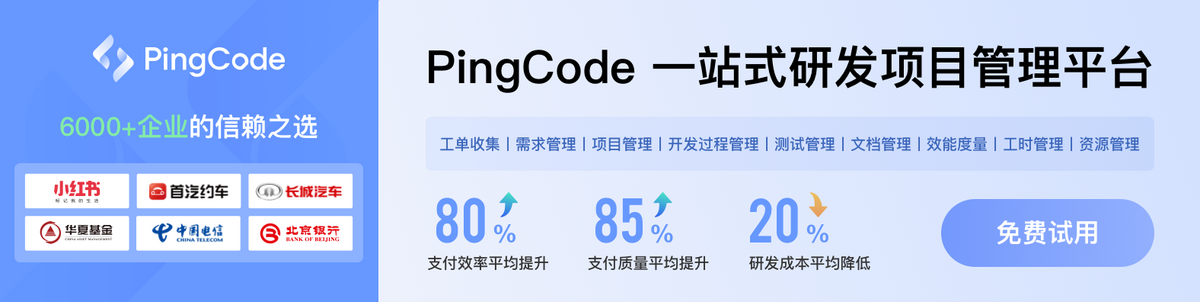