Python读取GB2313文本的方法有很多种,主要包括以下几种:使用open函数、使用pandas库、使用codecs模块、使用io模块。 我们将详细介绍其中的一种方法:使用open函数。通过这种方法,我们可以轻松读取GB2313编码的文本文件,并进行相关的处理。
为了更详细地理解这些方法,下面将介绍每种方法的具体使用步骤和示例代码。
一、使用open函数读取GB2313编码文本
使用Python自带的open函数读取GB2313编码的文本文件是最基础的方法。该方法主要通过指定编码参数来读取不同编码的文本文件。下面是详细的操作步骤和示例代码。
1、指定编码参数读取文件
首先,我们需要确保文本文件的编码是GB2313。然后,我们可以通过open函数的encoding参数指定编码类型,从而正确读取文件的内容。
def read_gb2313_file(file_path):
with open(file_path, 'r', encoding='gb2313') as file:
content = file.read()
return content
示例
file_path = 'path/to/your/gb2313_encoded_file.txt'
content = read_gb2313_file(file_path)
print(content)
2、处理读取的文本内容
读取GB2313编码的文本文件后,我们可以对内容进行进一步的处理,例如:文本分析、数据提取、格式转换等。
def process_text(content):
# 进行文本处理
processed_content = content.replace('\n', ' ')
return processed_content
示例
processed_content = process_text(content)
print(processed_content)
二、使用pandas库读取GB2313编码文本
Pandas是Python中常用的数据处理库,可以方便地读取和处理各种格式的数据文件。通过pandas库的read_csv函数,我们可以读取GB2313编码的CSV文件。
1、导入pandas库
首先,需要确保已经安装了pandas库。如果尚未安装,可以通过以下命令安装:
pip install pandas
2、读取GB2313编码的CSV文件
使用pandas库的read_csv函数读取GB2313编码的CSV文件,并指定encoding参数为'gb2313'。
import pandas as pd
def read_gb2313_csv(file_path):
df = pd.read_csv(file_path, encoding='gb2313')
return df
示例
file_path = 'path/to/your/gb2313_encoded_file.csv'
df = read_gb2313_csv(file_path)
print(df)
3、处理DataFrame数据
读取CSV文件后,我们可以使用pandas库提供的丰富函数对DataFrame进行进一步处理,例如:数据筛选、数据清洗、数据分析等。
def process_dataframe(df):
# 进行数据处理
df = df.dropna() # 删除缺失值
return df
示例
processed_df = process_dataframe(df)
print(processed_df)
三、使用codecs模块读取GB2313编码文本
Python的codecs模块提供了对不同编码类型的支持,可以方便地读取和写入各种编码格式的文件。通过codecs模块,我们可以轻松读取GB2313编码的文本文件。
1、导入codecs模块
首先,需要导入codecs模块。
import codecs
2、使用codecs模块读取GB2313编码的文本文件
使用codecs模块的open函数读取GB2313编码的文本文件,并指定encoding参数为'gb2313'。
def read_gb2313_file_with_codecs(file_path):
with codecs.open(file_path, 'r', encoding='gb2313') as file:
content = file.read()
return content
示例
file_path = 'path/to/your/gb2313_encoded_file.txt'
content = read_gb2313_file_with_codecs(file_path)
print(content)
3、处理读取的文本内容
读取GB2313编码的文本文件后,可以对内容进行进一步处理,例如:文本分析、数据提取、格式转换等。
def process_text_with_codecs(content):
# 进行文本处理
processed_content = content.replace('\n', ' ')
return processed_content
示例
processed_content = process_text_with_codecs(content)
print(processed_content)
四、使用io模块读取GB2313编码文本
Python的io模块提供了对文件操作的支持。通过io模块中的open函数,我们可以读取GB2313编码的文本文件。
1、导入io模块
首先,需要导入io模块。
import io
2、使用io模块读取GB2313编码的文本文件
使用io模块的open函数读取GB2313编码的文本文件,并指定encoding参数为'gb2313'。
def read_gb2313_file_with_io(file_path):
with io.open(file_path, 'r', encoding='gb2313') as file:
content = file.read()
return content
示例
file_path = 'path/to/your/gb2313_encoded_file.txt'
content = read_gb2313_file_with_io(file_path)
print(content)
3、处理读取的文本内容
读取GB2313编码的文本文件后,可以对内容进行进一步处理,例如:文本分析、数据提取、格式转换等。
def process_text_with_io(content):
# 进行文本处理
processed_content = content.replace('\n', ' ')
return processed_content
示例
processed_content = process_text_with_io(content)
print(processed_content)
总结
本文详细介绍了Python读取GB2313编码文本的四种方法:使用open函数、使用pandas库、使用codecs模块、使用io模块。每种方法都提供了具体的操作步骤和示例代码,方便读者根据实际需求选择合适的方法。
在实际应用中,可以根据文件类型、数据处理需求和个人习惯选择不同的方法。希望通过本文的介绍,读者能够掌握Python读取GB2313编码文本的多种方法,并能在实际项目中灵活应用。
相关问答FAQs:
Python可以读取gb2312编码的文本文件吗?
是的,Python提供了对多种字符编码的支持,包括gb2312。可以使用内置的open
函数,指定编码为'gb2312'
来读取相应的文本文件。例如:
with open('your_file.txt', 'r', encoding='gb2312') as file:
content = file.read()
print(content)
这样就可以正确读取gb2312编码的内容。
如何处理gb2312编码文本中的乱码问题?
在读取gb2312编码的文本文件时,如果出现乱码,首先要确认文件确实是使用该编码格式保存的。可以尝试使用errors='replace'
参数来处理读取时的错误。示例代码如下:
with open('your_file.txt', 'r', encoding='gb2312', errors='replace') as file:
content = file.read()
print(content)
这样,无法解码的字符将被替换为‘?’,从而避免程序崩溃。
可以使用Python读取其他编码格式的gb2312文本吗?
当然可以。Python支持多种编码格式的文本文件读取。只需在open
函数中将encoding
参数设置为相应的编码格式,比如'utf-8'
、'iso-8859-1'
等。读取时注意确保指定的编码与文件实际编码一致,以避免乱码。示例如下:
with open('your_file.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
这样就可以读取使用utf-8编码的文件。
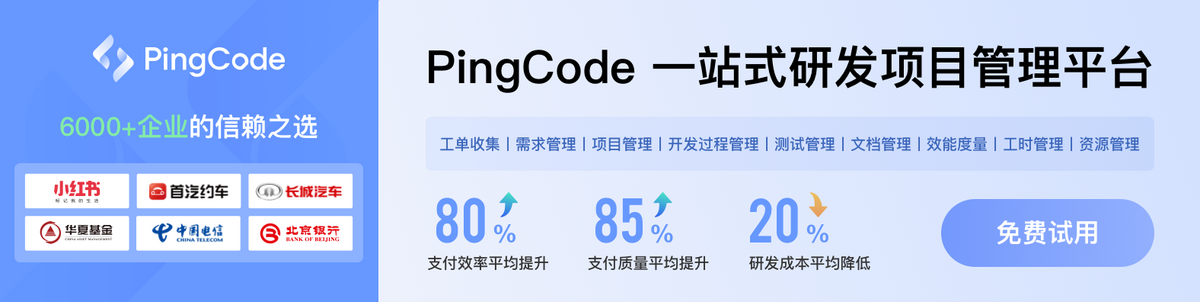