Python3 查找字符串的方法包括:使用find()方法、使用index()方法、使用in关键字、使用正则表达式。 其中,使用find()方法是最常见和推荐的方式,因为它在找不到字符串时会返回-1,而不会引发异常。
text = "Hello, welcome to the world of Python"
substring = "welcome"
position = text.find(substring)
if position != -1:
print(f"Substring found at index {position}")
else:
print("Substring not found")
一、FIND()方法
find()方法是Python内置的查找字符串子串的函数。它返回子串在字符串中的最低索引位置。如果子串不在字符串中,则返回-1。这种方法不会引发异常,这使得它在查找字符串子串时非常安全和高效。
text = "Hello, welcome to the world of Python"
substring = "world"
使用 find() 方法查找子串
position = text.find(substring)
if position != -1:
print(f"Substring '{substring}' found at index {position}")
else:
print(f"Substring '{substring}' not found")
在上面的例子中,find()方法成功找到了子串"world",并返回其在字符串中的位置。find()方法还允许我们指定开始和结束位置,从而在特定范围内查找子串。
# 指定查找范围
position = text.find(substring, 15, 30)
if position != -1:
print(f"Substring '{substring}' found at index {position} in specified range")
else:
print(f"Substring '{substring}' not found in specified range")
在这个例子中,我们指定了查找范围为索引15到30之间。
二、INDEX()方法
index()方法与find()方法类似,但如果子串不在字符串中,它会引发ValueError异常。这种方法适用于我们需要确保子串一定存在的情况。
text = "Hello, welcome to the world of Python"
substring = "Python"
try:
position = text.index(substring)
print(f"Substring '{substring}' found at index {position}")
except ValueError:
print(f"Substring '{substring}' not found")
在这个例子中,index()方法成功找到了子串"Python"。如果子串不存在,index()方法会引发异常,我们可以通过try-except块进行捕获和处理。
三、使用IN关键字
使用in关键字是最简单和最直观的方式来查找字符串子串。它返回一个布尔值,表示子串是否存在于字符串中。
text = "Hello, welcome to the world of Python"
substring = "welcome"
if substring in text:
print(f"Substring '{substring}' is present in the text")
else:
print(f"Substring '{substring}' is not present in the text")
在这个例子中,in关键字成功检测到子串"welcome"存在于字符串中。使用in关键字非常高效,因为它不需要我们关心子串的具体位置,只需要知道子串是否存在。
四、使用正则表达式
正则表达式是一种强大的字符串匹配工具,适用于复杂的查找条件。Python的re模块提供了丰富的正则表达式功能。
import re
text = "Hello, welcome to the world of Python"
pattern = r"w\w+"
使用 re.search() 方法查找符合模式的子串
match = re.search(pattern, text)
if match:
print(f"Pattern found: {match.group()} at index {match.start()}")
else:
print("Pattern not found")
在这个例子中,我们使用正则表达式模式"w\w+"来查找以'w'开头的单词。re.search()方法返回一个匹配对象,如果模式未找到则返回None。我们可以通过匹配对象获取匹配的子串及其位置。
五、综合运用多种方法
在实际应用中,我们可能需要综合运用多种方法来查找字符串。不同的方法有各自的优缺点,我们可以根据具体需求选择合适的方法。
text = "Hello, welcome to the world of Python"
substring = "Python"
使用 find() 方法
position = text.find(substring)
if position != -1:
print(f"Using find(): Substring '{substring}' found at index {position}")
使用 index() 方法
try:
position = text.index(substring)
print(f"Using index(): Substring '{substring}' found at index {position}")
except ValueError:
print(f"Using index(): Substring '{substring}' not found")
使用 in 关键字
if substring in text:
print(f"Using in keyword: Substring '{substring}' is present in the text")
else:
print(f"Using in keyword: Substring '{substring}' is not present in the text")
使用正则表达式
pattern = r"Python"
match = re.search(pattern, text)
if match:
print(f"Using regex: Pattern found: {match.group()} at index {match.start()}")
else:
print("Using regex: Pattern not found")
在这个综合例子中,我们分别使用了find()、index()、in关键字和正则表达式来查找子串"Python"。这种多种方法的综合运用可以确保我们能够灵活应对各种查找需求。
相关问答FAQs:
在Python3中,如何高效地查找字符串?
在Python3中,可以使用字符串的内置方法如find()
、index()
和count()
来查找字符串。find()
方法会返回子字符串首次出现的位置,如果未找到则返回-1;而index()
方法在未找到时会引发异常。此外,count()
方法可以用来计算子字符串在字符串中出现的次数。这些方法简单易用,适合不同的查找需求。
如果我想在字符串中查找多个子字符串,有什么推荐的方法吗?
可以使用循环结合上面提到的方法来查找多个子字符串。另一种方法是使用正则表达式模块re
,它提供了强大的字符串查找功能。通过re.findall()
可以同时查找多个模式,返回所有匹配的结果。这样不仅可以提升查找的灵活性,还能实现复杂的字符串处理。
在查找字符串时,如何忽略大小写?
要在查找字符串时忽略大小写,可以将待查找的字符串和目标字符串都转换为相同的大小写格式,通常是使用lower()
或upper()
方法。例如,可以使用string.lower().find(substring.lower())
来进行查找,这样就能确保大小写不影响查找结果。这样的方法简单而有效,适用于大多数场景。
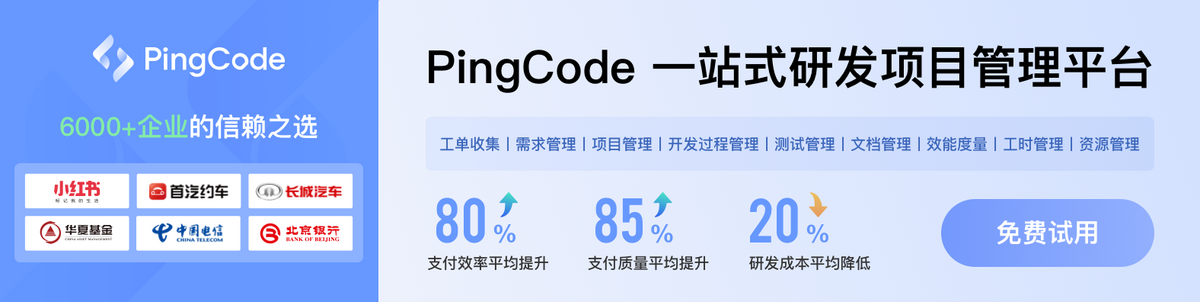