要用Python写一个秒杀程序,你需要掌握以下几个核心点:网络请求、并发处理、反爬虫策略、数据处理。 其中,网络请求和并发处理是最重要的部分,它们直接决定了程序的效率和成功率。接下来,我们将详细介绍这些要点,并提供具体实现的方法。
一、网络请求
在秒杀程序中,网络请求是用来与服务器进行交互的关键部分。你需要使用Python的requests
库来模拟HTTP请求,完成登录、获取商品信息、提交订单等操作。
1、使用Requests库
requests
是一个简单易用的HTTP库,适合处理网络请求。你可以使用它来发送GET和POST请求。以下是一个简单的例子:
import requests
发送GET请求
response = requests.get('https://example.com/product')
print(response.text)
发送POST请求
data = {'username': 'user', 'password': 'pass'}
response = requests.post('https://example.com/login', data=data)
print(response.json())
在实际的秒杀程序中,你需要根据具体的需求来构造请求,例如设置请求头、携带Cookies等。
2、处理Cookies和Session
在秒杀过程中,处理Cookies和Session是非常重要的,因为大多数网站会使用这些机制来管理用户的登录状态和购物车信息。你可以使用requests.Session
来保持会话:
session = requests.Session()
登录并保持会话
login_data = {'username': 'user', 'password': 'pass'}
session.post('https://example.com/login', data=login_data)
使用会话获取商品信息
response = session.get('https://example.com/product')
print(response.text)
二、并发处理
秒杀的关键在于抢占资源,因此并发处理至关重要。你可以使用Python的concurrent.futures
库或threading
库来实现并发请求。
1、使用ThreadPoolExecutor
concurrent.futures.ThreadPoolExecutor
是一个简单易用的线程池,可以用来管理多个线程并发执行任务。以下是一个简单的例子:
import concurrent.futures
import requests
def send_request(url):
response = requests.get(url)
print(response.text)
urls = ['https://example.com/product1', 'https://example.com/product2']
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(send_request, url) for url in urls]
concurrent.futures.wait(futures)
2、使用Threading库
threading
库提供了更底层的线程控制,可以用于实现更复杂的并发逻辑。以下是一个简单的例子:
import threading
import requests
def send_request(url):
response = requests.get(url)
print(response.text)
urls = ['https://example.com/product1', 'https://example.com/product2']
threads = []
for url in urls:
thread = threading.Thread(target=send_request, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
三、反爬虫策略
大多数电商平台都会有反爬虫机制,因此你需要采取一些策略来绕过这些限制,例如设置请求头、使用代理、模拟用户行为等。
1、设置请求头
通过设置请求头,你可以伪装成正常的浏览器请求。以下是一个示例:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.75 Safari/537.36',
'Referer': 'https://example.com'
}
response = requests.get('https://example.com/product', headers=headers)
print(response.text)
2、使用代理
使用代理可以隐藏你的真实IP地址,从而避免被服务器封禁。以下是一个示例:
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get('https://example.com', proxies=proxies)
print(response.text)
3、模拟用户行为
通过模拟用户行为(例如随机等待、点击操作等),你可以进一步降低被反爬虫机制检测到的风险。以下是一个示例:
import time
import random
随机等待
time.sleep(random.uniform(1, 3))
response = requests.get('https://example.com/product')
print(response.text)
四、数据处理
在秒杀程序中,你需要处理从服务器返回的数据,例如解析商品信息、提取库存状态等。你可以使用BeautifulSoup
或lxml
等库来解析HTML。
1、使用BeautifulSoup
BeautifulSoup
是一个强大的HTML解析库,适合处理复杂的HTML结构。以下是一个示例:
from bs4 import BeautifulSoup
html = '<html><body><h1>Product</h1><p>Price: $10</p></body></html>'
soup = BeautifulSoup(html, 'html.parser')
提取商品名称
product_name = soup.find('h1').text
print(product_name)
提取价格
price = soup.find('p').text
print(price)
2、使用lxml
lxml
是另一个强大的HTML解析库,适合处理大规模数据。以下是一个示例:
from lxml import etree
html = '<html><body><h1>Product</h1><p>Price: $10</p></body></html>'
tree = etree.HTML(html)
提取商品名称
product_name = tree.xpath('//h1/text()')[0]
print(product_name)
提取价格
price = tree.xpath('//p/text()')[0]
print(price)
五、完整的秒杀程序示例
结合以上内容,我们可以编写一个简单的秒杀程序。以下是一个完整的示例:
import requests
import concurrent.futures
import random
import time
from bs4 import BeautifulSoup
登录并保持会话
session = requests.Session()
login_data = {'username': 'user', 'password': 'pass'}
session.post('https://example.com/login', data=login_data)
获取商品信息
response = session.get('https://example.com/product')
soup = BeautifulSoup(response.text, 'html.parser')
product_name = soup.find('h1').text
price = soup.find('p').text
print(f'Product: {product_name}, Price: {price}')
并发发送秒杀请求
def send_request():
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.75 Safari/537.36',
'Referer': 'https://example.com'
}
# 随机等待
time.sleep(random.uniform(1, 3))
response = session.post('https://example.com/buy', headers=headers)
print(response.text)
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(send_request) for _ in range(10)]
concurrent.futures.wait(futures)
总结
编写一个秒杀程序需要你掌握网络请求、并发处理、反爬虫策略和数据处理等技能。通过使用requests
库进行网络请求、concurrent.futures
库进行并发处理,以及采用合适的反爬虫策略和数据处理方法,你可以编写一个高效的秒杀程序。希望这篇文章对你有所帮助,祝你秒杀成功!
相关问答FAQs:
如何开始使用Python编写秒杀程序?
编写秒杀程序的第一步是了解基本的Python编程知识,包括数据结构、网络请求和多线程处理。你需要熟悉Python的库,如Requests和Threading,以便能够模拟用户请求并处理并发访问。可以从简单的项目开始,逐步增加复杂度。
秒杀程序需要考虑哪些关键因素?
在设计秒杀程序时,关键因素包括请求的速率限制、处理高并发的能力和数据的一致性。你需要考虑如何实现高效的请求发送,同时避免被服务器检测到异常流量。此外,考虑使用缓存机制来提高响应速度,确保在高并发情况下依然能够提供流畅的用户体验。
有哪些常用的Python库可以帮助实现秒杀功能?
实现秒杀功能时,常用的Python库包括Requests(用于发送HTTP请求)、BeautifulSoup(用于解析HTML页面)、以及Asyncio(用于处理异步操作)。这些库能够帮助你更高效地处理网络请求和数据解析,提高程序的性能和稳定性。了解这些工具的使用方法将使你在开发过程中更加得心应手。
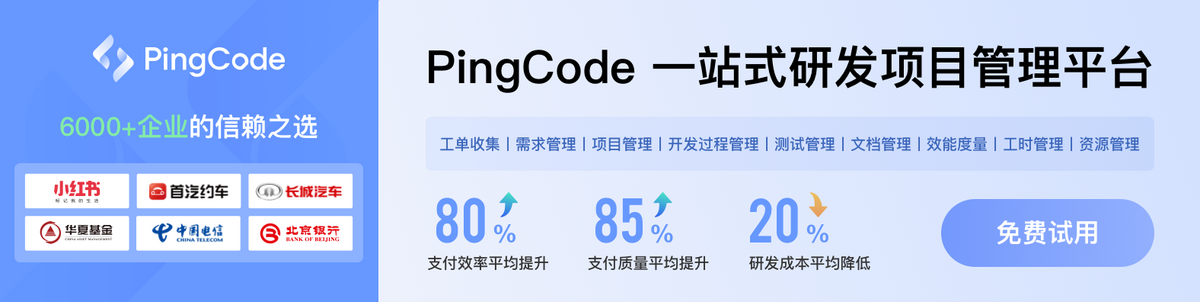