要从Python字符串中提取数字,可以使用正则表达式、字符串方法、列表解析等方法。最常用且强大的方法是使用正则表达式。本文将详细介绍几种方法,重点讲解正则表达式的使用。
使用正则表达式时,通过re
模块,我们可以轻松找到字符串中的数字。正则表达式强大且灵活,适用于各种复杂的匹配需求。下面将详细介绍如何使用正则表达式以及其他方法从字符串中提取数字。
一、正则表达式提取数字
正则表达式(Regular Expression,简称regex)是一种描述字符模式的特殊语法,用于查找和匹配字符串中的子串。Python通过re
模块提供对正则表达式的支持。
1. 安装和导入re
模块
re
模块是Python的内置模块,无需安装,只需导入即可使用。
import re
2. 使用re.findall()
方法
re.findall(pattern, string)
方法用于在字符串中查找所有与模式匹配的子串,并以列表形式返回。
import re
示例字符串
text = "The price is 100 dollars, and the discount is 20%."
正则表达式模式
pattern = r'\d+'
提取数字
numbers = re.findall(pattern, text)
print(numbers) # 输出:['100', '20']
详细描述:
\d
表示匹配一个数字字符。+
表示匹配前面的字符一次或多次。
二、使用字符串方法提取数字
Python的字符串方法也可以用于提取数字,尽管不如正则表达式灵活,但在简单场景下同样有效。
1. 使用字符串遍历和条件判断
通过遍历字符串中的每个字符,判断其是否为数字。
# 示例字符串
text = "The price is 100 dollars, and the discount is 20%."
存储数字
numbers = []
遍历字符串
for char in text:
if char.isdigit():
numbers.append(char)
print(numbers) # 输出:['1', '0', '0', '2', '0']
详细描述:
char.isdigit()
方法用于判断字符是否为数字。
2. 使用列表解析
列表解析是一种简洁优雅的方法,可以将遍历和条件判断结合在一行代码中完成。
# 示例字符串
text = "The price is 100 dollars, and the discount is 20%."
提取数字
numbers = [char for char in text if char.isdigit()]
print(numbers) # 输出:['1', '0', '0', '2', '0']
详细描述:
- 列表解析使代码更简洁,可读性更高。
三、结合方法提取数字
在实际应用中,常常需要提取完整的数字(而不是单个字符),这时可以结合使用字符串方法和正则表达式。
1. 提取完整数字
通过字符串拆分和条件判断,提取完整的数字。
# 示例字符串
text = "The price is 100 dollars, and the discount is 20%."
拆分字符串
parts = text.split()
存储数字
numbers = []
遍历每个部分
for part in parts:
if part.isdigit():
numbers.append(part)
print(numbers) # 输出:['100', '20']
详细描述:
split()
方法将字符串拆分为单词列表。part.isdigit()
判断每个单词是否为数字。
四、使用更复杂的正则表达式模式
在处理更复杂的字符串时,可以使用更复杂的正则表达式模式。例如,提取包含小数点的数字。
1. 提取包含小数点的数字
import re
示例字符串
text = "The price is 100.50 dollars, and the discount is 20.5%."
正则表达式模式
pattern = r'\d+\.?\d*'
提取数字
numbers = re.findall(pattern, text)
print(numbers) # 输出:['100.50', '20.5']
详细描述:
\d+
匹配一个或多个数字。\.?
匹配零个或一个小数点。\d*
匹配零个或多个数字。
五、从字符串中提取数字并转换为整数或浮点数
有时提取数字后需要将其转换为整数或浮点数,以便进行数学运算。
1. 提取并转换为整数
import re
示例字符串
text = "The price is 100 dollars, and the discount is 20%."
提取数字
pattern = r'\d+'
numbers = re.findall(pattern, text)
转换为整数
int_numbers = list(map(int, numbers))
print(int_numbers) # 输出:['100', '20']
详细描述:
map(int, numbers)
将提取的数字列表中的每个元素转换为整数。
2. 提取并转换为浮点数
import re
示例字符串
text = "The price is 100.50 dollars, and the discount is 20.5%."
提取数字
pattern = r'\d+\.?\d*'
numbers = re.findall(pattern, text)
转换为浮点数
float_numbers = list(map(float, numbers))
print(float_numbers) # 输出:['100.50', '20.5']
详细描述:
map(float, numbers)
将提取的数字列表中的每个元素转换为浮点数。
六、从字符串中提取负数
在某些场景中,需要从字符串中提取负数。此时可以使用带有负号的正则表达式模式。
1. 提取负数
import re
示例字符串
text = "The temperature is -5 degrees, and the wind speed is -20 km/h."
正则表达式模式
pattern = r'-?\d+\.?\d*'
提取数字
numbers = re.findall(pattern, text)
print(numbers) # 输出:['-5', '-20']
详细描述:
-?
匹配零个或一个负号。- 其他部分与前面类似。
七、结论
通过以上几种方法,可以轻松从Python字符串中提取数字。正则表达式方法最为强大,适用于各种复杂的匹配需求;字符串方法和列表解析适用于简单场景;结合方法可以提取完整数字;更复杂的正则表达式模式可以处理小数和负数。根据具体需求选择合适的方法,可以高效地完成任务。
相关问答FAQs:
如何在Python中提取字符串中的所有数字?
在Python中,可以使用正则表达式来提取字符串中的所有数字。使用re
模块的findall
方法,可以轻松实现。例如,re.findall(r'\d+', string)
可以返回字符串中所有的数字。你可以将这些数字存储在列表中,以便后续处理。
提取数字时如何处理小数和负数?
如果需要提取小数和负数,可以稍微修改正则表达式。对于小数,可以使用r'-?\d+(\.\d+)?'
,这个表达式将匹配可能带有负号的整数和小数。使用这种方式,你可以确保提取到的数字包含更复杂的格式。
如何将提取到的数字转换为整数或浮点数?
提取到的数字通常以字符串形式存在,若需要将它们转换为整数或浮点数,可以使用int()
或float()
函数进行转换。例如,int(num)
将字符串形式的数字转换为整数,而float(num)
则将其转换为浮点数。可以在提取后遍历数字列表进行转换。
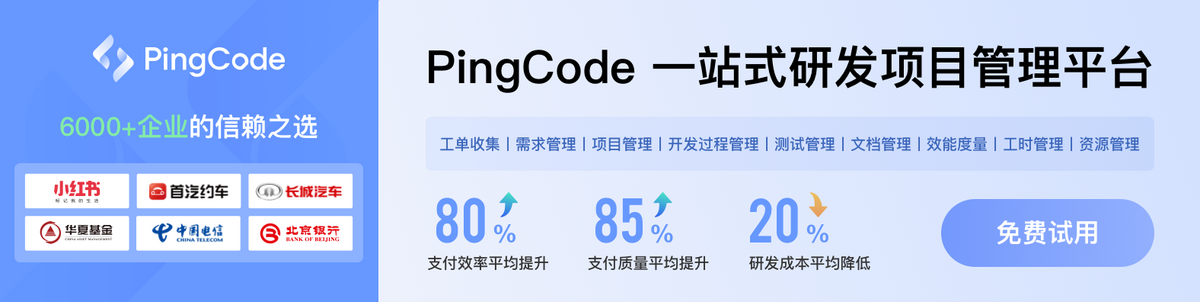