Python字符串如何赋值给变量赋值
在Python中,字符串可以通过直接赋值、使用字符串格式化、字符串拼接等方式赋值给变量。直接赋值、字符串格式化、字符串拼接是常见的方法。以下是详细介绍:
一、直接赋值
直接赋值是最简单且最常见的赋值方法。通过将字符串放在引号(单引号、双引号或三引号)中,然后赋值给变量即可。
# 使用单引号
str1 = 'Hello, World!'
使用双引号
str2 = "Python Programming"
使用三引号
str3 = '''This is a
multiline string.'''
这种方法简单明了,适用于大部分场景。
二、字符串格式化
字符串格式化是将变量值插入字符串中。Python提供了多种字符串格式化方式,如使用百分号 %
、str.format()
方法和 f-strings。
1. 使用百分号 %
name = 'John'
age = 30
greeting = 'Hello, %s! You are %d years old.' % (name, age)
2. 使用 str.format()
name = 'John'
age = 30
greeting = 'Hello, {}! You are {} years old.'.format(name, age)
3. 使用 f-strings
name = 'John'
age = 30
greeting = f'Hello, {name}! You are {age} years old.'
f-strings(格式化字符串字面值)是Python 3.6引入的,提供了更简洁的语法。
三、字符串拼接
字符串拼接是将多个字符串连接在一起形成一个新的字符串,并赋值给变量。可以使用加号 +
或 join()
方法进行拼接。
1. 使用加号 +
str1 = 'Hello'
str2 = 'World'
combined_str = str1 + ', ' + str2 + '!'
2. 使用 join()
parts = ['Hello', 'World']
combined_str = ', '.join(parts) + '!'
四、从用户输入获取字符串
通过 input()
函数从用户获取输入字符串,并赋值给变量。
user_input = input('Enter a string: ')
print(f'You entered: {user_input}')
五、从文件读取字符串
读取文件中的字符串,并赋值给变量。
# 打开文件并读取内容
with open('example.txt', 'r') as file:
file_content = file.read()
print(file_content)
六、从函数返回值获取字符串
通过定义函数,返回字符串,并赋值给变量。
def get_greeting(name):
return f'Hello, {name}!'
greeting = get_greeting('Alice')
print(greeting)
七、使用字符串常量和模板
Python中的 string
模块提供了常量和模板,可以用于字符串赋值。
1. 使用字符串常量
import string
alphabet = string.ascii_letters
print(alphabet)
2. 使用模板字符串
from string import Template
template = Template('Hello, $name!')
greeting = template.substitute(name='Alice')
print(greeting)
八、结合各种方法进行复杂赋值
在实际编程中,可能需要结合多种方法进行字符串赋值。
name = 'Bob'
age = 25
使用 f-strings 和拼接
intro = f'My name is {name} and I am {age} years old.'
details = 'I love programming in Python.'
结合字符串拼接
profile = intro + ' ' + details
print(profile)
九、处理特殊字符和转义字符
当字符串中包含特殊字符时,需要使用转义字符。
# 使用反斜杠进行转义
str_with_quotes = 'He said, "Python is amazing!"'
str_with_newline = 'Hello,\nWorld!'
print(str_with_quotes)
print(str_with_newline)
十、使用多行字符串
多行字符串使用三引号(单引号或双引号)。
multi_line_str = """This is a
multi-line string
in Python."""
print(multi_line_str)
十一、字符串操作和方法
Python字符串提供了丰富的方法,可以直接操作字符串,并赋值给变量。
1. 转换大小写
original_str = 'Hello, World!'
upper_str = original_str.upper()
lower_str = original_str.lower()
title_str = original_str.title()
print(upper_str)
print(lower_str)
print(title_str)
2. 去除空白字符
str_with_spaces = ' Hello, World! '
trimmed_str = str_with_spaces.strip()
print(trimmed_str)
3. 查找和替换
original_str = 'Hello, World!'
replaced_str = original_str.replace('World', 'Python')
print(replaced_str)
十二、结合数据结构
结合列表、字典等数据结构,可以更灵活地操作字符串并赋值。
1. 列表和字符串
words = ['Hello', 'World']
sentence = ' '.join(words)
print(sentence)
2. 字典和字符串格式化
info = {'name': 'Alice', 'age': 30}
formatted_str = 'Name: {name}, Age: {age}'.format(info)
print(formatted_str)
十三、使用正则表达式
通过 re
模块,可以使用正则表达式处理字符串,并赋值给变量。
import re
text = 'The quick brown fox jumps over the lazy dog.'
pattern = r'\b\w{4}\b'
matches = re.findall(pattern, text)
print(matches)
十四、处理字符串编码
在处理不同编码的字符串时,可以使用 encode()
和 decode()
方法。
original_str = 'Hello, World!'
encoded_str = original_str.encode('utf-8')
decoded_str = encoded_str.decode('utf-8')
print(decoded_str)
十五、处理字符串切片
通过字符串切片,可以提取字符串的子字符串。
original_str = 'Hello, World!'
sub_str = original_str[7:12]
print(sub_str)
十六、总结
综上所述,Python提供了多种方式来赋值字符串给变量,包括直接赋值、字符串格式化、字符串拼接等。每种方法都有其适用场景,灵活运用这些方法可以提高代码的可读性和效率。了解并掌握这些技巧,将有助于更高效地进行字符串操作和处理。
相关问答FAQs:
如何在Python中创建一个字符串变量?
在Python中,可以通过直接使用等号(=)来给变量赋值字符串。例如,你可以这样写:my_string = "Hello, World!"
。这行代码将字符串"Hello, World!"赋值给变量my_string
,你可以随时使用这个变量来访问或操作该字符串。
在Python中可以使用哪些方法来操作字符串?
Python提供了丰富的字符串方法,允许用户进行多种操作。常用的方法包括upper()
将字符串转换为大写,lower()
将其转换为小写,replace(old, new)
替换字符串中的某个部分,以及split(separator)
根据指定分隔符拆分字符串等。这些方法使得处理字符串变得非常灵活和方便。
如何在Python中检查字符串的类型或内容?
要检查一个变量是否为字符串类型,可以使用isinstance()
函数。例如,isinstance(my_string, str)
将返回True
如果my_string
是字符串类型。若要检查字符串是否包含特定的子字符串,可以使用in
运算符,如"Hello" in my_string
,这将返回True
如果my_string
中包含"Hello"。这些检查有助于确保代码的健壮性。
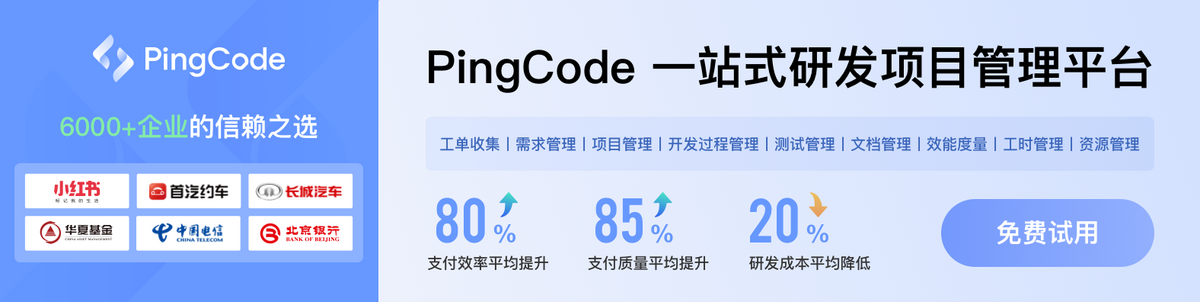