在Python中,继承不同的父类可以通过多重继承来实现、多重继承可以通过在类定义中列出多个父类名来实现、通过super()函数调用父类的初始化方法。在Python中,继承是面向对象编程的一个重要特性,它允许一个类(子类)从另一个类(父类)继承属性和方法。Python还支持多重继承,即一个类可以从多个父类继承属性和方法。下面将详细描述多重继承的实现方法及其注意事项。
一、多重继承的实现
多重继承可以通过在类定义中列出多个父类名来实现。下面是一个简单的示例:
class Parent1:
def __init__(self):
self.parent1_attribute = "Parent1 Attribute"
def parent1_method(self):
print("Parent1 Method")
class Parent2:
def __init__(self):
self.parent2_attribute = "Parent2 Attribute"
def parent2_method(self):
print("Parent2 Method")
class Child(Parent1, Parent2):
def __init__(self):
Parent1.__init__(self)
Parent2.__init__(self)
child = Child()
print(child.parent1_attribute)
print(child.parent2_attribute)
child.parent1_method()
child.parent2_method()
在上面的示例中,Child
类同时继承了Parent1
和Parent2
类,并在其构造函数中分别调用了两个父类的构造函数,以确保父类的属性被正确初始化。
二、使用super()函数调用父类的初始化方法
在多重继承的场景中,使用super()
函数调用父类的初始化方法可以避免显式调用每个父类的构造函数。super()
函数会按照方法解析顺序(Method Resolution Order,MRO)调用父类的方法。以下是一个示例:
class Parent1:
def __init__(self):
self.parent1_attribute = "Parent1 Attribute"
print("Parent1 __init__ called")
class Parent2:
def __init__(self):
self.parent2_attribute = "Parent2 Attribute"
print("Parent2 __init__ called")
class Child(Parent1, Parent2):
def __init__(self):
super().__init__()
print("Child __init__ called")
child = Child()
print(child.parent1_attribute)
print(child.parent2_attribute)
在上面的示例中,super().__init__()
会按照MRO调用Parent1
和Parent2
的构造函数。
三、方法解析顺序(MRO)
在多重继承中,方法解析顺序(MRO)决定了调用父类方法的顺序。Python使用C3线性化算法来计算MRO。可以使用<class>.__mro__
或<class>.mro()
方法查看一个类的MRO。以下是一个示例:
class Parent1:
pass
class Parent2:
pass
class Child(Parent1, Parent2):
pass
print(Child.__mro__)
print(Child.mro())
输出结果为:
(<class '__main__.Child'>, <class '__main__.Parent1'>, <class '__main__.Parent2'>, <class 'object'>)
[<class '__main__.Child'>, <class '__main__.Parent1'>, <class '__main__.Parent2'>, <class 'object'>]
四、菱形继承问题
在多重继承中,如果存在多个父类继承自同一个祖先类,可能会出现菱形继承问题。以下是一个示例:
class Grandparent:
def __init__(self):
print("Grandparent __init__ called")
class Parent1(Grandparent):
def __init__(self):
super().__init__()
print("Parent1 __init__ called")
class Parent2(Grandparent):
def __init__(self):
super().__init__()
print("Parent2 __init__ called")
class Child(Parent1, Parent2):
def __init__(self):
super().__init__()
print("Child __init__ called")
child = Child()
在上面的示例中,Grandparent
类的构造函数会被调用两次。为了避免这种情况,可以使用super()
函数,这样Grandparent
类的构造函数只会被调用一次。
五、实践中的多重继承
在实际开发中,多重继承可以用于实现混入(Mixin)模式。混入是一种设计模式,允许将多个类的功能组合到一个类中,而不需要使用多重继承的复杂性。以下是一个示例:
class LogMixin:
def log(self, message):
print(f"Log: {message}")
class DataMixin:
def save_data(self, data):
print(f"Data saved: {data}")
class Application(LogMixin, DataMixin):
def process_data(self, data):
self.log("Processing data")
self.save_data(data)
app = Application()
app.process_data("Some data")
在上面的示例中,Application
类通过继承LogMixin
和DataMixin
类,实现了日志记录和数据保存的功能。
六、总结
多重继承是Python中的一个强大特性,可以通过在类定义中列出多个父类名来实现。使用super()
函数可以避免显式调用每个父类的构造函数,并按照MRO调用父类的方法。在实际开发中,混入模式可以用于实现多重继承的功能,而不需要使用多重继承的复杂性。理解和正确使用多重继承可以提高代码的可重用性和可维护性。
相关问答FAQs:
在Python中,如何实现多重继承?
多重继承是指一个子类可以继承多个父类。在Python中,可以通过在类定义时将多个父类放在括号内来实现。例如:class ChildClass(ParentClass1, ParentClass2):
。在这种情况下,ChildClass将同时继承ParentClass1和ParentClass2的属性和方法。需要注意的是,使用多重继承时,要确保父类之间没有命名冲突,以避免潜在的混淆。
多重继承可能带来哪些问题?
多重继承可以带来一些复杂性,尤其是当多个父类中存在同名方法时,Python会使用C3线性化算法来决定方法解析顺序(MRO)。这可能导致一些意想不到的行为,因此在设计类结构时,需要仔细考虑父类的选择和它们之间的关系。文档和注释可以帮助提高代码的可读性和可维护性。
如何使用super()函数在多重继承中调用父类的方法?
在多重继承中,可以使用super()
函数来调用父类的方法。通过super()
,可以确保按照MRO顺序调用父类的方法,这样可以避免直接指定父类可能导致的问题。使用方法示例:super(ChildClass, self).method_name()
,这将调用当前类的父类中对应的方法。利用这个特性,可以在复杂的继承层次中保持代码的清晰性和可维护性。
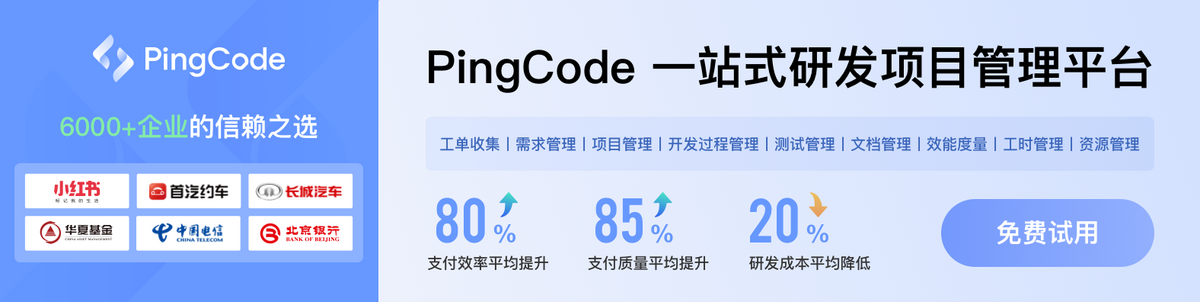