在Python中有多种方法可以在列表中查找元素,包括使用in
关键字、index()
方法、find()
方法(在字符串中)、list comprehension
等。最常用的方法有:使用in
关键字、使用index()
方法、遍历列表、使用filter()
函数。我们将详细展开其中的一些方法。
一、使用in
关键字
使用in
关键字是最简单、直接的方法之一。它可以用于检查某个元素是否存在于列表中,并返回一个布尔值。
my_list = [1, 2, 3, 4, 5]
element = 3
if element in my_list:
print(f"{element} is in the list")
else:
print(f"{element} is not in the list")
详细描述:in
关键字的优点在于其简洁性和直观性。它的时间复杂度为O(n),适合于检查列表中是否存在某个元素。
二、使用index()
方法
index()
方法用于查找元素在列表中的位置,并返回它的索引。如果元素不在列表中,会引发一个ValueError
异常。
my_list = [1, 2, 3, 4, 5]
element = 3
try:
index = my_list.index(element)
print(f"Element {element} found at index {index}")
except ValueError:
print(f"Element {element} is not in the list")
详细描述:index()
方法不仅可以检查元素是否存在,还可以得到元素的位置。它的时间复杂度也是O(n),在需要知道元素位置时非常有用。
三、遍历列表
手动遍历列表是一种灵活但相对较繁琐的方法。这种方法可以在查找过程中执行额外的操作。
my_list = [1, 2, 3, 4, 5]
element = 3
found = False
for i, e in enumerate(my_list):
if e == element:
found = True
print(f"Element {element} found at index {i}")
break
if not found:
print(f"Element {element} is not in the list")
详细描述:手动遍历列表的优点是可以在查找过程中执行自定义操作。虽然这种方法看似繁琐,但在特定场景下是非常有用的。
四、使用filter()
函数
filter()
函数可以用于筛选出所有满足条件的元素。结合lambda
表达式,可以非常方便地查找特定元素。
my_list = [1, 2, 3, 4, 5]
element = 3
filtered_list = list(filter(lambda x: x == element, my_list))
if filtered_list:
print(f"Element {element} found")
else:
print(f"Element {element} is not in the list")
详细描述:filter()
函数适合用于更复杂的条件筛选。它的时间复杂度同样为O(n),但在处理更复杂条件时非常强大。
五、使用列表推导式
列表推导式是一种简洁的方式来创建新的列表。在查找元素时,它可以被用来生成一个包含所有满足条件的元素的子列表。
my_list = [1, 2, 3, 4, 5]
element = 3
found_elements = [x for x in my_list if x == element]
if found_elements:
print(f"Element {element} found")
else:
print(f"Element {element} is not in the list")
详细描述:列表推导式的优点在于其简洁性和可读性。它非常适合用于简单的筛选操作。
六、使用any()
函数
any()
函数可以用来检查可迭代对象中是否存在至少一个满足条件的元素。
my_list = [1, 2, 3, 4, 5]
element = 3
if any(x == element for x in my_list):
print(f"Element {element} found")
else:
print(f"Element {element} is not in the list")
详细描述:any()
函数非常高效,适合用于判断条件是否被满足。它的时间复杂度也是O(n)。
七、使用collections.Counter
collections.Counter
是一个专门用于计数的容器,可以非常方便地统计元素出现的次数。
from collections import Counter
my_list = [1, 2, 3, 4, 5]
element = 3
counter = Counter(my_list)
if counter[element] > 0:
print(f"Element {element} found {counter[element]} time(s)")
else:
print(f"Element {element} is not in the list")
详细描述:Counter
不仅可以检查元素是否存在,还可以统计元素出现的次数。适合用于需要频繁查找和统计的场景。
八、使用numpy
库
对于包含大量数据的列表,使用numpy
库可以显著提高查找效率。
import numpy as np
my_list = [1, 2, 3, 4, 5]
element = 3
np_array = np.array(my_list)
if element in np_array:
print(f"Element {element} found")
else:
print(f"Element {element} is not in the list")
详细描述:numpy
库在处理大数据时非常高效。它使用C语言编写,性能优异。
九、使用pandas
库
pandas
库提供了强大的数据处理功能,适合处理结构化数据。
import pandas as pd
my_list = [1, 2, 3, 4, 5]
element = 3
series = pd.Series(my_list)
if element in series.values:
print(f"Element {element} found")
else:
print(f"Element {element} is not in the list")
详细描述:pandas
库非常适合用于数据分析和处理。它的查找功能强大,适合处理复杂数据。
十、使用自定义函数
根据具体需求,可以编写自定义函数来查找元素。这种方法灵活度最高,可以根据需求进行定制。
def find_element(lst, el):
for i, e in enumerate(lst):
if e == el:
return i
return -1
my_list = [1, 2, 3, 4, 5]
element = 3
index = find_element(my_list, element)
if index != -1:
print(f"Element {element} found at index {index}")
else:
print(f"Element {element} is not in the list")
详细描述:自定义函数的优点在于灵活性,可以根据具体需求进行调整。适合用于特定场景。
总结
在Python中查找列表元素的方法有很多,每种方法都有其优点和适用场景。in
关键字适合快速检查,index()
适合需要知道位置时,遍历列表适合执行额外操作,filter()
和any()
适合复杂条件筛选,Counter
适合统计元素次数,numpy
和pandas
适合大数据处理,自定义函数适合特定需求。根据具体需求选择合适的方法,可以提高代码的效率和可读性。
相关问答FAQs:
在Python中,如何查找列表中的特定元素?
在Python中,可以使用in
关键字来检查一个元素是否在列表中。例如,如果你有一个列表my_list
,要查找元素x
是否存在于该列表中,可以使用if x in my_list:
。如果存在,程序会返回True
,否则返回False
。
Python提供了哪些方法来获取列表中元素的索引?
可以使用list.index(value)
方法来找到元素在列表中的第一个出现位置。如果元素不存在,Python会抛出一个ValueError
异常。为了避免这种情况,可以先使用in
关键字确认元素是否在列表中。
如何在列表中查找多个元素?
可以使用列表解析或循环遍历来查找多个元素。例如,可以编写一个列表理解来过滤出所有符合条件的元素。示例代码如下:found_items = [item for item in my_list if item in search_list]
,其中search_list
是你要查找的元素集合。这样的方式既高效又简洁,便于管理和维护。
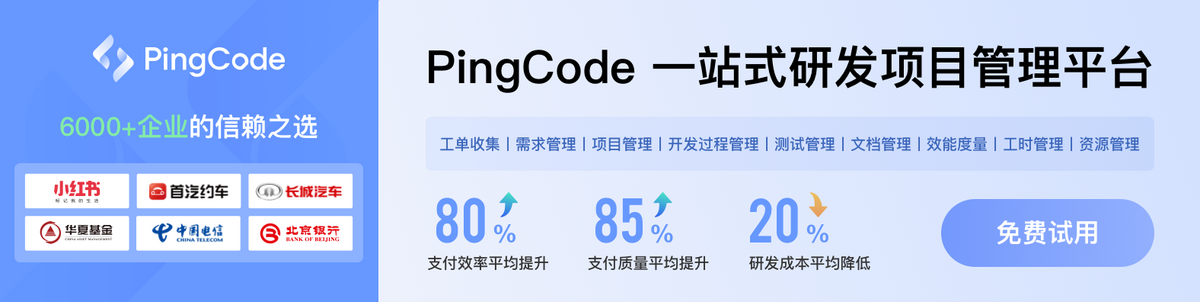