Python在文本中找到特定内容的方法有:字符串方法、正则表达式、使用内置库、使用第三方库。其中,最常用和强大的方法是使用正则表达式,因为它允许你定义复杂的搜索模式。
一、字符串方法
Python的字符串方法如find()
、index()
、count()
、startswith()
和endswith()
可以用于在文本中找到特定内容。虽然这些方法简单易用,但它们的功能有限,适用于比较简单的搜索任务。
使用 find()
方法
text = "Hello, welcome to the world of Python!"
position = text.find("Python")
if position != -1:
print(f"Found 'Python' at position {position}")
else:
print("Not found")
使用 count()
方法
text = "Hello, welcome to the world of Python! Python is great."
count = text.count("Python")
print(f"'Python' found {count} times")
二、正则表达式
正则表达式(regular expressions)是一个强大的工具,可以用来匹配复杂的文本模式。Python提供了re
模块来支持正则表达式。
使用 re.search()
查找
import re
text = "Hello, welcome to the world of Python!"
pattern = r"Python"
match = re.search(pattern, text)
if match:
print(f"Found '{match.group()}' at position {match.start()}")
else:
print("Not found")
使用 re.findall()
查找所有匹配
import re
text = "Hello, welcome to the world of Python! Python is great."
pattern = r"Python"
matches = re.findall(pattern, text)
print(f"Found matches: {matches}")
三、使用内置库
Python的内置库如string
、difflib
等也可以用于文本搜索。例如,difflib
库提供了方法来比较文本和找出相似度。
使用 difflib
库
import difflib
text = "Hello, welcome to the world of Python!"
pattern = "Python"
matches = difflib.get_close_matches(pattern, text.split(), n=1, cutoff=0.6)
print(f"Close matches: {matches}")
四、使用第三方库
有些第三方库如Whoosh
、Flashtext
等可以用于更复杂的搜索任务。Flashtext
库特别适用于搜索和替换大量关键词。
使用 Flashtext
库
from flashtext import KeywordProcessor
text = "Hello, welcome to the world of Python! Python is great."
keyword_processor = KeywordProcessor()
keyword_processor.add_keyword('Python')
matches = keyword_processor.extract_keywords(text)
print(f"Found matches: {matches}")
详细描述正则表达式的使用
正则表达式是一种用于描述字符模式的工具。通过正则表达式,我们可以方便地进行复杂的文本搜索、替换和解析任务。re
模块提供了丰富的功能来处理正则表达式,包括re.search()
、re.match()
、re.fullmatch()
、re.findall()
、re.finditer()
、re.sub()
等。
正则表达式基础语法
.
: 匹配任意一个字符(除了换行符)。^
: 匹配字符串的开头。$
: 匹配字符串的结尾。*
: 匹配前面的字符零次或多次。+
: 匹配前面的字符一次或多次。?
: 匹配前面的字符零次或一次。{n}
: 匹配前面的字符恰好n次。{n,}
: 匹配前面的字符至少n次。{n,m}
: 匹配前面的字符至少n次,至多m次。[abc]
: 匹配方括号中的任意一个字符。[^abc]
: 匹配不在方括号中的任意一个字符。|
: 匹配左右任意一个表达式。()
:捕获组,用于提取子字符串。
使用示例
- 匹配一个Email地址
import re
text = "Please contact us at support@example.com for assistance."
pattern = r'[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+'
matches = re.findall(pattern, text)
print(f"Found email addresses: {matches}")
- 替换文本中的日期格式
import re
text = "Today's date is 2023-11-01. Tomorrow's date is 2023-11-02."
pattern = r'(\d{4})-(\d{2})-(\d{2})'
replacement = r'\2/\3/\1'
new_text = re.sub(pattern, replacement, text)
print(f"Reformatted text: {new_text}")
正则表达式在处理复杂文本搜索任务时非常高效和灵活。掌握正则表达式的语法和使用技巧,可以大大提高文本处理的效率和准确性。
五、总结
在Python中找到特定内容的方法多种多样,从简单的字符串方法到强大的正则表达式,再到使用内置库和第三方库,每种方法都有其适用的场景和优缺点。对于简单的搜索任务,字符串方法足够使用;对于复杂的文本模式匹配,正则表达式无疑是最强大的工具;对于需要高效处理大量关键词的场景,第三方库如Flashtext
是不错的选择。掌握这些方法,能够使你在处理文本搜索任务时游刃有余。
相关问答FAQs:
如何使用Python查找文本中的特定字符串?
使用Python查找特定字符串可以通过内置的in
运算符或者字符串的find()
、index()
方法来实现。in
运算符非常简单,只需在字符串中直接检查特定内容是否存在。find()
和index()
方法可以返回特定字符串的索引位置,find()
会返回-1如果未找到,而index()
则会引发异常。
如何处理大小写敏感的搜索?
在进行字符串搜索时,大小写可能会影响结果。如果希望忽略大小写,可以将原始字符串和要查找的字符串都转换为小写或大写。例如,使用str.lower()
方法将两者都转化为小写,这样可以确保搜索不受大小写的限制。
在Python中如何查找多个特定内容?
如果需要查找多个特定字符串,可以使用循环或列表推导式结合in
运算符来处理。创建一个包含所有要查找字符串的列表,然后遍历该列表并检查每个元素是否存在于目标文本中。这样可以有效地处理多个搜索条件,提升搜索的灵活性。
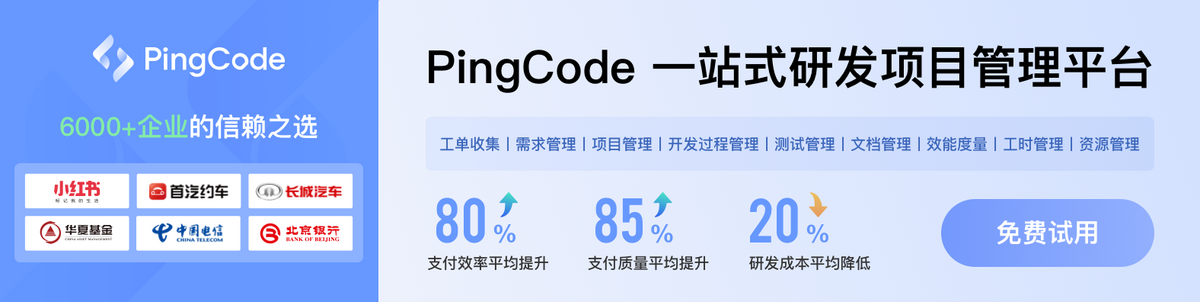