使用Python匹配字符串时,可以使用正则表达式、字符串方法、结合多种方法实现复杂匹配。 其中,正则表达式是一种强大的工具,它允许你指定复杂的模式来匹配字符串内容。re
模块提供了多种函数来实现正则表达式匹配,如re.search()
、re.match()
、re.findall()
等。接下来,我们将详细探讨如何使用这些方法来实现同时匹配多个条件。
一、正则表达式概述与基本使用
正则表达式(Regular Expressions)是一种用于匹配文本的模式。它允许你指定复杂的搜索条件。Python的re
模块提供了对正则表达式的支持。
1、导入re
模块
在使用正则表达式之前,首先需要导入re
模块:
import re
2、基本匹配函数
re.match(pattern, string)
:从字符串的起始位置匹配一个模式。re.search(pattern, string)
:搜索整个字符串,返回第一个匹配的对象。re.findall(pattern, string)
:搜索整个字符串,返回所有非重叠的匹配。
import re
text = "Python is a powerful programming language."
使用re.match()从字符串的起始位置匹配
match_result = re.match(r'Python', text)
if match_result:
print("Match:", match_result.group())
使用re.search()在整个字符串中搜索
search_result = re.search(r'powerful', text)
if search_result:
print("Search:", search_result.group())
使用re.findall()找到所有匹配项
findall_result = re.findall(r'language', text)
print("Findall:", findall_result)
二、同时匹配多个条件
要同时匹配多个条件,可以使用以下几种方法:
1、使用|
操作符
|
操作符表示“或”,可以用来匹配多个模式中的任意一个。
text = "Python is powerful and fast."
匹配“powerful”或“fast”
pattern = r'powerful|fast'
result = re.findall(pattern, text)
print("Result:", result)
2、使用捕获组
捕获组可以通过括号()
来指定,允许你在一个模式中匹配多个子模式。
text = "Python is powerful and fast."
捕获“powerful”和“fast”
pattern = r'(powerful|fast)'
result = re.findall(pattern, text)
print("Result:", result)
3、使用lookahead断言
lookahead断言(肯定前瞻断言)允许你指定在某个位置后必须存在的模式。
text = "Python is powerful and fast."
同时匹配“powerful”和“fast”
pattern = r'(?=.*powerful)(?=.*fast)'
result = re.search(pattern, text)
if result:
print("Both conditions are matched.")
三、字符串方法
除了正则表达式,Python的字符串方法也可以用于字符串匹配。这些方法包括str.find()
、str.startswith()
、str.endswith()
等。
1、str.find()
str.find()
方法返回子字符串在字符串中的最低索引,如果子字符串不在字符串中则返回-1。
text = "Python is powerful and fast."
if text.find("powerful") != -1 and text.find("fast") != -1:
print("Both 'powerful' and 'fast' are found.")
2、str.startswith()
和str.endswith()
这些方法分别用于检查字符串是否以指定子字符串开头或结尾。
text = "Python is powerful and fast."
if text.startswith("Python") and text.endswith("fast."):
print("The text starts with 'Python' and ends with 'fast.'")
四、结合多种方法实现复杂匹配
在实际使用中,可以结合正则表达式和字符串方法来实现更加复杂的匹配需求。
1、结合使用正则表达式和字符串方法
text = "Python is powerful and fast."
使用正则表达式匹配单词
words = re.findall(r'\b\w+\b', text)
检查是否同时包含多个指定单词
required_words = ["powerful", "fast"]
if all(word in words for word in required_words):
print("The text contains all required words.")
2、使用自定义函数实现复杂匹配
可以编写自定义函数来实现更复杂的匹配逻辑:
def match_conditions(text, conditions):
for condition in conditions:
if not re.search(condition, text):
return False
return True
text = "Python is powerful and fast."
conditions = [r'powerful', r'fast']
if match_conditions(text, conditions):
print("The text matches all conditions.")
五、性能优化
在处理大型文本数据时,需要考虑性能优化。可以使用以下方法来提高匹配效率:
1、预编译正则表达式
使用re.compile()
预编译正则表达式,可以提高匹配速度。
text = "Python is powerful and fast."
预编译正则表达式
pattern = re.compile(r'powerful|fast')
result = pattern.findall(text)
print("Result:", result)
2、合理选择匹配方法
根据具体需求选择合适的匹配方法。例如,对于简单的子字符串查找,可以使用str.find()
方法,而对于复杂模式匹配,使用正则表达式。
3、避免不必要的匹配
在处理大量数据时,尽量减少不必要的匹配操作。例如,可以先使用简单的字符串方法进行初步筛选,然后再使用正则表达式进行详细匹配。
def match_conditions(text, conditions):
if "powerful" not in text or "fast" not in text:
return False
for condition in conditions:
if not re.search(condition, text):
return False
return True
text = "Python is powerful and fast."
conditions = [r'powerful', r'fast']
if match_conditions(text, conditions):
print("The text matches all conditions.")
六、总结
Python提供了多种方法来实现字符串匹配,包括正则表达式和字符串方法。正则表达式是一种强大的工具,允许你指定复杂的匹配模式。通过结合使用正则表达式和字符串方法,可以实现复杂的匹配需求。为了提高匹配效率,可以使用预编译正则表达式和合理选择匹配方法。在处理大型文本数据时,尽量避免不必要的匹配操作。
希望通过本文的详细介绍,你能够更加熟练地使用Python进行字符串匹配,并能根据具体需求选择合适的方法。无论是简单的子字符串查找,还是复杂的模式匹配,Python都能提供强大的支持。
相关问答FAQs:
如何在Python中使用正则表达式同时匹配多个字符串?
在Python中,可以使用re
模块中的正则表达式来实现同时匹配多个字符串。通过使用|
运算符,可以将多个模式组合在一起。例如,re.search(r'模式1|模式2', 字符串)
可以同时匹配“模式1”和“模式2”。这使得在一个字符串中寻找多个潜在匹配变得简单而高效。
使用Python进行字符串匹配时,如何提高匹配效率?
为了提高字符串匹配的效率,可以考虑使用编译正则表达式的方式,例如,使用re.compile()
方法将模式编译为正则表达式对象。这样,在多次匹配时,可以避免每次调用时的编译开销。此外,选择合适的匹配模式和限制搜索范围,也能有效提高效率。
在Python中,如何处理大小写敏感的匹配?
在Python的正则表达式中,默认情况下匹配是大小写敏感的。如果需要进行不区分大小写的匹配,可以在调用匹配函数时添加re.IGNORECASE
参数。例如,re.search(r'模式', 字符串, re.IGNORECASE)
将忽略大小写差异,确保更广泛的匹配结果。这样可以确保在处理用户输入时,能够更好地匹配各种形式的字符串。
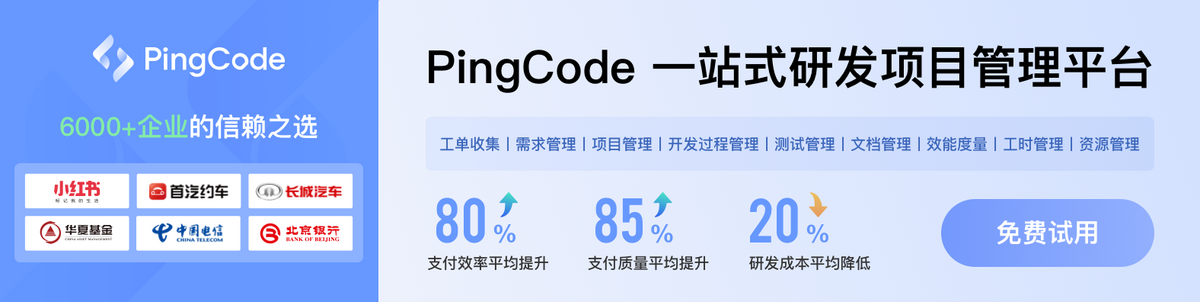