在Python中,生成一个矩阵tensor的方法有很多,主要依赖于TensorFlow和PyTorch等深度学习框架。你可以使用tf.constant
、tf.Variable
、tf.zeros
、tf.ones
等函数生成TensorFlow的张量;也可以使用torch.tensor
、torch.zeros
、torch.ones
等函数生成PyTorch的张量。
这里我们将详细描述如何在TensorFlow和PyTorch中生成一个矩阵张量,并且会深入探讨使用这些框架生成和操作张量的不同方法。
一、TensorFlow中的矩阵张量生成
TensorFlow是一个广泛使用的深度学习框架,提供了许多方法来生成和操作张量。以下是一些常用的方法:
1.1、使用 tf.constant
tf.constant
可以创建一个常量张量。你可以直接传递一个列表或数组来创建一个2D矩阵。
import tensorflow as tf
创建一个2x2的常量矩阵
matrix = tf.constant([[1, 2], [3, 4]], dtype=tf.int32)
print(matrix)
1.2、使用 tf.Variable
tf.Variable
创建一个可变张量。可变张量可以在训练过程中进行调整。
# 创建一个2x2的可变矩阵
matrix = tf.Variable([[1, 2], [3, 4]], dtype=tf.int32)
print(matrix)
1.3、使用 tf.zeros
和 tf.ones
你可以使用 tf.zeros
和 tf.ones
创建全零或全一的矩阵。
# 创建一个2x2的全零矩阵
zero_matrix = tf.zeros([2, 2], dtype=tf.float32)
print(zero_matrix)
创建一个2x2的全一矩阵
one_matrix = tf.ones([2, 2], dtype=tf.float32)
print(one_matrix)
1.4、使用 tf.eye
tf.eye
创建一个单位矩阵。
# 创建一个2x2的单位矩阵
identity_matrix = tf.eye(2, dtype=tf.float32)
print(identity_matrix)
1.5、使用 tf.random.normal
和 tf.random.uniform
你可以使用 tf.random.normal
和 tf.random.uniform
生成随机矩阵。
# 创建一个2x2的正态分布随机矩阵
normal_matrix = tf.random.normal([2, 2], mean=0.0, stddev=1.0)
print(normal_matrix)
创建一个2x2的均匀分布随机矩阵
uniform_matrix = tf.random.uniform([2, 2], minval=0, maxval=1)
print(uniform_matrix)
二、PyTorch中的矩阵张量生成
PyTorch是另一个流行的深度学习框架,也提供了丰富的张量生成和操作方法。以下是一些常用的方法:
2.1、使用 torch.tensor
torch.tensor
可以创建一个张量。你可以直接传递一个列表或数组来创建一个2D矩阵。
import torch
创建一个2x2的常量矩阵
matrix = torch.tensor([[1, 2], [3, 4]], dtype=torch.int32)
print(matrix)
2.2、使用 torch.zeros
和 torch.ones
你可以使用 torch.zeros
和 torch.ones
创建全零或全一的矩阵。
# 创建一个2x2的全零矩阵
zero_matrix = torch.zeros([2, 2], dtype=torch.float32)
print(zero_matrix)
创建一个2x2的全一矩阵
one_matrix = torch.ones([2, 2], dtype=torch.float32)
print(one_matrix)
2.3、使用 torch.eye
torch.eye
创建一个单位矩阵。
# 创建一个2x2的单位矩阵
identity_matrix = torch.eye(2, dtype=torch.float32)
print(identity_matrix)
2.4、使用 torch.randn
和 torch.rand
你可以使用 torch.randn
和 torch.rand
生成随机矩阵。
# 创建一个2x2的正态分布随机矩阵
normal_matrix = torch.randn([2, 2])
print(normal_matrix)
创建一个2x2的均匀分布随机矩阵
uniform_matrix = torch.rand([2, 2])
print(uniform_matrix)
三、总结
在TensorFlow和PyTorch中生成一个矩阵张量的方法非常多样,包括使用常量、变量、全零、全一、单位矩阵以及随机生成的矩阵。这些方法各有优劣,可以根据具体需求选择合适的方法。例如,使用tf.constant
和torch.tensor
可以创建常量矩阵,适用于无需改变的参数;使用tf.Variable
则可以创建可变矩阵,适用于需要在训练过程中更新的参数。此外,使用随机生成的方法可以初始化参数,有助于模型训练的收敛。
希望这篇文章能够帮助你更好地理解如何在Python中生成一个矩阵张量,并为你的深度学习项目提供有力的支持。
相关问答FAQs:
如何在Python中创建一个矩阵tensor?
在Python中,使用NumPy库可以轻松创建一个矩阵tensor。可以通过使用numpy.array()
函数来定义一个二维数组,从而生成一个矩阵tensor。例如:
import numpy as np
matrix_tensor = np.array([[1, 2], [3, 4]])
print(matrix_tensor)
上述代码将生成一个2×2的矩阵tensor。
可以使用哪些库来生成矩阵tensor?
除了NumPy,TensorFlow和PyTorch也是流行的库,用于生成和操作矩阵tensor。TensorFlow使用tf.constant()
或tf.Variable()
函数创建tensor,而PyTorch则使用torch.tensor()
函数。例如:
import tensorflow as tf
tensor_tf = tf.constant([[1, 2], [3, 4]])
print(tensor_tf)
import torch
tensor_pt = torch.tensor([[1, 2], [3, 4]])
print(tensor_pt)
如何生成随机矩阵tensor?
生成随机矩阵tensor可以使用NumPy或其他库中的随机函数。使用NumPy的numpy.random.rand()
可以生成一个包含随机数的矩阵tensor。以下是一个例子:
random_tensor = np.random.rand(3, 3) # 创建一个3x3的随机矩阵
print(random_tensor)
在TensorFlow中,使用tf.random.uniform()
可以生成随机tensor,语法如下:
random_tensor_tf = tf.random.uniform(shape=(3, 3), minval=0, maxval=1)
print(random_tensor_tf)
通过这些方法,可以根据需求轻松生成各种矩阵tensor。
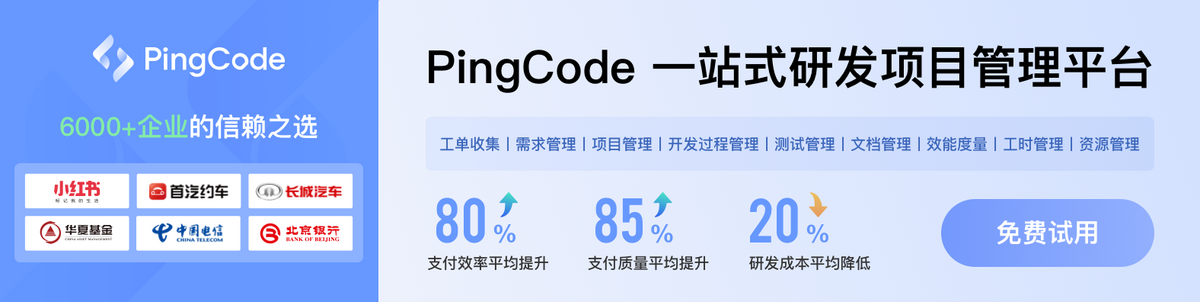