删除文件及文件夹的主要方法有:os.remove()、os.rmdir()、shutil.rmtree()。其中,os.remove()用于删除单个文件,os.rmdir()用于删除单个空文件夹,shutil.rmtree()用于删除文件夹及其所有内容。下面将详细介绍这几种方法,并提供具体的代码示例。
一、os.remove()删除文件
使用os.remove()可以删除指定路径的单个文件。这个方法不会删除文件夹。如果指定的路径是一个文件夹,会抛出一个错误。
import os
文件路径
file_path = 'path/to/your/file.txt'
删除文件
os.remove(file_path)
print(f"{file_path} has been deleted.")
注意: 在删除文件之前,最好先检查文件是否存在,否则会抛出FileNotFoundError。
if os.path.exists(file_path):
os.remove(file_path)
print(f"{file_path} has been deleted.")
else:
print(f"{file_path} does not exist.")
二、os.rmdir()删除空文件夹
os.rmdir()只能删除空文件夹,如果文件夹不为空,会抛出OSError。
import os
文件夹路径
dir_path = 'path/to/your/directory'
删除空文件夹
os.rmdir(dir_path)
print(f"{dir_path} has been deleted.")
注意: 在删除文件夹之前,最好先检查文件夹是否存在。
if os.path.exists(dir_path):
os.rmdir(dir_path)
print(f"{dir_path} has been deleted.")
else:
print(f"{dir_path} does not exist.")
三、shutil.rmtree()删除文件夹及其所有内容
如果需要删除非空文件夹及其所有内容,可以使用shutil.rmtree()。
import shutil
文件夹路径
dir_path = 'path/to/your/directory'
删除文件夹及其所有内容
shutil.rmtree(dir_path)
print(f"{dir_path} and all its content have been deleted.")
注意: 在删除文件夹及其内容之前,最好先检查文件夹是否存在。
if os.path.exists(dir_path):
shutil.rmtree(dir_path)
print(f"{dir_path} and all its content have been deleted.")
else:
print(f"{dir_path} does not exist.")
四、异常处理
在删除文件和文件夹时,可能会遇到各种异常情况,如文件或文件夹不存在、没有权限删除等。可以使用try-except块来处理这些异常。
import os
import shutil
def delete_file(file_path):
try:
if os.path.exists(file_path):
os.remove(file_path)
print(f"{file_path} has been deleted.")
else:
print(f"{file_path} does not exist.")
except Exception as e:
print(f"Error occurred while deleting file: {e}")
def delete_directory(dir_path):
try:
if os.path.exists(dir_path):
shutil.rmtree(dir_path)
print(f"{dir_path} and all its content have been deleted.")
else:
print(f"{dir_path} does not exist.")
except Exception as e:
print(f"Error occurred while deleting directory: {e}")
使用示例
delete_file('path/to/your/file.txt')
delete_directory('path/to/your/directory')
五、用户确认删除操作
为了避免误删除重要文件或文件夹,可以在删除操作之前添加用户确认步骤。
import os
import shutil
def user_confirm(prompt):
while True:
response = input(prompt).lower()
if response in ['y', 'n']:
return response == 'y'
else:
print("Please respond with 'y' or 'n'.")
def delete_file(file_path):
if user_confirm(f"Are you sure you want to delete {file_path}? (y/n): "):
try:
if os.path.exists(file_path):
os.remove(file_path)
print(f"{file_path} has been deleted.")
else:
print(f"{file_path} does not exist.")
except Exception as e:
print(f"Error occurred while deleting file: {e}")
def delete_directory(dir_path):
if user_confirm(f"Are you sure you want to delete {dir_path} and all its content? (y/n): "):
try:
if os.path.exists(dir_path):
shutil.rmtree(dir_path)
print(f"{dir_path} and all its content have been deleted.")
else:
print(f"{dir_path} does not exist.")
except Exception as e:
print(f"Error occurred while deleting directory: {e}")
使用示例
delete_file('path/to/your/file.txt')
delete_directory('path/to/your/directory')
六、日志记录删除操作
为了记录删除操作,可以将删除操作记录到日志文件中。
import os
import shutil
import logging
配置日志记录
logging.basicConfig(filename='file_operations.log', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
def delete_file(file_path):
try:
if os.path.exists(file_path):
os.remove(file_path)
logging.info(f"{file_path} has been deleted.")
else:
logging.warning(f"{file_path} does not exist.")
except Exception as e:
logging.error(f"Error occurred while deleting file: {e}")
def delete_directory(dir_path):
try:
if os.path.exists(dir_path):
shutil.rmtree(dir_path)
logging.info(f"{dir_path} and all its content have been deleted.")
else:
logging.warning(f"{dir_path} does not exist.")
except Exception as e:
logging.error(f"Error occurred while deleting directory: {e}")
使用示例
delete_file('path/to/your/file.txt')
delete_directory('path/to/your/directory')
通过以上方法,您可以有效地删除文件和文件夹,并在删除操作中进行异常处理、用户确认和日志记录。这些措施可以帮助您避免误删除重要文件或文件夹,并在操作出现错误时进行有效处理。
相关问答FAQs:
如何在Python中安全地删除文件?
在Python中,您可以使用os
模块中的remove()
函数安全地删除文件。首先,确保您有文件的正确路径,并且在删除之前检查文件是否存在。可以使用os.path.exists()
来确认文件的存在性,以避免因尝试删除不存在的文件而引发错误。
如何使用Python删除整个文件夹?
删除整个文件夹可以使用shutil
模块中的rmtree()
函数。这个函数会递归地删除指定路径下的所有文件和子文件夹。在使用此功能之前,建议备份文件夹内容,因为这个操作是不可逆的。
使用Python删除空文件夹的最佳方法是什么?
对于空文件夹,可以使用os
模块中的rmdir()
函数。该函数仅在文件夹为空的情况下有效。如果您尝试删除一个非空文件夹,将会引发OSError
。在删除之前,确保文件夹确实为空,以避免错误。
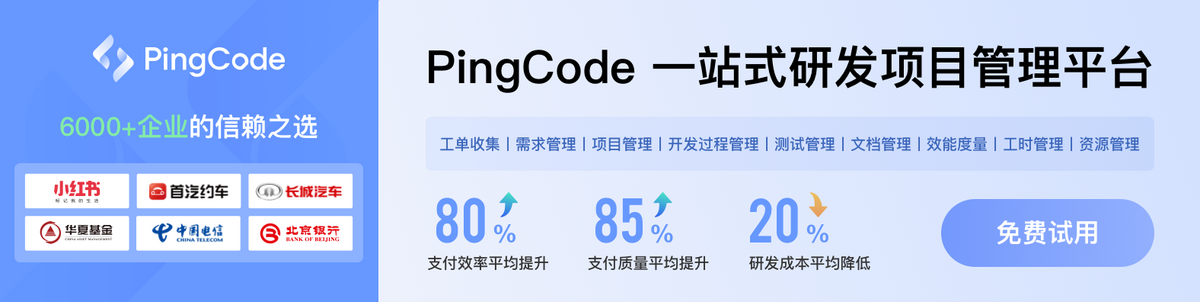