用Python编写一场烟花
用Python编写一场烟花效果,可以通过图形界面库和物理模拟库实现。使用Pygame库、使用Turtle图形库、使用Matplotlib动画。其中,使用Pygame库是比较推荐的方法,因为它提供了丰富的图形和动画功能,可以实现更复杂和逼真的效果。接下来,我们将详细介绍如何使用Pygame库编写一场烟花效果。
一、安装和导入Pygame库
首先,我们需要安装Pygame库。如果你还没有安装Pygame,可以使用以下命令来安装:
pip install pygame
安装完成后,我们在代码中导入Pygame库:
import pygame
import random
import math
二、初始化Pygame和设置窗口
在编写烟花效果之前,我们需要初始化Pygame,并设置一个窗口来显示烟花效果:
# 初始化Pygame
pygame.init()
设置窗口的大小
window_size = (800, 600)
screen = pygame.display.set_mode(window_size)
pygame.display.set_caption("Fireworks Display")
设置帧率
clock = pygame.time.Clock()
FPS = 60
三、定义烟花粒子类
为了实现烟花效果,我们需要定义一个粒子类,每个粒子代表烟花的一部分。粒子类需要包含位置、速度、颜色和寿命等属性:
class Particle:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
self.radius = random.randint(2, 4)
self.lifetime = random.randint(50, 100)
self.angle = random.uniform(0, 2 * math.pi)
self.speed = random.uniform(2, 5)
self.vx = self.speed * math.cos(self.angle)
self.vy = self.speed * math.sin(self.angle)
def update(self):
self.x += self.vx
self.y += self.vy
self.vy += 0.05 # 模拟重力
self.lifetime -= 1
def draw(self, screen):
if self.lifetime > 0:
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.radius)
四、生成烟花
为了生成烟花,我们可以定义一个函数,每次调用该函数时生成一组粒子,并将它们添加到一个粒子列表中:
def create_firework():
x = random.randint(100, 700)
y = random.randint(100, 500)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
particles = [Particle(x, y, color) for _ in range(100)]
return particles
五、主循环
在主循环中,我们需要处理事件、更新和绘制粒子,并控制帧率:
particles = []
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 每隔一段时间生成新的烟花
if random.random() < 0.05:
particles.extend(create_firework())
screen.fill((0, 0, 0))
for particle in particles[:]:
particle.update()
particle.draw(screen)
if particle.lifetime <= 0:
particles.remove(particle)
pygame.display.flip()
clock.tick(FPS)
pygame.quit()
六、完整代码
import pygame
import random
import math
初始化Pygame
pygame.init()
设置窗口的大小
window_size = (800, 600)
screen = pygame.display.set_mode(window_size)
pygame.display.set_caption("Fireworks Display")
设置帧率
clock = pygame.time.Clock()
FPS = 60
class Particle:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
self.radius = random.randint(2, 4)
self.lifetime = random.randint(50, 100)
self.angle = random.uniform(0, 2 * math.pi)
self.speed = random.uniform(2, 5)
self.vx = self.speed * math.cos(self.angle)
self.vy = self.speed * math.sin(self.angle)
def update(self):
self.x += self.vx
self.y += self.vy
self.vy += 0.05 # 模拟重力
self.lifetime -= 1
def draw(self, screen):
if self.lifetime > 0:
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.radius)
def create_firework():
x = random.randint(100, 700)
y = random.randint(100, 500)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
particles = [Particle(x, y, color) for _ in range(100)]
return particles
particles = []
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 每隔一段时间生成新的烟花
if random.random() < 0.05:
particles.extend(create_firework())
screen.fill((0, 0, 0))
for particle in particles[:]:
particle.update()
particle.draw(screen)
if particle.lifetime <= 0:
particles.remove(particle)
pygame.display.flip()
clock.tick(FPS)
pygame.quit()
七、解释与扩展
在这段代码中,我们通过定义粒子类和生成烟花的函数,实现了一个简单的烟花效果。我们可以通过调整粒子的属性和行为,进一步增强烟花效果。例如:
- 颜色渐变:在粒子的生命周期内,逐渐改变它的颜色,使其在消失前变暗或改变颜色。
- 轨迹效果:在粒子移动时,绘制一条渐隐的轨迹,模拟烟花在空中留下的痕迹。
- 多种烟花形状:通过调整粒子的初始速度和角度,生成不同形状的烟花,如环形、星形等。
扩展一、颜色渐变
我们可以在粒子的 update
方法中添加颜色渐变的效果,使粒子在消失前逐渐变暗:
def update(self):
self.x += self.vx
self.y += self.vy
self.vy += 0.05 # 模拟重力
self.lifetime -= 1
# 颜色渐变
self.color = (
max(0, self.color[0] - 2),
max(0, self.color[1] - 2),
max(0, self.color[2] - 2)
)
扩展二、轨迹效果
我们可以在粒子的 draw
方法中绘制一条渐隐的轨迹:
def draw(self, screen):
if self.lifetime > 0:
trail_length = 5
for i in range(trail_length):
alpha = int(255 * (1 - i / trail_length))
color = (self.color[0], self.color[1], self.color[2], alpha)
pos = (int(self.x - self.vx * i), int(self.y - self.vy * i))
pygame.draw.circle(screen, color, pos, self.radius)
扩展三、多种烟花形状
通过调整粒子的初始速度和角度,我们可以生成不同形状的烟花。例如,生成星形烟花:
def create_firework():
x = random.randint(100, 700)
y = random.randint(100, 500)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
particles = []
for _ in range(100):
angle = random.uniform(0, 2 * math.pi)
speed = random.uniform(2, 5)
if random.random() < 0.5:
angle += math.pi / 4 # 星形效果
vx = speed * math.cos(angle)
vy = speed * math.sin(angle)
particles.append(Particle(x, y, color))
return particles
通过这些扩展和调整,我们可以实现更丰富和逼真的烟花效果。希望这篇文章能帮助你掌握如何用Python编写一场烟花,并激发你进行更多的创意和尝试。
相关问答FAQs:
如何在Python中创建烟花动画?
创建烟花动画的基本步骤包括使用Python的图形库,如Pygame或Turtle。首先,您需要安装相应的库,然后编写代码来定义烟花的发射、爆炸以及颜色变化等特效。可以通过设置随机位置和颜色来使烟花效果更加生动。
哪些Python库适合制作烟花效果?
常见的Python库有Pygame和Turtle。Pygame适合制作复杂的动画和游戏效果,提供了丰富的功能来处理图形、声音和输入。而Turtle则更简单,适合初学者进行基础的图形绘制和动画制作。
如何为烟花动画添加音效?
在Pygame中,可以使用内置的音频功能来添加音效。您需要准备音效文件(如WAV或MP3格式),在代码中加载这些文件,并在烟花爆炸时播放相应的声音效果。确保音效与视觉效果同步,以增强整体体验。
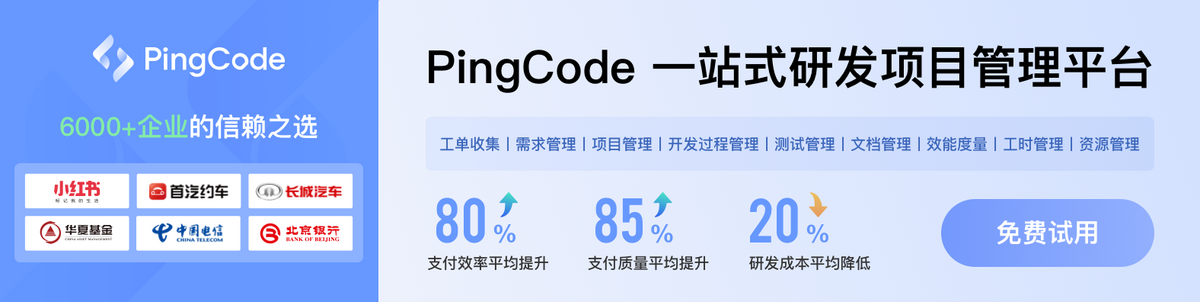