在Python中读取文本文件并指定文件路径是一项非常基础但又非常重要的操作。你可以使用绝对路径、相对路径、使用os.path
模块来处理路径、以及使用with
语句来确保文件被正确地打开和关闭。下面将详细描述这些方法中的一种。
推荐的方法是使用os.path
模块来处理路径。因为不同操作系统的文件路径分隔符不同,所以使用os.path
模块可以使你的代码更加跨平台。
import os
获取当前文件的目录
current_directory = os.path.dirname(os.path.abspath(__file__))
拼接文件路径
file_path = os.path.join(current_directory, 'your_file.txt')
读取文件
with open(file_path, 'r') as file:
content = file.read()
print(content)
一、绝对路径和相对路径
1、绝对路径
绝对路径是指从根目录开始的完整路径。这样可以确保文件路径在任何地方都有效,但它的缺点是缺乏灵活性。
# 使用绝对路径
file_path = 'C:/Users/YourUsername/Documents/your_file.txt'
with open(file_path, 'r') as file:
content = file.read()
print(content)
2、相对路径
相对路径是指相对于当前工作目录的路径。它的优点是更加灵活,但在不同的运行环境下可能会有所不同。
# 使用相对路径
file_path = 'your_file.txt'
with open(file_path, 'r') as file:
content = file.read()
print(content)
二、使用os.path
模块
os.path
模块提供了一些有用的方法来操作路径,使代码更加跨平台。
import os
获取当前工作目录
current_directory = os.getcwd()
拼接文件路径
file_path = os.path.join(current_directory, 'your_file.txt')
with open(file_path, 'r') as file:
content = file.read()
print(content)
三、确保文件被正确打开和关闭
在Python中,使用with
语句来处理文件操作是一种最佳实践。这样可以确保文件被正确地打开和关闭,即使在发生异常的情况下。
file_path = 'your_file.txt'
使用 with 语句
with open(file_path, 'r') as file:
content = file.read()
print(content)
四、处理文件路径中的特殊字符
有时文件路径中可能包含空格或其他特殊字符,这时候可以使用原始字符串(r
)来处理。
# 文件路径中包含空格
file_path = r'C:\Users\Your Username\Documents\your_file.txt'
with open(file_path, 'r') as file:
content = file.read()
print(content)
五、处理不同操作系统的文件路径
不同操作系统的文件路径分隔符不同,Windows使用反斜杠(),而Linux和macOS使用正斜杠(
/
)。使用os.path
模块可以帮助你处理这一问题。
import os
获取当前工作目录
current_directory = os.path.dirname(os.path.abspath(__file__))
拼接文件路径
file_path = os.path.join(current_directory, 'your_file.txt')
with open(file_path, 'r') as file:
content = file.read()
print(content)
六、读取大文件
如果你需要读取一个非常大的文件,可以考虑逐行读取文件,而不是一次性将整个文件内容读入内存。
file_path = 'your_file.txt'
with open(file_path, 'r') as file:
for line in file:
print(line.strip())
七、处理不同编码的文件
有时你需要读取不同编码的文件,比如UTF-8、GBK等。在这种情况下,你可以在open
函数中指定编码。
file_path = 'your_file.txt'
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
八、异常处理
在文件操作中,异常处理也是非常重要的。你可以使用try-except
块来处理可能出现的异常。
file_path = 'your_file.txt'
try:
with open(file_path, 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print(f"The file at path {file_path} was not found.")
except IOError:
print(f"An I/O error occurred while accessing {file_path}.")
九、写入文件
除了读取文件,有时你也需要写入文件。你可以使用open
函数的'w'
模式来写入文件。
file_path = 'your_file.txt'
with open(file_path, 'w') as file:
file.write("Hello, World!")
如果你想追加内容到文件,可以使用'a'
模式。
file_path = 'your_file.txt'
with open(file_path, 'a') as file:
file.write("\nHello again!")
十、示例代码总结
以下是一个完整的示例代码,展示了如何使用os.path
模块来读取和写入文件,并处理可能的异常。
import os
获取当前工作目录
current_directory = os.path.dirname(os.path.abspath(__file__))
拼接文件路径
file_path = os.path.join(current_directory, 'your_file.txt')
尝试读取文件
try:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
except FileNotFoundError:
print(f"The file at path {file_path} was not found.")
except IOError:
print(f"An I/O error occurred while accessing {file_path}.")
尝试写入文件
try:
with open(file_path, 'w', encoding='utf-8') as file:
file.write("Hello, World!")
except IOError:
print(f"An I/O error occurred while writing to {file_path}.")
通过以上方法和示例代码,你可以更好地理解和处理Python中的文件路径操作。无论是绝对路径还是相对路径,使用os.path
模块处理路径都是一种更好的选择,可以使你的代码更加健壮和跨平台。在实际开发中,根据具体需求选择合适的方法来处理文件路径和文件操作。
相关问答FAQs:
如何在Python中指定txt文件的路径?
在Python中,您可以使用绝对路径或相对路径来指定txt文件的路径。绝对路径是从系统根目录开始的完整路径,而相对路径则是相对于当前工作目录的路径。确保在路径中使用正确的斜杠(Windows使用反斜杠\,而Linux和macOS使用正斜杠/),并考虑使用原始字符串(在字符串前加r)来避免转义字符的问题。
如果我的txt文件在不同的文件夹中,我该如何读取?
要读取位于不同文件夹中的txt文件,您需要在打开文件时提供正确的路径。例如,如果txt文件位于“D:\documents\example.txt”,您可以使用open(r'D:\documents\example.txt', 'r')
来打开文件。确保路径中没有拼写错误,并且文件确实存在于指定位置。
在读取txt文件时,如何处理文件路径错误?
在读取txt文件时,如果指定的路径错误,Python会抛出FileNotFoundError
。您可以使用try-except
语句来捕获这个错误,以便提供友好的错误消息或进行其他处理。例如:
try:
with open('your_file_path.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("指定的文件路径不存在,请检查路径是否正确。")
这样可以有效地防止程序崩溃,并帮助用户了解问题所在。
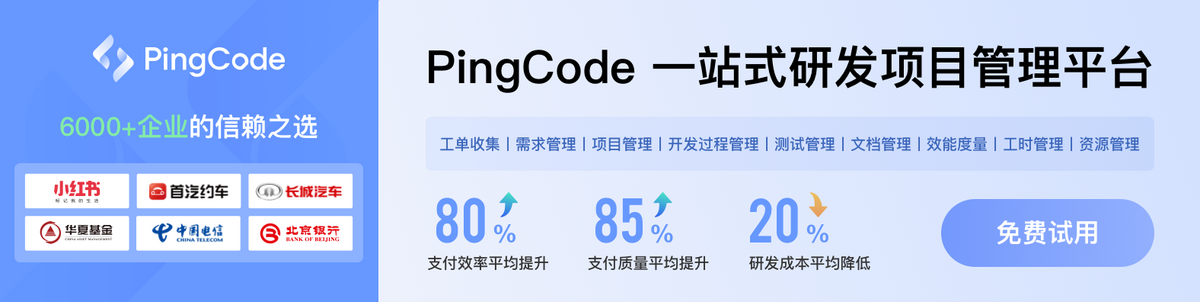