在Python中,可以使用tkinter
库、PyQt
库、wxPython
库等在窗体上显示结果,推荐使用tkinter
库,因为它是Python标准库的一部分、易于学习且功能强大。 使用tkinter
库创建窗体并显示结果的步骤包括:1. 导入tkinter
库;2. 创建主窗口;3. 创建组件(如标签、按钮等);4. 将组件添加到窗口;5. 显示窗口。下面将详细介绍如何使用tkinter
库在窗体上显示结果。
一、导入tkinter
库
首先需要导入tkinter
库,以便在Python程序中使用其功能。可以使用以下代码导入tkinter
库:
import tkinter as tk
导入后,可以使用tk
作为前缀来引用tkinter
库中的类和函数。
二、创建主窗口
创建主窗口是所有tkinter
程序的第一步。可以使用Tk()
函数创建主窗口,并将其赋值给一个变量,例如root
。然后,可以使用mainloop()
方法启动主窗口的事件循环,使其保持运行状态,直到用户关闭窗口。以下是创建主窗口的示例代码:
root = tk.Tk()
root.title("My Application")
root.geometry("400x300")
三、创建组件
在tkinter
中,可以使用各种组件(如标签、按钮、文本框等)来与用户进行交互。要在窗体上显示结果,可以使用Label
组件。以下是创建一个标签组件的示例代码:
label = tk.Label(root, text="Hello, World!")
四、将组件添加到窗口
创建组件后,需要将其添加到主窗口中。可以使用pack()
、grid()
或place()
方法来进行布局。以下是将标签组件添加到主窗口的示例代码:
label.pack()
五、显示窗口
最后,启动主窗口的事件循环,使其保持运行状态,直到用户关闭窗口。以下是完整的示例代码:
import tkinter as tk
def show_result():
result = "Hello, World!"
label.config(text=result)
root = tk.Tk()
root.title("My Application")
root.geometry("400x300")
label = tk.Label(root, text="Click the button to see the result")
label.pack(pady=20)
button = tk.Button(root, text="Show Result", command=show_result)
button.pack(pady=20)
root.mainloop()
以上代码创建了一个tkinter
窗口,并在窗体上显示一个标签和一个按钮。当用户点击按钮时,标签上的文本会更改为结果。
六、进一步优化和扩展
接下来,我们可以进一步优化和扩展上述代码,使其更加实用和专业。
1、添加输入框
可以添加一个输入框(Entry
组件),让用户输入一些数据,并在窗体上显示结果。例如:
import tkinter as tk
def show_result():
user_input = entry.get()
result = f"Hello, {user_input}!"
label.config(text=result)
root = tk.Tk()
root.title("My Application")
root.geometry("400x300")
label = tk.Label(root, text="Enter your name and click the button:")
label.pack(pady=20)
entry = tk.Entry(root)
entry.pack(pady=20)
button = tk.Button(root, text="Show Result", command=show_result)
button.pack(pady=20)
root.mainloop()
2、添加更多组件
可以添加更多组件,例如多行文本框(Text
组件)、复选框(Checkbutton
组件)、单选按钮(Radiobutton
组件)等,以增强用户交互。例如:
import tkinter as tk
def show_result():
user_input = entry.get()
result = f"Hello, {user_input}!"
if var.get():
result += " You have agreed to the terms."
label.config(text=result)
root = tk.Tk()
root.title("My Application")
root.geometry("400x300")
label = tk.Label(root, text="Enter your name and click the button:")
label.pack(pady=20)
entry = tk.Entry(root)
entry.pack(pady=20)
var = tk.BooleanVar()
checkbutton = tk.Checkbutton(root, text="I agree to the terms", variable=var)
checkbutton.pack(pady=20)
button = tk.Button(root, text="Show Result", command=show_result)
button.pack(pady=20)
root.mainloop()
3、使用grid
布局管理器
可以使用grid
布局管理器代替pack
布局管理器,以便更精确地控制组件的布局。例如:
import tkinter as tk
def show_result():
user_input = entry.get()
result = f"Hello, {user_input}!"
if var.get():
result += " You have agreed to the terms."
label.config(text=result)
root = tk.Tk()
root.title("My Application")
root.geometry("400x300")
label = tk.Label(root, text="Enter your name and click the button:")
label.grid(row=0, column=0, columnspan=2, pady=20)
entry = tk.Entry(root)
entry.grid(row=1, column=0, columnspan=2, pady=20)
var = tk.BooleanVar()
checkbutton = tk.Checkbutton(root, text="I agree to the terms", variable=var)
checkbutton.grid(row=2, column=0, columnspan=2, pady=20)
button = tk.Button(root, text="Show Result", command=show_result)
button.grid(row=3, column=0, columnspan=2, pady=20)
root.mainloop()
4、使用place
布局管理器
可以使用place
布局管理器以绝对位置或相对位置放置组件。例如:
import tkinter as tk
def show_result():
user_input = entry.get()
result = f"Hello, {user_input}!"
if var.get():
result += " You have agreed to the terms."
label.config(text=result)
root = tk.Tk()
root.title("My Application")
root.geometry("400x300")
label = tk.Label(root, text="Enter your name and click the button:")
label.place(x=50, y=50)
entry = tk.Entry(root)
entry.place(x=50, y=100)
var = tk.BooleanVar()
checkbutton = tk.Checkbutton(root, text="I agree to the terms", variable=var)
checkbutton.place(x=50, y=150)
button = tk.Button(root, text="Show Result", command=show_result)
button.place(x=50, y=200)
root.mainloop()
5、使用tkinter
主题
ttk
模块提供了更现代的tkinter
小部件,可以使应用程序看起来更专业。例如:
import tkinter as tk
from tkinter import ttk
def show_result():
user_input = entry.get()
result = f"Hello, {user_input}!"
if var.get():
result += " You have agreed to the terms."
label.config(text=result)
root = tk.Tk()
root.title("My Application")
root.geometry("400x300")
label = ttk.Label(root, text="Enter your name and click the button:")
label.grid(row=0, column=0, columnspan=2, pady=20)
entry = ttk.Entry(root)
entry.grid(row=1, column=0, columnspan=2, pady=20)
var = tk.BooleanVar()
checkbutton = ttk.Checkbutton(root, text="I agree to the terms", variable=var)
checkbutton.grid(row=2, column=0, columnspan=2, pady=20)
button = ttk.Button(root, text="Show Result", command=show_result)
button.grid(row=3, column=0, columnspan=2, pady=20)
root.mainloop()
使用ttk
模块,您可以获得现代化的外观和感觉,并且可以更轻松地应用主题。
总结
以上内容详细介绍了如何使用tkinter
库在Python窗体上显示结果。通过导入tkinter
库、创建主窗口、创建组件、将组件添加到窗口、显示窗口等步骤,可以轻松实现窗体显示结果的功能。同时,通过添加输入框、更多组件、使用不同的布局管理器和ttk
主题,可以进一步优化和扩展您的应用程序,使其更加实用和专业。
相关问答FAQs:
如何在Python窗体中显示计算结果?
在Python中,可以使用Tkinter库创建窗体应用程序。通过设置标签(Label)或文本框(Text)组件,可以轻松地在窗体上显示计算结果。首先,您需要导入Tkinter库,创建一个主窗口,并在窗口中添加相应的组件,以展示您的计算结果。
使用Tkinter创建窗体需要哪些基本步骤?
创建Tkinter窗体的基本步骤包括导入Tkinter库,实例化Tk类,设置窗口标题和大小,创建并布局组件(如按钮、标签、文本框),以及使用mainloop()方法启动事件循环。通过这些步骤,您可以构建一个简单的用户界面来显示结果。
如何处理用户输入并在窗体中实时更新结果?
可以通过绑定事件处理函数来处理用户输入。在Tkinter中,可以使用Entry组件让用户输入数据,并通过按钮的命令属性触发计算。当用户点击按钮时,可以获取Entry中的值,执行计算,并将结果更新到Label或Text组件中,从而实现实时更新效果。
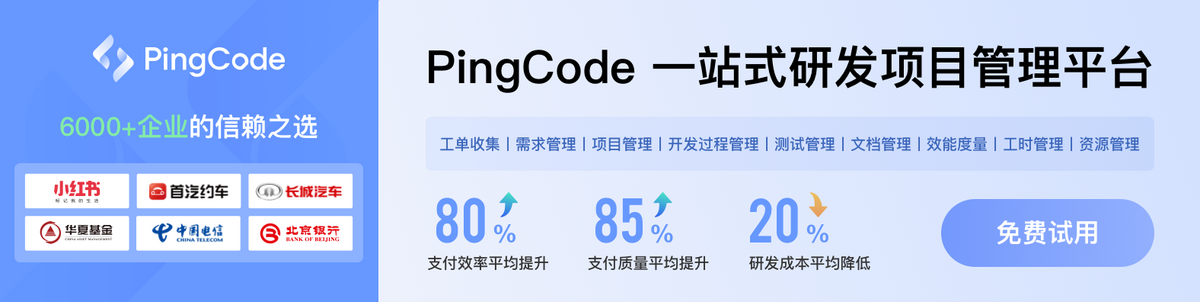