编写 Python 打印价格列表程序的方法有很多种,使用数据结构、格式化输出、循环等方法来完成。首先,你需要定义商品及其价格,然后通过格式化输出将它们打印到控制台。下面将详细描述如何使用Python编写一个打印价格列表的程序。
一、定义商品及其价格
首先需要定义商品及其价格。可以使用字典来存储商品名称和价格,这样可以方便地进行查找和操作。
# 定义商品及其价格
products = {
'Apple': 1.2,
'Banana': 0.5,
'Cherry': 2.5,
'Date': 3.0,
'Elderberry': 1.5
}
二、格式化输出
为了使输出更加整洁美观,使用字符串格式化方法来打印价格列表。可以使用str.format()
方法或f-string(Python 3.6及以上版本)来实现。
# 使用 str.format() 方法
for product, price in products.items():
print("{:<10} : ${:>5.2f}".format(product, price))
使用 f-string 方法
for product, price in products.items():
print(f"{product:<10} : ${price:>5.2f}")
三、添加头部和尾部
可以添加表头和表尾来增强可读性。
# 打印表头
print("{:<10} : {}".format("Product", "Price"))
print("-" * 20)
打印商品及其价格
for product, price in products.items():
print(f"{product:<10} : ${price:>5.2f}")
打印表尾
print("-" * 20)
四、计算总价格
如果需要计算并打印所有商品的总价格,可以在循环中累加价格。
# 计算总价格
total_price = 0.0
打印商品及其价格
for product, price in products.items():
print(f"{product:<10} : ${price:>5.2f}")
total_price += price
打印总价格
print("-" * 20)
print(f"{'Total':<10} : ${total_price:>5.2f}")
五、添加用户交互
可以添加用户输入功能来动态添加商品及其价格。
# 定义商品及其价格
products = {}
获取用户输入
while True:
product = input("Enter product name (or 'done' to finish): ")
if product.lower() == 'done':
break
price = float(input(f"Enter price for {product}: "))
products[product] = price
打印表头
print("{:<10} : {}".format("Product", "Price"))
print("-" * 20)
计算总价格
total_price = 0.0
打印商品及其价格
for product, price in products.items():
print(f"{product:<10} : ${price:>5.2f}")
total_price += price
打印总价格
print("-" * 20)
print(f"{'Total':<10} : ${total_price:>5.2f}")
六、处理异常和数据验证
在实际应用中,用户输入可能包含错误数据,因此需要添加异常处理和数据验证功能。
# 定义商品及其价格
products = {}
获取用户输入
while True:
product = input("Enter product name (or 'done' to finish): ")
if product.lower() == 'done':
break
try:
price = float(input(f"Enter price for {product}: "))
if price < 0:
raise ValueError("Price cannot be negative")
products[product] = price
except ValueError as e:
print(f"Invalid input: {e}")
打印表头
print("{:<10} : {}".format("Product", "Price"))
print("-" * 20)
计算总价格
total_price = 0.0
打印商品及其价格
for product, price in products.items():
print(f"{product:<10} : ${price:>5.2f}")
total_price += price
打印总价格
print("-" * 20)
print(f"{'Total':<10} : ${total_price:>5.2f}")
七、将数据写入文件
可以将商品及其价格写入文件,以便后续读取和处理。
# 定义商品及其价格
products = {}
获取用户输入
while True:
product = input("Enter product name (or 'done' to finish): ")
if product.lower() == 'done':
break
try:
price = float(input(f"Enter price for {product}: "))
if price < 0:
raise ValueError("Price cannot be negative")
products[product] = price
except ValueError as e:
print(f"Invalid input: {e}")
写入文件
with open('price_list.txt', 'w') as file:
file.write("{:<10} : {}\n".format("Product", "Price"))
file.write("-" * 20 + "\n")
total_price = 0.0
for product, price in products.items():
file.write(f"{product:<10} : ${price:>5.2f}\n")
total_price += price
file.write("-" * 20 + "\n")
file.write(f"{'Total':<10} : ${total_price:>5.2f}\n")
print("Price list saved to 'price_list.txt'")
八、读取文件中的数据
可以从文件中读取商品及其价格,并打印到控制台。
# 从文件读取数据并打印
try:
with open('price_list.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line, end='')
except FileNotFoundError:
print("File not found. Please make sure 'price_list.txt' exists.")
通过上述步骤,您已经学习了如何使用Python编写一个打印价格列表的程序,包括定义商品及其价格、格式化输出、添加用户交互、处理异常和数据验证、将数据写入文件以及从文件中读取数据。希望这些内容对您有所帮助!
总结:定义商品及其价格、格式化输出、计算总价格、添加用户交互、处理异常和数据验证、将数据写入文件、从文件中读取数据。这些步骤涵盖了一个基本的价格列表程序所需的所有功能。
相关问答FAQs:
如何在Python中创建和打印一个价格列表?
要创建和打印一个价格列表,可以使用Python的列表数据结构。首先,定义一个包含价格的列表,然后使用循环遍历并打印每个价格。示例代码如下:
prices = [10.99, 15.49, 7.99, 5.00]
for price in prices:
print(f"价格: {price}元")
这种方法简单易懂,适合初学者。
如何格式化价格输出以提高可读性?
为了提高输出价格的可读性,可以使用Python的格式化字符串功能。例如,使用format
方法或f-strings可以确保价格以两位小数形式显示。以下是一个示例:
prices = [10.99, 15.49, 7.99, 5.00]
for price in prices:
print(f"价格: {price:.2f}元")
这样,输出的价格更加整齐,便于阅读。
如何将价格列表保存到文件中?
如果需要将价格列表保存到文件中,可以使用Python的文件操作功能。以下是一个简单的示例,展示如何将价格写入一个文本文件:
prices = [10.99, 15.49, 7.99, 5.00]
with open('prices.txt', 'w') as file:
for price in prices:
file.write(f"{price:.2f}元\n")
通过这种方式,价格将被保存到名为prices.txt
的文件中,方便后续查看或处理。
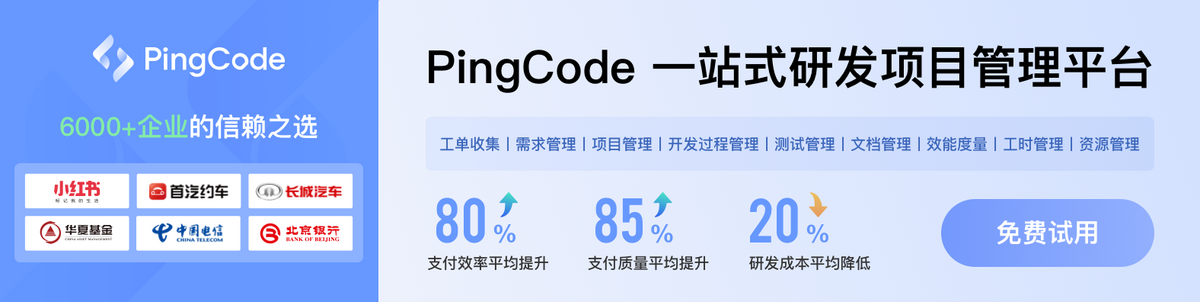