一、使用matplotlib中的自动退出功能、使用tkinter的定时退出、在终端中设置退出命令
使用matplotlib中的自动退出功能。Matplotlib是Python中最流行的绘图库之一,允许用户创建和自定义各种类型的图表。通过设置定时器,可以在绘图完成后自动退出程序。下面将详细介绍这一方法。
使用matplotlib
库绘图并自动退出,可以通过设置定时器来实现。首先,安装matplotlib
库(如果尚未安装):
pip install matplotlib
然后,使用以下代码示例来绘制图形并在指定时间后自动退出:
import matplotlib.pyplot as plt
from threading import Timer
def exit_program():
plt.close('all')
绘制图形
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('Sample Plot')
设置定时器,5秒后退出程序
t = Timer(5, exit_program)
t.start()
plt.show()
在上述代码中,使用threading.Timer
来设置定时器,exit_program
函数将在5秒后关闭所有图形窗口并退出程序。
二、使用tkinter的定时退出
Tkinter是Python的标准GUI库,可以用来创建图形用户界面。在绘图完成后,可以使用tkinter的定时功能来自动退出程序。
首先,安装tkinter
库(如果尚未安装):
pip install tk
然后,使用以下代码示例来创建图形并在指定时间后自动退出:
import tkinter as tk
from tkinter import Canvas
def exit_program():
root.destroy()
创建主窗口
root = tk.Tk()
root.title("Sample Plot")
创建Canvas对象
canvas = Canvas(root, width=400, height=300)
canvas.pack()
绘制图形
canvas.create_line(50, 50, 300, 200, fill="blue", width=2)
canvas.create_text(200, 150, text="Sample Plot", font=("Arial", 20))
设置定时器,5秒后退出程序
root.after(5000, exit_program)
运行主窗口
root.mainloop()
在上述代码中,使用root.after
方法来设置定时器,exit_program
函数将在5秒后关闭主窗口并退出程序。
三、在终端中设置退出命令
如果你在终端中运行Python脚本,可以使用os._exit
函数来实现自动退出。首先,安装os
库(如果尚未安装):
pip install os
然后,使用以下代码示例来绘制图形并在指定时间后自动退出:
import matplotlib.pyplot as plt
import os
from threading import Timer
def exit_program():
os._exit(0)
绘制图形
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('Sample Plot')
设置定时器,5秒后退出程序
t = Timer(5, exit_program)
t.start()
plt.show()
在上述代码中,使用threading.Timer
来设置定时器,exit_program
函数将在5秒后使用os._exit(0)
退出程序。
四、使用pyplot的动画功能
Matplotlib中的pyplot
模块提供了动画功能,可以用于创建动态图形。在动画结束后,可以自动关闭图形窗口并退出程序。
首先,安装matplotlib
库(如果尚未安装):
pip install matplotlib
然后,使用以下代码示例来创建动画并在动画结束后自动退出:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
def update(frame):
line.set_ydata([1, 2, 3, 4, frame])
return line,
创建图形和线条对象
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4, 5], [1, 2, 3, 4, 5])
创建动画对象
ani = animation.FuncAnimation(fig, update, frames=range(5), repeat=False)
设置定时器,动画结束后退出程序
ani.event_source.stop_after(5000)
plt.show()
在上述代码中,使用animation.FuncAnimation
来创建动画,ani.event_source.stop_after
方法将在5秒后停止动画并退出程序。
五、使用PyQt5库
PyQt5是Python的一个GUI库,可以用来创建图形用户界面。在绘图完成后,可以使用PyQt5的定时功能来自动退出程序。
首先,安装PyQt5
库(如果尚未安装):
pip install PyQt5
然后,使用以下代码示例来创建图形并在指定时间后自动退出:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5.QtCore import QTimer
import matplotlib.pyplot as plt
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建图形
self.figure, self.ax = plt.subplots()
self.ax.plot([1, 2, 3], [4, 5, 6])
self.ax.set_title('Sample Plot')
# 创建定时器,5秒后退出程序
self.timer = QTimer(self)
self.timer.timeout.connect(self.exit_program)
self.timer.start(5000)
def exit_program(self):
self.close()
sys.exit()
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
在上述代码中,使用QTimer
来设置定时器,exit_program
函数将在5秒后关闭主窗口并退出程序。
六、使用Pygame库
Pygame是Python的一个游戏开发库,可以用来创建图形用户界面和动画。在绘图完成后,可以使用Pygame的定时功能来自动退出程序。
首先,安装pygame
库(如果尚未安装):
pip install pygame
然后,使用以下代码示例来创建图形并在指定时间后自动退出:
import pygame
import sys
def exit_program():
pygame.quit()
sys.exit()
初始化Pygame
pygame.init()
创建窗口
screen = pygame.display.set_mode((400, 300))
pygame.display.set_caption("Sample Plot")
绘制图形
screen.fill((255, 255, 255))
pygame.draw.line(screen, (0, 0, 255), (50, 50), (300, 200), 2)
font = pygame.font.Font(None, 36)
text = font.render("Sample Plot", True, (0, 0, 0))
screen.blit(text, (150, 150))
设置定时器,5秒后退出程序
pygame.time.set_timer(pygame.USEREVENT, 5000)
事件循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.USEREVENT:
exit_program()
pygame.display.flip()
pygame.quit()
在上述代码中,使用pygame.time.set_timer
来设置定时器,在5秒后触发pygame.USEREVENT
事件,exit_program
函数将在事件触发后退出程序。
总结
本文介绍了如何在Python中绘图完成后自动退出程序的几种方法,包括使用matplotlib、tkinter、终端命令、pyplot动画、PyQt5和Pygame。通过设置定时器,可以在绘图完成后自动关闭图形窗口并退出程序。根据具体需求,选择合适的方法实现自动退出功能。
相关问答FAQs:
在使用Python进行绘图时,如何设置程序在完成绘图后自动退出?
在Python中,可以通过在绘图代码的最后添加plt.show()
或plt.close()
来确保程序在显示完图形后自动退出。使用plt.show()
会显示图形窗口,而plt.close()
可以在图形关闭后结束程序。确保你的绘图代码在这两条命令之前执行。
是否可以通过设置计时器让绘图窗口在指定时间后自动关闭?
是的,可以使用time.sleep()
函数来设置一个计时器。例如,绘图完成后,可以调用time.sleep(5)
来让程序等待5秒,然后使用plt.close()
来关闭图形窗口。这样用户可以在短时间内查看图形,之后程序将自动退出。
在使用Jupyter Notebook时,如何确保绘图完成后自动退出?
在Jupyter Notebook中,通常不需要手动退出,因为Notebook会自动管理绘图的显示。然而,如果你希望在绘图后清理输出,可以使用plt.clf()
清空当前图形或plt.close('all')
关闭所有打开的图形窗口。这样可以保持Notebook的整洁,确保不会留下多余的图形输出。
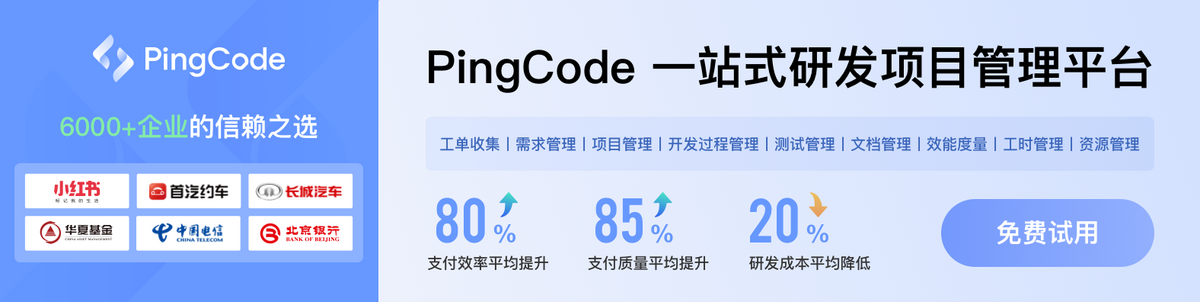