Python批量读取文件中的文本,可以使用循环、路径操作库和文件处理函数来实现。 首先,我们可以使用Python的os
库来遍历文件目录,然后利用open
函数逐个读取文件内容。使用os
库遍历文件目录、读取文件内容并处理、存储读取的数据,是实现这一目标的核心步骤。下面,我们将详细介绍如何实现这一过程。
一、导入必要的库
在开始批量读取文件之前,我们需要导入一些必要的库,比如os
和glob
。os
库可以帮助我们进行文件和目录的操作,而glob
库可以帮助我们匹配特定模式的文件。
import os
import glob
二、获取文件列表
我们需要指定一个目录路径,并获取该目录下的所有文件列表。可以通过os.listdir
或glob.glob
函数来实现。
# 使用os.listdir
directory_path = 'your_directory_path'
file_list = os.listdir(directory_path)
使用glob.glob
file_list = glob.glob(os.path.join(directory_path, '*.txt'))
三、读取文件内容
获取文件列表后,我们可以使用循环遍历每个文件,并使用open
函数打开文件,读取文件内容。可以选择逐行读取或者一次性读取整个文件内容。
for file_name in file_list:
file_path = os.path.join(directory_path, file_name)
with open(file_path, 'r', encoding='utf-8') as file:
file_content = file.read()
# 处理文件内容
print(file_content)
四、处理和存储数据
在读取文件内容后,我们可以根据需要对数据进行处理和存储。例如,可以将数据存储在列表或字典中,或者将处理后的数据写入新的文件。
all_contents = {}
for file_name in file_list:
file_path = os.path.join(directory_path, file_name)
with open(file_path, 'r', encoding='utf-8') as file:
file_content = file.read()
all_contents[file_name] = file_content
输出所有内容
for file_name, content in all_contents.items():
print(f'File: {file_name}')
print(content)
详细步骤和注意事项
1、导入必要的库
在Python中,os
库和glob
库是操作文件和目录的常用库。os
库提供了丰富的函数用于文件和目录操作,而glob
库则提供了文件名模式匹配功能,能够帮助我们找到符合特定模式的文件。
import os
import glob
2、获取文件列表
首先,我们需要指定要读取文件的目录路径。可以通过os.listdir
函数列出目录中的所有文件和目录,但我们通常只需要特定类型的文件,比如文本文件(.txt)。这时,可以使用glob
库来匹配特定模式的文件。
directory_path = 'your_directory_path'
file_list = glob.glob(os.path.join(directory_path, '*.txt'))
在上面的代码中,我们使用glob.glob
函数获取指定目录下所有以.txt
结尾的文件,并将其路径存储在file_list
列表中。
3、读取文件内容
获取文件列表后,我们可以使用循环遍历每个文件,并使用open
函数打开文件。可以选择逐行读取或者一次性读取整个文件内容。
for file_name in file_list:
file_path = os.path.join(directory_path, file_name)
with open(file_path, 'r', encoding='utf-8') as file:
file_content = file.read()
print(file_content)
在上面的代码中,我们使用with open(file_path, 'r', encoding='utf-8') as file
语句打开文件,并读取文件内容。使用with
语句可以确保文件在读取完毕后自动关闭。
4、处理和存储数据
在读取文件内容后,我们可以根据需要对数据进行处理和存储。例如,可以将数据存储在列表或字典中,或者将处理后的数据写入新的文件。
all_contents = {}
for file_name in file_list:
file_path = os.path.join(directory_path, file_name)
with open(file_path, 'r', encoding='utf-8') as file:
file_content = file.read()
all_contents[file_name] = file_content
输出所有内容
for file_name, content in all_contents.items():
print(f'File: {file_name}')
print(content)
在上面的代码中,我们将每个文件的内容存储在all_contents
字典中,其中键为文件名,值为文件内容。最后,我们遍历all_contents
字典,并打印每个文件的内容。
进一步优化和扩展
在实际应用中,我们可能需要对读取的文件内容进行进一步处理和分析。以下是一些常见的扩展和优化方法:
1、并行处理
如果需要读取和处理大量文件,可以考虑使用多线程或多进程来加速处理过程。Python的concurrent.futures
库提供了方便的并行处理接口。
from concurrent.futures import ThreadPoolExecutor
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
return file.read()
with ThreadPoolExecutor() as executor:
results = list(executor.map(read_file, file_list))
for file_name, content in zip(file_list, results):
print(f'File: {file_name}')
print(content)
在上面的代码中,我们使用ThreadPoolExecutor
并行读取文件内容,并将结果存储在results
列表中。
2、异常处理
在读取文件过程中,可能会遇到各种异常情况,比如文件不存在、权限不足等。可以使用try-except
语句捕获和处理这些异常,确保程序的健壮性。
for file_name in file_list:
file_path = os.path.join(directory_path, file_name)
try:
with open(file_path, 'r', encoding='utf-8') as file:
file_content = file.read()
print(file_content)
except Exception as e:
print(f'Error reading file {file_name}: {e}')
在上面的代码中,我们使用try-except
语句捕获并打印读取文件时发生的异常。
3、文件内容处理
在读取文件内容后,可以根据需要对数据进行进一步处理和分析。比如,可以使用正则表达式提取特定信息、进行数据清洗和转换等。
import re
for file_name in file_list:
file_path = os.path.join(directory_path, file_name)
with open(file_path, 'r', encoding='utf-8') as file:
file_content = file.read()
# 使用正则表达式提取特定信息
matches = re.findall(r'\b\w+\b', file_content)
print(matches)
在上面的代码中,我们使用正则表达式匹配文件内容中的所有单词,并将其存储在matches
列表中。
通过上述步骤和方法,我们可以实现Python批量读取文件中的文本,并对读取的数据进行进一步处理和分析。根据具体需求,可以灵活调整和扩展代码,以满足不同的应用场景。
相关问答FAQs:
如何使用Python读取多个文件中的文本?
要批量读取文件中的文本,可以使用Python的os
模块结合open
函数。首先,使用os.listdir()
获取指定目录下的所有文件名,然后逐个打开文件并读取内容。示例代码如下:
import os
directory = '你的文件夹路径'
for filename in os.listdir(directory):
if filename.endswith('.txt'): # 只读取文本文件
with open(os.path.join(directory, filename), 'r', encoding='utf-8') as file:
content = file.read()
print(content) # 打印每个文件的内容
这种方式适合处理大量文件,确保文件编码正确以避免乱码。
在读取文本文件时,如何处理文件编码问题?
在读取文件时,可能会遇到编码不一致的问题。使用open
函数时,可以通过encoding
参数指定文件编码,如utf-8
、gbk
等。如果不确定文件编码,可以使用chardet
库自动检测编码。示例代码如下:
import chardet
with open('文件路径', 'rb') as f:
result = chardet.detect(f.read())
encoding = result['encoding']
with open('文件路径', 'r', encoding=encoding) as file:
content = file.read()
这样可以有效避免因编码不匹配导致的读取错误。
如何将读取的文本内容保存到一个新的文件中?
在读取多个文件的文本后,通常会希望将它们合并并保存到一个新文件中。使用open
函数可以创建或覆盖文件。以下是一个简单的示例:
output_file = '合并后的文件.txt'
with open(output_file, 'w', encoding='utf-8') as outfile:
for filename in os.listdir(directory):
if filename.endswith('.txt'):
with open(os.path.join(directory, filename), 'r', encoding='utf-8') as infile:
content = infile.read()
outfile.write(content + '\n') # 每个文件内容后添加换行
这种方法可以将所有读取的文本内容整齐地保存到一个文件中,方便后续使用。
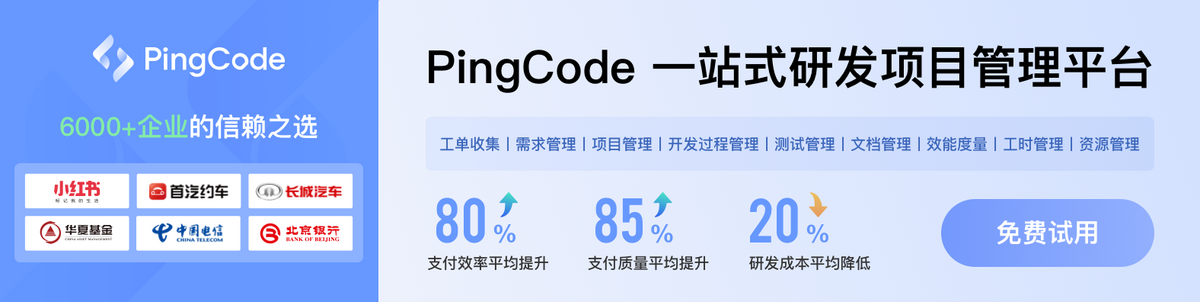