在Python中,修改Excel数据库连接的方法包括:使用适当的库(如pandas、openpyxl、xlrd)、读取Excel文件、更改连接参数、保存更改。以下将详细描述如何实现这一过程。
一、安装和导入所需的库
在使用Python与Excel文件进行交互时,常用的库有pandas、openpyxl、xlrd等。首先,需要确保这些库已经安装,并在代码中导入它们。
import pandas as pd
from openpyxl import load_workbook
二、读取Excel文件
使用pandas或openpyxl读取Excel文件。pandas提供了方便的read_excel
方法,而openpyxl则适用于更复杂的Excel文件操作。
# 使用pandas读取Excel文件
df = pd.read_excel('your_file.xlsx', sheet_name='Sheet1')
使用openpyxl读取Excel文件
wb = load_workbook('your_file.xlsx')
sheet = wb['Sheet1']
三、修改数据库连接字符串
假设Excel文件中某个单元格存储了数据库连接字符串,可以通过修改该单元格的值来更新连接字符串。
# 假设连接字符串在A1单元格
sheet['A1'] = 'new_connection_string'
四、保存更改
在修改完连接字符串后,需要将更改保存到Excel文件中。
wb.save('your_file.xlsx')
五、详细示例
以下是一个完整的示例,展示了如何使用Python修改Excel中的数据库连接字符串。
import pandas as pd
from openpyxl import load_workbook
读取Excel文件
file_path = 'your_file.xlsx'
wb = load_workbook(file_path)
sheet = wb['Sheet1']
修改连接字符串
old_connection_string = sheet['A1'].value
new_connection_string = 'your_new_connection_string'
sheet['A1'] = new_connection_string
保存更改
wb.save(file_path)
print(f"Successfully updated the connection string from {old_connection_string} to {new_connection_string}")
六、处理多张表和复杂操作
在实际应用中,可能需要处理多个工作表或进行更复杂的操作。以下是一些常见的场景和处理方法。
- 处理多个工作表:
可以使用for循环遍历所有工作表,并在每个工作表中修改连接字符串。
for sheet_name in wb.sheetnames:
sheet = wb[sheet_name]
# 假设连接字符串在每个工作表的A1单元格
sheet['A1'] = 'new_connection_string'
- 条件修改:
有时候需要根据特定条件修改连接字符串。例如,只修改连接字符串包含特定关键词的单元格。
for sheet_name in wb.sheetnames:
sheet = wb[sheet_name]
for row in sheet.iter_rows():
for cell in row:
if 'old_keyword' in str(cell.value):
cell.value = cell.value.replace('old_keyword', 'new_keyword')
- 处理复杂数据结构:
在一些复杂的Excel文件中,连接字符串可能嵌套在特定的结构中。可以结合使用pandas和openpyxl来处理这些情况。
for sheet_name in wb.sheetnames:
df = pd.read_excel(file_path, sheet_name=sheet_name)
# 假设连接字符串在某一列中
df['connection_column'] = df['connection_column'].apply(lambda x: x.replace('old_keyword', 'new_keyword') if isinstance(x, str) else x)
df.to_excel(file_path, sheet_name=sheet_name, index=False)
七、错误处理和日志记录
在进行文件操作时,添加错误处理和日志记录是非常重要的,以便在出现问题时能够快速定位和解决。
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
try:
file_path = 'your_file.xlsx'
wb = load_workbook(file_path)
for sheet_name in wb.sheetnames:
sheet = wb[sheet_name]
old_connection_string = sheet['A1'].value
new_connection_string = 'your_new_connection_string'
sheet['A1'] = new_connection_string
logging.info(f"Updated connection string in {sheet_name} from {old_connection_string} to {new_connection_string}")
wb.save(file_path)
logging.info("All changes saved successfully.")
except Exception as e:
logging.error(f"An error occurred: {e}")
以上是关于如何使用Python修改Excel数据库连接的详细步骤和示例。通过这些方法,可以灵活地读取、修改和保存Excel文件中的数据连接字符串,满足不同场景下的需求。
相关问答FAQs:
如何在Python中连接到不同的Excel文件?
在Python中连接到不同的Excel文件,可以使用pandas
库中的read_excel
函数。只需指定新的文件路径即可。例如:df = pd.read_excel('新的文件路径.xlsx')
。确保安装了openpyxl
或xlrd
库,以支持Excel文件的读取。
使用Python修改Excel数据库连接时需要注意哪些事项?
在修改Excel数据库连接时,需确认文件的路径是否正确,文件是否被其他程序占用,以及所需的库是否已正确安装。此外,确保使用的Excel文件格式(如.xlsx
或.xls
)与所用的库兼容,以避免读取错误。
Python如何处理Excel文件中的数据更新?
可以使用pandas
库中的to_excel
函数来更新Excel文件中的数据。首先,读取文件并进行相应的数据处理,之后使用df.to_excel('文件路径.xlsx', index=False)
将修改后的数据写回文件。确保在此过程中备份原始文件,以防数据丢失。
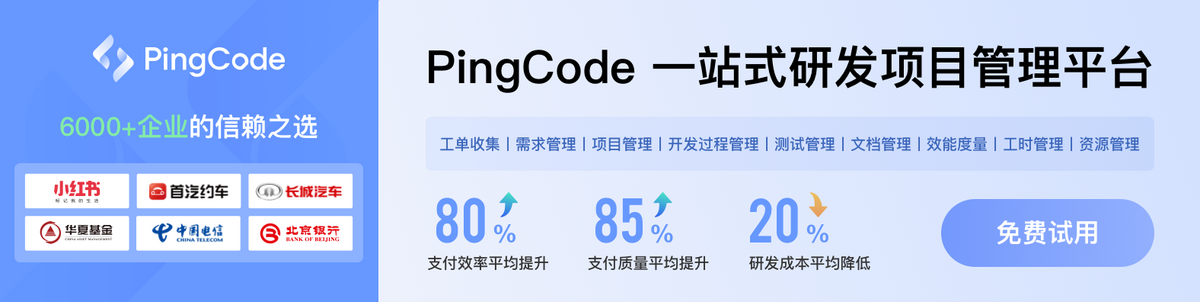