在Python中定义带有可选参数的函数可以使用默认参数、*args和kwargs。默认参数、*args、kwargs是Python中定义带有可选参数的函数的三种主要方式。下面将详细介绍使用默认参数的方式。
默认参数: 在函数定义中通过赋予参数一个默认值,可以使参数变成可选的。如果调用函数时没有传递该参数的值,函数会使用定义时指定的默认值。
def greet(name, message="Hello"):
print(f"{message}, {name}!")
在上面的例子中,message
是一个默认参数。如果调用函数greet
时不传递message
的值,它将使用默认值"Hello"
。
一、默认参数
默认参数是Python中最常见的可选参数。通过在函数定义时给参数赋予一个默认值,如果调用函数时没有提供该参数的值,那么函数就会使用这个默认值。
1、定义和使用默认参数
在函数定义时,可以为某些参数指定默认值:
def describe_pet(pet_name, animal_type='dog'):
"""Display information about a pet."""
print(f"\nI have a {animal_type}.")
print(f"My {animal_type}'s name is {pet_name}.")
在这个例子中,animal_type
有一个默认值'dog'
。如果在调用describe_pet
时不传递animal_type
,函数将使用默认值:
describe_pet('Willie')
输出:
I have a dog.
My dog's name is Willie.
当然,也可以传递animal_type
的值来覆盖默认值:
describe_pet('Harry', 'hamster')
输出:
I have a hamster.
My hamster's name is Harry.
2、多个默认参数
函数可以有多个默认参数:
def make_shirt(size='L', message='I love Python'):
print(f"\nThe size of the shirt is {size} and the message on it is '{message}'.")
make_shirt()
make_shirt(size='M')
make_shirt(message='Hello World')
make_shirt(size='S', message='Python is Fun')
输出:
The size of the shirt is L and the message on it is 'I love Python'.
The size of the shirt is M and the message on it is 'I love Python'.
The size of the shirt is L and the message on it is 'Hello World'.
The size of the shirt is S and the message on it is 'Python is Fun'.
二、*args
*args
允许函数接受任意数量的位置参数。这些参数会被存储在一个元组中,函数可以逐个处理它们。
1、定义和使用*args
def make_pizza(*toppings):
"""Print the list of toppings that have been requested."""
print("\nMaking a pizza with the following toppings:")
for topping in toppings:
print(f"- {topping}")
调用函数时,可以传递任意数量的参数:
make_pizza('pepperoni')
make_pizza('mushrooms', 'green peppers', 'extra cheese')
输出:
Making a pizza with the following toppings:
- pepperoni
Making a pizza with the following toppings:
- mushrooms
- green peppers
- extra cheese
2、结合位置参数和*args
可以将args与位置参数结合使用,但args必须放在位置参数之后:
def build_profile(first, last, *args):
"""Build a dictionary containing everything we know about a user."""
profile = {}
profile['first_name'] = first
profile['last_name'] = last
profile['additional_info'] = args
return profile
调用函数时,位置参数将被存储在first
和last
中,额外的参数将被存储在args
中:
user_profile = build_profile('albert', 'einstein', 'physicist', 'nobel prize winner')
print(user_profile)
输出:
{'first_name': 'albert', 'last_name': 'einstein', 'additional_info': ('physicist', 'nobel prize winner')}
三、kwargs
kwargs
允许函数接受任意数量的关键字参数。这些参数会被存储在一个字典中,函数可以逐个处理它们。
1、定义和使用kwargs
def build_profile(first, last, user_info):
"""Build a dictionary containing everything we know about a user."""
profile = {}
profile['first_name'] = first
profile['last_name'] = last
for key, value in user_info.items():
profile[key] = value
return profile
调用函数时,可以传递任意数量的关键字参数:
user_profile = build_profile('albert', 'einstein', location='princeton', field='physics')
print(user_profile)
输出:
{'first_name': 'albert', 'last_name': 'einstein', 'location': 'princeton', 'field': 'physics'}
2、结合位置参数、默认参数和kwargs
可以将kwargs与位置参数和默认参数结合使用,但kwargs必须放在参数列表的最后:
def make_car(manufacturer, model, options):
"""Build a dictionary containing everything we know about a car."""
car = {}
car['manufacturer'] = manufacturer
car['model'] = model
for key, value in options.items():
car[key] = value
return car
调用函数时,位置参数将被存储在manufacturer
和model
中,额外的关键字参数将被存储在options
中:
car = make_car('subaru', 'outback', color='blue', tow_package=True)
print(car)
输出:
{'manufacturer': 'subaru', 'model': 'outback', 'color': 'blue', 'tow_package': True}
四、总结
Python提供了多种方式来定义带有可选参数的函数:默认参数、*args和kwargs。默认参数允许在函数定义时为参数指定一个默认值,*args允许函数接受任意数量的位置参数,而kwargs允许函数接受任意数量的关键字参数。通过这三种方式,可以灵活地定义和使用带有可选参数的函数,提高代码的可读性和可维护性。
相关问答FAQs:
在Python中,如何定义一个带有默认值的可选参数的函数?
在Python中,可以通过在函数定义时为参数指定默认值来创建可选参数。默认值将用于未提供该参数值的情况。例如,定义一个函数greet
,可以如下实现:
def greet(name, greeting="Hello"):
return f"{greeting}, {name}!"
在调用greet("Alice")
时,将返回"Hello, Alice!"
,而调用greet("Alice", "Hi")
则返回"Hi, Alice!"
。
如果我想定义一个接受任意数量可选参数的函数,该怎么做?
可以使用*args
和<strong>kwargs
来定义可以接受任意数量位置参数和关键字参数的函数。*args
允许你传递多个位置参数,而</strong>kwargs
允许你传递多个关键字参数。示例如下:
def display_info(*args, **kwargs):
print("Positional arguments:", args)
print("Keyword arguments:", kwargs)
display_info(1, 2, 3, name="Alice", age=30)
在这个例子中,args
将包含位置参数,而kwargs
将包含关键字参数。
如何在函数中使用可选参数来实现函数重载的效果?
Python不支持函数重载,但可以通过使用可选参数和条件语句来模拟重载的效果。可以定义一个函数,根据传入参数的数量和类型执行不同的逻辑。例如:
def calculate_area(length, width=None):
if width is None:
return length * length # 处理正方形
return length * width # 处理矩形
print(calculate_area(5)) # 输出 25
print(calculate_area(5, 10)) # 输出 50
通过这种方式,可以根据不同的参数形式实现不同的功能。
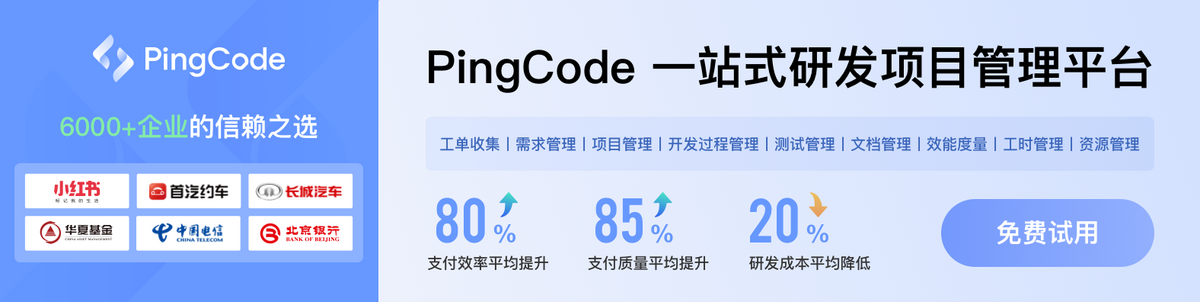