在python中画平面的反弹线,可以通过几个步骤来实现:使用库如matplotlib绘制图形、定义反弹线的起点和终点、计算反弹后的路径。使用matplotlib绘制、定义反射规则、实现反弹路径。
接下来,我们将详细说明每个步骤,并提供示例代码来展示如何实现这些步骤。
一、使用Matplotlib绘制平面
首先,我们需要安装matplotlib库(如果尚未安装),然后使用它来绘制一个平面。
import matplotlib.pyplot as plt
def draw_plane():
plt.figure(figsize=(8, 6))
plt.axhline(0, color='black', linewidth=1)
plt.axvline(0, color='black', linewidth=1)
plt.xlim(-10, 10)
plt.ylim(-10, 10)
plt.gca().set_aspect('equal', adjustable='box')
plt.grid(True)
plt.title('Plane with Rebounding Line')
plt.show()
draw_plane()
二、定义反弹线的起点和终点
接下来,我们定义反弹线的起点和终点,并计算反弹后的路径。
import numpy as np
Define start and end points
start_point = np.array([2, 3])
end_point = np.array([8, 9])
Plot the initial line
plt.plot([start_point[0], end_point[0]], [start_point[1], end_point[1]], 'r--', label='Initial Line')
plt.scatter([start_point[0], end_point[0]], [start_point[1], end_point[1]], color='red')
三、计算反弹后的路径
为了计算反弹后的路径,我们需要考虑反射规则。假设线段在接触边界时会发生反弹。
def reflect(point, boundary):
if boundary == 'x':
return np.array([point[0], -point[1]])
elif boundary == 'y':
return np.array([-point[0], point[1]])
Reflect the end point over the x-axis
reflected_point_x = reflect(end_point, 'x')
Reflect the end point over the y-axis
reflected_point_y = reflect(end_point, 'y')
Plot the reflected lines
plt.plot([start_point[0], reflected_point_x[0]], [start_point[1], reflected_point_x[1]], 'b--', label='Reflected Line (x-axis)')
plt.plot([start_point[0], reflected_point_y[0]], [start_point[1], reflected_point_y[1]], 'g--', label='Reflected Line (y-axis)')
plt.scatter([reflected_point_x[0], reflected_point_y[0]], [reflected_point_x[1], reflected_point_y[1]], color='blue')
四、实现多次反弹路径
可以通过循环来实现多次反弹路径,并绘制这些路径。
num_reflections = 5
current_point = end_point
for i in range(num_reflections):
reflected_point_x = reflect(current_point, 'x')
reflected_point_y = reflect(current_point, 'y')
plt.plot([current_point[0], reflected_point_x[0]], [current_point[1], reflected_point_x[1]], 'b--')
plt.plot([current_point[0], reflected_point_y[0]], [current_point[1], reflected_point_y[1]], 'g--')
plt.scatter([reflected_point_x[0], reflected_point_y[0]], [reflected_point_x[1], reflected_point_y[1]], color='blue')
current_point = reflected_point_x
plt.legend()
plt.show()
五、总结
通过上述步骤,我们展示了如何使用Python和matplotlib库绘制平面的反弹线。首先,我们定义了一个平面,然后绘制了初始线段。接下来,我们计算了线段在接触边界时的反弹,并通过循环实现了多次反弹路径。最后,我们使用matplotlib库绘制了所有这些路径,并展示了结果。
这只是一个基本的示例,实际应用中可能需要根据具体需求进行调整和扩展。希望这篇文章能为您在Python中绘制平面的反弹线提供帮助。
相关问答FAQs:
如何在Python中绘制反弹线?
在Python中,绘制反弹线通常可以使用Matplotlib库。首先,确保已安装Matplotlib库。通过定义反弹线的起点和终点坐标,结合Matplotlib的plot功能,就能够绘制出反弹线。在实际应用中,可以根据需要自定义线条的颜色、样式和宽度,以增强图形的可视化效果。
反弹线的绘制需要哪些基础知识?
要绘制反弹线,用户需要掌握Python的基本语法以及如何使用Matplotlib库。此外,了解坐标系统和如何设置坐标轴会对绘图过程有所帮助。熟悉numpy库也有助于生成数据点,从而使得反弹线更加精确和美观。
可以在反弹线中添加标注吗?
当然可以!在使用Matplotlib绘制反弹线时,可以利用plt.text()
或plt.annotate()
函数在特定点上添加标注。这些标注可以用来解释反弹线的意义或提供额外的信息,例如价格水平、时间点等。这不仅能提高图形的可读性,还能帮助观众更好地理解数据背后的故事。
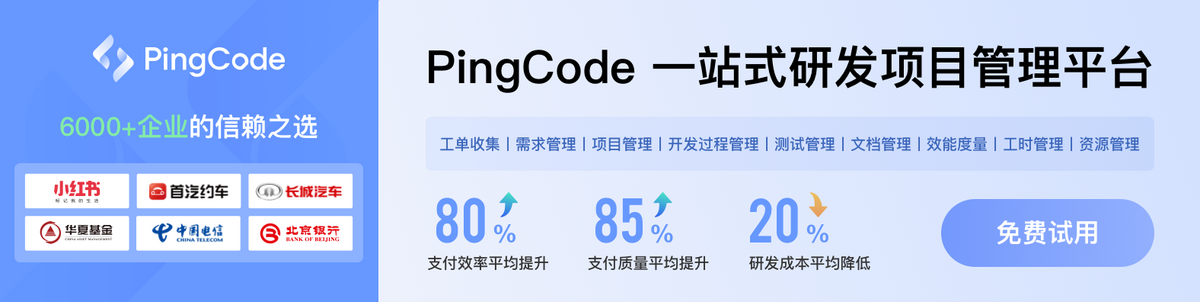