如何用Python搜索要用的素材
利用Python搜索素材可以通过以下几种方式实现:使用搜索引擎API、爬虫技术、利用现有的素材平台API、对搜索结果进行筛选。使用搜索引擎API是一种高效且便捷的方法。比如,Google Custom Search API和Bing Search API都提供了强大的搜索功能,可以直接获取搜索结果。
一、使用搜索引擎API
- Google Custom Search API
Google Custom Search API是一个强大的工具,允许开发者在他们的应用程序中嵌入Google搜索功能。首先,你需要在Google Developers Console中创建一个项目,并启用Custom Search API,然后获取API密钥和自定义搜索引擎ID。
import requests
def google_search(query, api_key, cse_id, num=10):
url = f"https://www.googleapis.com/customsearch/v1?q={query}&key={api_key}&cx={cse_id}&num={num}"
response = requests.get(url)
results = response.json()
return results
api_key = 'YOUR_API_KEY'
cse_id = 'YOUR_CSE_ID'
query = 'Python programming tutorials'
results = google_search(query, api_key, cse_id)
for item in results['items']:
print(f"Title: {item['title']}\nLink: {item['link']}\n")
- Bing Search API
Bing Search API是另一种强大的搜索引擎API。首先,你需要在Microsoft Azure门户中创建一个Bing Search资源,并获取API密钥。
import requests
def bing_search(query, api_key, count=10):
url = f"https://api.bing.microsoft.com/v7.0/search?q={query}&count={count}"
headers = {"Ocp-Apim-Subscription-Key": api_key}
response = requests.get(url, headers=headers)
results = response.json()
return results
api_key = 'YOUR_BING_API_KEY'
query = 'Python programming tutorials'
results = bing_search(query, api_key)
for item in results['webPages']['value']:
print(f"Title: {item['name']}\nLink: {item['url']}\n")
二、利用爬虫技术
利用爬虫技术可以从网页中提取所需的素材。常用的爬虫工具包括BeautifulSoup和Scrapy。
- BeautifulSoup
BeautifulSoup是一个用于解析HTML和XML的Python库,可以轻松地从网页中提取数据。
import requests
from bs4 import BeautifulSoup
def get_html_content(url):
response = requests.get(url)
return response.content
def parse_html(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
titles = soup.find_all('h2')
for title in titles:
print(title.text)
url = 'https://example.com'
html_content = get_html_content(url)
parse_html(html_content)
- Scrapy
Scrapy是一个功能强大的Python爬虫框架,适用于更复杂的数据提取任务。
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
for title in response.css('h2::text').getall():
yield {'title': title}
运行爬虫
scrapy runspider example_spider.py -o output.json
三、利用现有的素材平台API
许多素材平台提供了API,可以直接获取所需的素材。例如,Pexels和Unsplash提供了图片搜索API。
- Pexels API
Pexels API允许开发者搜索和获取高质量的图片。
import requests
def search_pexels(query, api_key, per_page=10):
url = f"https://api.pexels.com/v1/search?query={query}&per_page={per_page}"
headers = {"Authorization": api_key}
response = requests.get(url, headers=headers)
results = response.json()
return results
api_key = 'YOUR_PEXELS_API_KEY'
query = 'nature'
results = search_pexels(query, api_key)
for photo in results['photos']:
print(f"Photographer: {photo['photographer']}\nURL: {photo['url']}\n")
- Unsplash API
Unsplash API提供了免费的高质量图片搜索服务。
import requests
def search_unsplash(query, api_key, per_page=10):
url = f"https://api.unsplash.com/search/photos?query={query}&per_page={per_page}"
headers = {"Authorization": f"Client-ID {api_key}"}
response = requests.get(url, headers=headers)
results = response.json()
return results
api_key = 'YOUR_UNSPLASH_API_KEY'
query = 'mountains'
results = search_unsplash(query, api_key)
for photo in results['results']:
print(f"Photographer: {photo['user']['name']}\nURL: {photo['urls']['full']}\n")
四、对搜索结果进行筛选
在获取到搜索结果后,还需要对结果进行筛选,确保获得的是高质量和相关的素材。可以根据以下几点进行筛选:
- 相关性
确保搜索结果与搜索关键词高度相关,可以通过分析标题、描述等信息来判断。
- 质量
对于图片和视频等素材,可以根据分辨率、清晰度等指标来筛选高质量的素材。
- 来源
优先选择信誉良好的网站和平台提供的素材,确保素材的可靠性和合法性。
- 更新频率
优先选择最近更新的素材,确保获取的是最新的信息和资源。
通过以上几种方法和策略,利用Python搜索和获取所需的素材变得更加高效和便捷。无论是通过搜索引擎API、爬虫技术,还是利用现有的素材平台API,都可以帮助你快速找到所需的高质量素材。同时,对搜索结果进行筛选,确保获得的是相关且高质量的素材,从而提高工作效率和素材的应用效果。
相关问答FAQs:
如何使用Python自动化搜索素材?
可以利用Python中的网络爬虫库,比如BeautifulSoup和Requests,来自动化搜索所需的素材。通过编写脚本,指定关键词和目标网站,程序能够抓取页面内容并提取相关素材。此外,还可以结合API接口来获取更高质量的素材,比如使用Unsplash或Pexels的API。
有哪些Python库推荐用于素材搜索?
推荐使用BeautifulSoup进行HTML解析,Requests用于发送网络请求,Scrapy则是一个强大的爬虫框架,适合大规模爬取。对于图像处理和分析,可以考虑使用Pillow或OpenCV库。这些工具结合使用可以显著提高搜索素材的效率和质量。
在搜索素材时如何处理版权问题?
搜索素材时,确保遵循相关法律法规是至关重要的。可以优先选择使用开放版权或公共领域的素材,或者使用提供明确授权的素材库。通过API获取素材时,注意阅读并遵循各个平台的使用条款,确保合法使用素材以避免侵权。
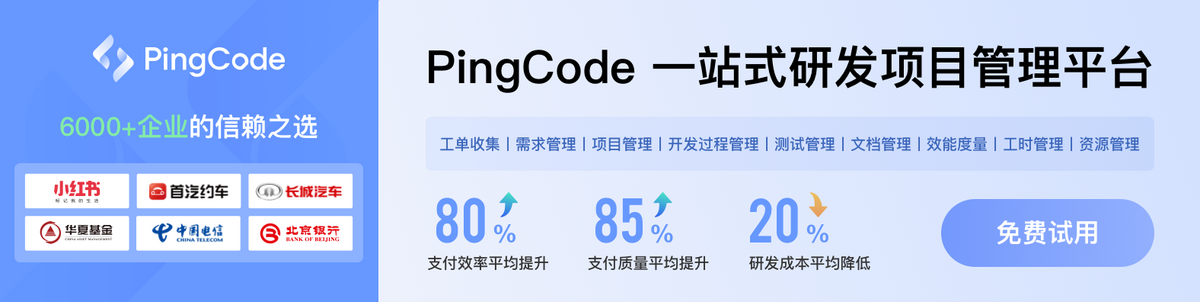