在Python中编写一个函数时,首先需要了解如何定义函数、参数传递、返回值的处理以及函数的调用。 函数的定义通常使用def
关键字,后跟函数名和括号,括号内可以包含参数。函数体用缩进表示。函数可以有返回值,也可以没有返回值。定义函数、参数传递、返回值的处理、函数的调用,这些都是编写Python函数的重要步骤。接下来,我们将详细解释这些步骤并提供示例。
一、定义函数
在Python中定义函数使用def
关键字,后面跟上函数名和圆括号,圆括号内可以包含参数列表。函数的主体包含在冒号后面的缩进块中。
def function_name(parameters):
# function body
pass
例如,定义一个简单的函数来计算两个数的和:
def add_numbers(a, b):
return a + b
在这个例子中,add_numbers
是函数名,a
和b
是参数,函数体返回这两个参数的和。
二、参数传递
函数可以接受任意数量的参数,参数可以是必需的、可选的或具有默认值的。以下是几种常见的参数传递方式:
1、位置参数
位置参数是最常见的参数类型,调用函数时参数按位置传递。
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # 输出: Hello, Alice!
2、关键字参数
关键字参数允许在调用函数时明确指定参数名称,这使得代码更具可读性。
def greet(name, message):
print(f"{message}, {name}!")
greet(name="Bob", message="Good morning") # 输出: Good morning, Bob!
3、默认参数
默认参数允许在函数定义时为参数指定默认值,如果调用函数时没有提供相应参数,则使用默认值。
def greet(name, message="Hello"):
print(f"{message}, {name}!")
greet("Charlie") # 输出: Hello, Charlie!
greet("David", "Hi") # 输出: Hi, David!
4、可变参数
可变参数允许函数接受任意数量的位置参数或关键字参数。使用*args
和kwargs
表示可变位置参数和可变关键字参数。
def greet(*names):
for name in names:
print(f"Hello, {name}!")
greet("Alice", "Bob", "Charlie") # 输出: Hello, Alice! Hello, Bob! Hello, Charlie!
def display_info(info):
for key, value in info.items():
print(f"{key}: {value}")
display_info(name="Alice", age=25, city="New York")
输出:
name: Alice
age: 25
city: New York
三、返回值的处理
函数可以返回一个值或多个值,使用return
关键字返回结果。
1、返回单个值
def add_numbers(a, b):
return a + b
result = add_numbers(3, 5)
print(result) # 输出: 8
2、返回多个值
函数可以返回多个值,使用逗号分隔返回值,返回的结果是一个元组。
def calculate(a, b):
sum_result = a + b
diff_result = a - b
return sum_result, diff_result
sum_val, diff_val = calculate(10, 5)
print(f"Sum: {sum_val}, Difference: {diff_val}")
输出: Sum: 15, Difference: 5
四、函数的调用
定义函数后,可以在代码的其他地方调用它。调用函数时,提供必要的参数,并接收返回值(如果有)。
def multiply(a, b):
return a * b
result = multiply(4, 5)
print(f"Multiplication Result: {result}")
输出: Multiplication Result: 20
五、函数的作用域
在编写函数时,了解变量的作用域很重要。Python中有两种主要的作用域:全局作用域和局部作用域。
1、全局作用域
全局变量在整个模块中都可以访问。
global_var = "I am global"
def print_global():
print(global_var)
print_global() # 输出: I am global
2、局部作用域
局部变量仅在函数内部可见。
def print_local():
local_var = "I am local"
print(local_var)
print_local() # 输出: I am local
print(local_var) # 报错: NameError: name 'local_var' is not defined
3、修改全局变量
使用global
关键字可以在函数内部修改全局变量。
counter = 0
def increment_counter():
global counter
counter += 1
increment_counter()
print(counter) # 输出: 1
六、嵌套函数和闭包
Python支持在函数内部定义函数,这称为嵌套函数。嵌套函数可以访问外部函数的变量,这种能力称为闭包。
1、嵌套函数
def outer_function():
def inner_function():
print("This is the inner function")
inner_function()
outer_function()
输出: This is the inner function
2、闭包
闭包是指内部函数可以记住并访问其外部函数的变量,即使外部函数已经完成执行。
def outer_function(msg):
def inner_function():
print(msg)
return inner_function
closure = outer_function("Hello, World!")
closure() # 输出: Hello, World!
七、递归函数
递归函数是指在函数内部调用自身的函数。递归函数通常用于解决分治问题,如计算阶乘、斐波那契数列等。
1、计算阶乘
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
print(factorial(5)) # 输出: 120
2、斐波那契数列
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(6)) # 输出: 8
八、匿名函数
匿名函数是指没有函数名的函数,使用lambda
关键字定义。匿名函数通常用于需要短期使用的简单函数。
add = lambda x, y: x + y
print(add(3, 5)) # 输出: 8
匿名函数可以作为参数传递给其他函数。
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x 2, numbers))
print(squared_numbers) # 输出: [1, 4, 9, 16, 25]
九、装饰器
装饰器是一个函数,接受另一个函数作为参数,并返回一个新的函数。装饰器用于在不修改原函数代码的情况下扩展其功能。
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
输出:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
十、文档字符串
文档字符串(Docstring)用于为函数提供说明文档。文档字符串使用三引号字符串定义,通常放在函数体的第一行。
def add_numbers(a, b):
"""
This function adds two numbers and returns the result.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
print(add_numbers.__doc__)
输出:
This function adds two numbers and returns the result.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
总结
编写Python函数是Python编程中的基础技能。通过掌握定义函数、参数传递、返回值的处理、函数的调用等知识,可以编写出高效、可重用和易维护的代码。此外,了解函数的作用域、嵌套函数和闭包、递归函数、匿名函数、装饰器等高级功能,可以进一步提升编程技巧和代码质量。在实际编程中,通过合理使用函数,可以提高代码的可读性和可维护性,减少代码重复,提高开发效率。
相关问答FAQs:
如何在Python中定义一个函数?
在Python中,定义一个函数非常简单。你可以使用def
关键字,后面跟上函数名和括号。函数体需要缩进,通常包含你想要执行的代码。例如:
def my_function():
print("Hello, World!")
这个例子中,my_function
是函数名,调用它将打印“Hello, World!”。
如何给Python函数添加参数?
在函数定义中,可以在括号内添加参数。参数允许你向函数传递信息。例如:
def greet(name):
print(f"Hello, {name}!")
在这个例子中,greet
函数接受一个名为name
的参数,并打印出问候语。调用方式为:greet("Alice")
,将输出“Hello, Alice!”。
如何返回值到调用函数的地方?
Python的函数可以使用return
语句返回值。返回值可以是任何数据类型,如数字、字符串或列表。例如:
def add(a, b):
return a + b
在这个例子中,add
函数接受两个参数并返回它们的和。你可以这样调用它并保存结果:result = add(5, 3)
,result
将会是8。
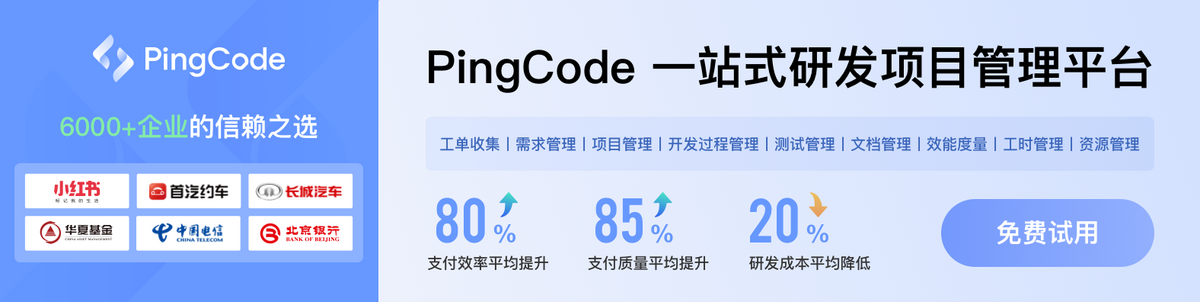