如何用Python控制微博粉丝数量
用Python控制微博粉丝数量可以通过以下方法:编写自动化脚本、利用微博API、数据分析、内容优化、互动管理。 其中,编写自动化脚本是实现这一目标的一个重要手段。通过编写自动化脚本,你可以自动化执行一些日常操作,如发布微博、点赞、关注等,从而增加你的微博曝光率和粉丝数量。
编写自动化脚本可以有效地减少手动操作的时间和精力,提高工作效率。你可以使用Python中的一些库,如Selenium、BeautifulSoup等,来编写这些自动化脚本。通过这些脚本,你可以自动化执行一些重复性的操作,如定时发布微博、自动点赞和关注等,从而增加你的微博活跃度和曝光率。
以下是详细内容:
一、编写自动化脚本
编写自动化脚本是通过Python控制微博粉丝数量的一个重要方法。自动化脚本可以帮助你自动化执行一些日常操作,从而增加微博的曝光率和粉丝数量。这里我们将介绍如何使用Selenium和BeautifulSoup来编写自动化脚本。
1、使用Selenium
Selenium是一个用于自动化网页操作的工具,可以通过Python与其结合使用来编写自动化脚本。以下是一个简单的示例,展示如何使用Selenium自动登录微博并发布一条微博:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
设置webdriver路径
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
打开微博登录页面
driver.get('https://weibo.com/login.php')
输入用户名和密码
username = driver.find_element_by_name('username')
password = driver.find_element_by_name('password')
username.send_keys('your_username')
password.send_keys('your_password')
点击登录按钮
login_button = driver.find_element_by_xpath('//a[@node-type="submitBtn"]')
login_button.click()
等待页面加载
time.sleep(5)
发布一条微博
weibo_content = 'Hello, this is an automated post from Selenium!'
weibo_textarea = driver.find_element_by_xpath('//textarea[@node-type="textEl"]')
weibo_textarea.send_keys(weibo_content)
post_button = driver.find_element_by_xpath('//a[@action-type="post"]')
post_button.click()
关闭浏览器
driver.quit()
2、使用BeautifulSoup
BeautifulSoup是一个用于解析HTML和XML文档的Python库,可以帮助你从网页中提取数据。以下是一个示例,展示如何使用BeautifulSoup从微博页面中提取粉丝数量:
import requests
from bs4 import BeautifulSoup
发送请求获取微博页面
url = 'https://weibo.com/u/your_user_id'
response = requests.get(url)
解析页面内容
soup = BeautifulSoup(response.content, 'html.parser')
提取粉丝数量
fans_count = soup.find('span', class_='fans_count').text
print(f'当前粉丝数量: {fans_count}')
二、利用微博API
微博API提供了一系列的接口,可以帮助你获取微博数据和执行一些操作。通过调用微博API,你可以实现自动化发布微博、获取粉丝列表等功能。以下是一些常用的微博API接口及其用法。
1、获取Access Token
在使用微博API之前,你需要先获取Access Token。以下是一个示例,展示如何使用OAuth2.0协议获取Access Token:
import requests
微博OAuth2.0授权地址
auth_url = 'https://api.weibo.com/oauth2/authorize'
client_id = 'your_client_id'
redirect_uri = 'your_redirect_uri'
获取授权码
auth_response = requests.get(auth_url, params={
'client_id': client_id,
'redirect_uri': redirect_uri,
'response_type': 'code'
})
print(f'请访问以下地址并授权: {auth_response.url}')
auth_code = input('请输入授权码: ')
获取Access Token
token_url = 'https://api.weibo.com/oauth2/access_token'
token_response = requests.post(token_url, data={
'client_id': client_id,
'client_secret': 'your_client_secret',
'grant_type': 'authorization_code',
'code': auth_code,
'redirect_uri': redirect_uri
})
access_token = token_response.json()['access_token']
print(f'Access Token: {access_token}')
2、发布微博
使用获取的Access Token,你可以调用微博API发布微博。以下是一个示例,展示如何使用微博API发布一条微博:
import requests
发布微博接口地址
post_url = 'https://api.weibo.com/2/statuses/update.json'
access_token = 'your_access_token'
status = 'Hello, this is a post from Weibo API!'
发布微博
post_response = requests.post(post_url, data={
'access_token': access_token,
'status': status
})
print(f'发布结果: {post_response.json()}')
3、获取粉丝列表
使用微博API,你还可以获取粉丝列表。以下是一个示例,展示如何使用微博API获取粉丝列表:
import requests
获取粉丝列表接口地址
followers_url = 'https://api.weibo.com/2/friendships/followers.json'
access_token = 'your_access_token'
user_id = 'your_user_id'
获取粉丝列表
followers_response = requests.get(followers_url, params={
'access_token': access_token,
'uid': user_id,
'count': 10 # 获取前10个粉丝
})
followers = followers_response.json()['users']
for follower in followers:
print(f'粉丝昵称: {follower["screen_name"]}')
三、数据分析
数据分析可以帮助你了解微博的运营情况,从而制定更有效的策略来增加粉丝数量。通过分析微博的数据,你可以找到哪些内容受欢迎、哪些时间段发布微博效果更好等。以下是一些常用的数据分析方法。
1、内容分析
内容分析可以帮助你了解哪些类型的微博内容更受欢迎。你可以通过统计微博的点赞数、评论数和转发数来判断哪些内容更受粉丝喜爱。以下是一个示例,展示如何统计微博的点赞数、评论数和转发数:
import requests
获取用户微博列表接口地址
statuses_url = 'https://api.weibo.com/2/statuses/user_timeline.json'
access_token = 'your_access_token'
user_id = 'your_user_id'
获取用户微博列表
statuses_response = requests.get(statuses_url, params={
'access_token': access_token,
'uid': user_id,
'count': 10 # 获取前10条微博
})
statuses = statuses_response.json()['statuses']
for status in statuses:
print(f'微博内容: {status["text"]}')
print(f'点赞数: {status["attitudes_count"]}')
print(f'评论数: {status["comments_count"]}')
print(f'转发数: {status["reposts_count"]}')
print('--------------------------')
2、时间分析
时间分析可以帮助你了解哪些时间段发布微博效果更好。你可以通过统计不同时间段发布微博的点赞数、评论数和转发数来判断最佳的发布时间。以下是一个示例,展示如何统计不同时间段发布微博的效果:
import requests
from datetime import datetime
获取用户微博列表接口地址
statuses_url = 'https://api.weibo.com/2/statuses/user_timeline.json'
access_token = 'your_access_token'
user_id = 'your_user_id'
获取用户微博列表
statuses_response = requests.get(statuses_url, params={
'access_token': access_token,
'uid': user_id,
'count': 100 # 获取前100条微博
})
statuses = statuses_response.json()['statuses']
统计不同时间段发布微博的效果
time_effects = {}
for status in statuses:
created_at = datetime.strptime(status['created_at'], '%a %b %d %H:%M:%S %z %Y')
hour = created_at.hour
if hour not in time_effects:
time_effects[hour] = {'attitudes': 0, 'comments': 0, 'reposts': 0, 'count': 0}
time_effects[hour]['attitudes'] += status['attitudes_count']
time_effects[hour]['comments'] += status['comments_count']
time_effects[hour]['reposts'] += status['reposts_count']
time_effects[hour]['count'] += 1
for hour, effects in time_effects.items():
print(f'时间段: {hour}:00 - {hour}:59')
print(f'平均点赞数: {effects["attitudes"] / effects["count"]}')
print(f'平均评论数: {effects["comments"] / effects["count"]}')
print(f'平均转发数: {effects["reposts"] / effects["count"]}')
print('--------------------------')
四、内容优化
内容优化是通过提高微博内容的质量和吸引力来增加粉丝数量的重要方法。通过发布高质量、有趣和有价值的内容,你可以吸引更多的用户关注你的微博。以下是一些内容优化的策略。
1、发布高质量内容
高质量的内容是吸引粉丝的关键。你可以通过发布有趣、有价值和有吸引力的内容来吸引更多的用户关注你的微博。以下是一些发布高质量内容的建议:
- 原创内容:发布原创内容可以展示你的独特观点和个性,从而吸引更多的用户关注。
- 图文并茂:在微博中添加图片和视频可以增加内容的吸引力,从而吸引更多的用户阅读和互动。
- 热点话题:参与热点话题讨论可以增加微博的曝光率,从而吸引更多的用户关注。
2、定期更新
定期更新微博内容可以保持用户的关注度和活跃度。你可以制定一个发布计划,定期发布新内容,从而保持微博的活跃度。以下是一些定期更新的建议:
- 制定发布计划:制定一个详细的发布计划,包括发布的频率、时间和内容类型。
- 使用定时发布工具:使用定时发布工具可以帮助你自动化发布微博内容,从而保持定期更新。
- 保持互动:定期与粉丝互动可以增加用户的粘性和活跃度,从而增加粉丝数量。
五、互动管理
互动管理是通过与粉丝进行互动来增加粉丝数量的重要方法。通过积极与粉丝互动,你可以增加用户的粘性和活跃度,从而吸引更多的用户关注你的微博。以下是一些互动管理的策略。
1、回复评论
回复粉丝的评论可以增加用户的粘性和活跃度。你可以通过回复粉丝的评论来展示你的关心和互动,从而增加用户的满意度和忠诚度。以下是一个示例,展示如何使用微博API回复粉丝的评论:
import requests
回复评论接口地址
reply_url = 'https://api.weibo.com/2/comments/reply.json'
access_token = 'your_access_token'
comment_id = 'comment_id_to_reply'
status_id = 'status_id_of_the_comment'
reply_text = 'Thank you for your comment!'
回复评论
reply_response = requests.post(reply_url, data={
'access_token': access_token,
'cid': comment_id,
'id': status_id,
'comment': reply_text
})
print(f'回复结果: {reply_response.json()}')
2、举办活动
举办活动是增加粉丝互动和活跃度的有效方法。你可以通过举办各种活动,如抽奖、有奖问答等,来吸引更多的用户参与和关注。以下是一些举办活动的建议:
- 抽奖活动:通过举办抽奖活动可以吸引更多的用户参与和关注,从而增加粉丝数量。
- 有奖问答:通过举办有奖问答活动可以增加用户的互动和参与度,从而增加粉丝数量。
- 合作推广:与其他微博用户或品牌合作举办活动可以增加微博的曝光率和粉丝数量。
综上所述,通过编写自动化脚本、利用微博API、数据分析、内容优化和互动管理,你可以有效地控制微博粉丝数量。希望这些方法和建议可以帮助你在微博上取得更好的运营效果。
相关问答FAQs:
如何使用Python自动化管理我的微博粉丝?
使用Python进行微博粉丝管理,可以通过微博的API接口实现。首先,你需要申请一个开发者账号并获取API密钥。之后,利用Python的requests库可以向微博的API发送请求,获取粉丝数据、关注用户或执行其他操作。请确保遵守微博的使用条款,避免刷粉或其他违规行为。
Python控制微博粉丝数量的实现难度大吗?
实现难度取决于你的编程基础和对微博API的理解。如果你具备基本的Python编程知识,并了解API的使用,控制微博粉丝数量并不复杂。建议从简单的操作开始,例如获取粉丝列表,逐步深入到添加或删除粉丝的功能。
有哪些Python库可以帮助我管理微博账号?
常用的Python库包括requests和beautifulsoup4,前者用于发送HTTP请求,后者适合进行网页解析。此外,还有一些第三方库专门为微博API开发,例如weibo-py,这些库能简化与微博的交互,帮助你更高效地管理粉丝。使用这些库时,请确保遵循相关的API使用规范。
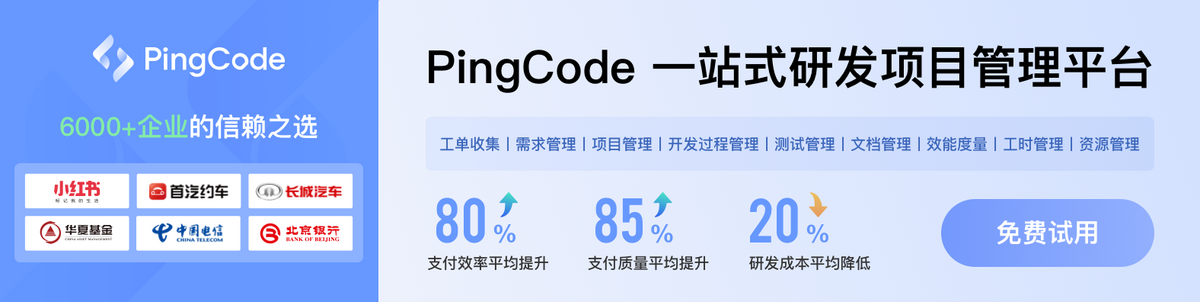