Python检查字符串是否为数字的方法有多种,包括使用内置函数、正则表达式以及其他库。常见方法有:str.isdigit()
、str.isnumeric()
、str.isdecimal()
、正则表达式和使用try-except
块来捕获转换异常。下面我们将详细描述其中一些方法及其适用场景。
一、使用内置方法
1. 使用 str.isdigit()
str.isdigit() 是最常用的方法之一,它检查字符串中的每个字符是否都是数字字符。它适用于所有阿拉伯数字字符。
def is_digit(s):
return s.isdigit()
示例
print(is_digit("12345")) # 输出: True
print(is_digit("1234a5")) # 输出: False
2. 使用 str.isnumeric()
str.isnumeric() 方法与 str.isdigit()
类似,但它还包括其他形式的数字字符,如罗马数字和中文数字。
def is_numeric(s):
return s.isnumeric()
示例
print(is_numeric("12345")) # 输出: True
print(is_numeric("一二三四五")) # 输出: True
print(is_numeric("1234a5")) # 输出: False
3. 使用 str.isdecimal()
str.isdecimal() 方法检查字符串中的每个字符是否都是十进制字符,它是最严格的检查方法,仅适用于纯数字。
def is_decimal(s):
return s.isdecimal()
示例
print(is_decimal("12345")) # 输出: True
print(is_decimal("123.45")) # 输出: False
二、使用正则表达式
正则表达式提供了更灵活的方式来检查字符串是否为数字,尤其适用于复杂的数字格式。
import re
def is_numeric_regex(s):
pattern = re.compile(r'^\d+$')
return bool(pattern.match(s))
示例
print(is_numeric_regex("12345")) # 输出: True
print(is_numeric_regex("1234a5")) # 输出: False
三、使用 try-except
块
使用 try-except
块可以捕获转换异常,以确定字符串是否可以转换为数字。这种方法适用于浮点数和整数。
def is_number(s):
try:
float(s)
return True
except ValueError:
return False
示例
print(is_number("12345")) # 输出: True
print(is_number("1234.5")) # 输出: True
print(is_number("1234a5")) # 输出: False
四、使用 str.translate()
和 str.maketrans()
这种方法可以用来移除字符串中的特定字符,并检查剩余部分是否为数字。
def is_number_translate(s):
trans_table = str.maketrans('', '', '.,')
cleaned_s = s.translate(trans_table)
return cleaned_s.isdigit()
示例
print(is_number_translate("12345")) # 输出: True
print(is_number_translate("12,345")) # 输出: True
print(is_number_translate("12.345")) # 输出: True
print(is_number_translate("12.34a5")) # 输出: False
五、使用外部库
1. 使用 numpy
库
numpy
提供了强大的数值处理功能,可以用于检查字符串是否为数字。
import numpy as np
def is_number_numpy(s):
try:
np.float64(s)
return True
except ValueError:
return False
示例
print(is_number_numpy("12345")) # 输出: True
print(is_number_numpy("1234.5")) # 输出: True
print(is_number_numpy("1234a5")) # 输出: False
2. 使用 pandas
库
pandas
也提供了类似的功能,通过其类型转换功能检查字符串是否为数字。
import pandas as pd
def is_number_pandas(s):
try:
pd.to_numeric(s)
return True
except ValueError:
return False
示例
print(is_number_pandas("12345")) # 输出: True
print(is_number_pandas("1234.5")) # 输出: True
print(is_number_pandas("1234a5")) # 输出: False
六、综合应用场景
在实际应用中,选择适当的方法取决于具体需求和数据的特性。例如:
1. 检查用户输入是否为整数
如果你需要检查用户输入的字符串是否为整数,可以使用 str.isdigit()
方法:
user_input = input("请输入一个整数: ")
if user_input.isdigit():
print("输入是一个整数")
else:
print("输入不是一个整数")
2. 检查浮点数输入
对于浮点数输入,可以使用 try-except
块:
user_input = input("请输入一个数字: ")
if is_number(user_input):
print("输入是一个数字")
else:
print("输入不是一个数字")
3. 处理带有逗号的数字
如果需要处理带有逗号的数字,可以使用 str.translate()
和 str.maketrans()
方法:
user_input = input("请输入一个数字: ")
if is_number_translate(user_input):
print("输入是一个数字")
else:
print("输入不是一个数字")
总结
在Python中检查字符串是否为数字的方法多种多样,选择适合的方法可以提高代码的可读性和效率。str.isdigit()、str.isnumeric()、str.isdecimal() 适用于简单的数字检查;正则表达式 提供了更灵活的方式;try-except 块适用于浮点数和更复杂的数字格式;外部库 如 numpy 和 pandas 提供了强大的数值处理功能。根据具体应用场景选择适当的方法,可以有效地解决字符串数字检查问题。
相关问答FAQs:
如何在Python中判断一个字符串是否表示数字?
在Python中,可以使用字符串的方法和异常处理来检查字符串是否是数字。最常用的方法是使用str.isdigit()
,该方法会返回True,如果字符串只包含数字字符。另一种方式是尝试将字符串转换为数字类型(如int
或float
),如果转换成功,则说明字符串是数字。
如果字符串包含空格或特殊字符,该如何处理?
如果字符串中包含空格或特殊字符,str.isdigit()
方法将返回False。为了处理这种情况,可以使用str.strip()
方法去除前后的空格,并结合正则表达式来检查字符串是否只包含数字。使用re.match(r'^\d+$', your_string)
可以帮助你验证字符串是否只包含数字。
如何处理不同数字格式的字符串,例如带小数点或负号的数字?
对于带小数点或负号的字符串,可以使用异常处理来判断。通过尝试将字符串转换为float
类型,如果没有引发异常,那么该字符串就可以被视为数字。示例代码如下:
def is_numeric(string):
try:
float(string)
return True
except ValueError:
return False
使用这种方法可以有效检测出包括整数、小数和负数在内的各种数字格式。
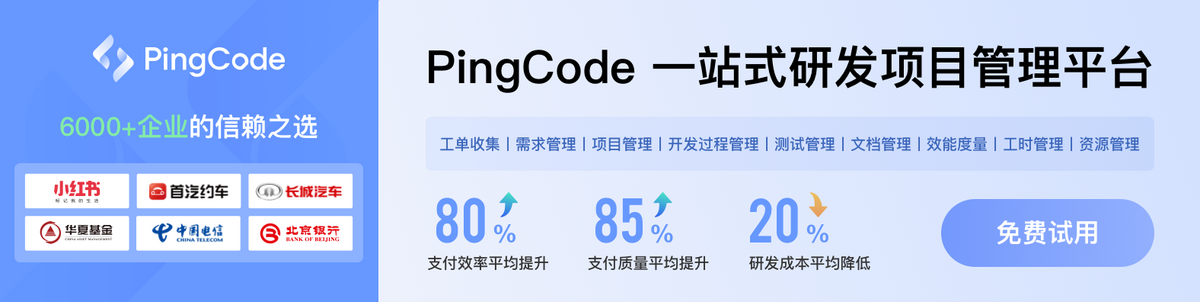