Python 只执行一次代码的方法包括:使用函数、使用类和单例模式、使用装饰器、使用全局变量和条件语句。 其中,使用单例模式是较为常见且有效的方法。通过单例模式,可以确保一个类只有一个实例,并提供一个全局访问点。接下来,我们将详细解释单例模式在 Python 中的实现,以及其他几种常用方法。
一、使用函数
使用函数是最简单的一种方法,通过定义一个函数并在需要的时候调用它,可以确保代码只执行一次。
def execute_once():
print("This code is executed once.")
execute_once()
在这个例子中,execute_once
函数中的代码只会在调用的时候执行一次。如果不再调用该函数,代码也不会再次执行。
二、使用类和单例模式
单例模式是一种设计模式,它确保一个类只有一个实例,并提供一个全局访问点。Python 中可以通过多种方式实现单例模式,以下是几种常见的实现方法:
- 使用类变量
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if not cls._instance:
cls._instance = super(Singleton, cls).__new__(cls, *args, kwargs)
return cls._instance
def __init__(self):
print("This code is executed once.")
singleton = Singleton()
singleton2 = Singleton()
在这个例子中,Singleton
类通过类变量_instance
来确保只有一个实例被创建,并且__init__
方法中的代码只会执行一次。
- 使用装饰器
def singleton(cls):
instances = {}
def get_instance(*args, kwargs):
if cls not in instances:
instances[cls] = cls(*args, kwargs)
return instances[cls]
return get_instance
@singleton
class Singleton:
def __init__(self):
print("This code is executed once.")
singleton = Singleton()
singleton2 = Singleton()
这个例子中,通过定义一个装饰器singleton
,可以将任何类转换为单例模式。装饰器通过一个字典instances
来存储类的实例,确保每个类只有一个实例。
三、使用全局变量和条件语句
使用全局变量和条件语句也可以确保代码只执行一次:
executed = False
def execute_once():
global executed
if not executed:
print("This code is executed once.")
executed = True
execute_once()
execute_once()
在这个例子中,通过全局变量executed
来记录代码是否已经执行过,从而确保代码只执行一次。
四、使用线程安全的单例模式
在多线程环境中,确保单例模式的线程安全是非常重要的。以下是一个线程安全的单例模式实现:
import threading
class Singleton:
_instance = None
_lock = threading.Lock()
def __new__(cls, *args, kwargs):
if not cls._instance:
with cls._lock:
if not cls._instance:
cls._instance = super(Singleton, cls).__new__(cls, *args, kwargs)
return cls._instance
def __init__(self):
print("This code is executed once.")
singleton = Singleton()
singleton2 = Singleton()
在这个例子中,通过使用threading.Lock
来确保代码在多线程环境中也是线程安全的。锁机制确保在创建实例的过程中不会有多个线程同时进入,确保单例模式的正确性。
五、使用模块级别的变量
在 Python 中,模块本身就是一个单例。可以利用这一特性,通过模块级别的变量来确保代码只执行一次:
# module_singleton.py
if 'singleton_instance' not in globals():
print("This code is executed once.")
singleton_instance = True
main.py
import module_singleton
import module_singleton
在这个例子中,通过检查模块的全局变量singleton_instance
是否存在,来确保代码只执行一次。由于模块在第一次导入时会执行一次,后续再次导入时不会重复执行,因此可以达到只执行一次的效果。
综上所述,Python 中有多种方法可以确保代码只执行一次,包括使用函数、使用类和单例模式、使用装饰器、使用全局变量和条件语句以及使用模块级别的变量。根据具体的需求和场景,选择合适的方法可以有效地确保代码的执行只发生一次。在实际开发中,单例模式是一种常用且有效的设计模式,可以很好地解决这一问题。通过多种实现方式,可以根据不同的需求选择合适的单例模式实现方式,从而确保代码的执行逻辑和性能优化。
相关问答FAQs:
在Python中,如何确保某段代码只在程序运行时执行一次?
为了确保某段代码只执行一次,可以使用模块级别的代码或函数内部的标志变量。通过在模块中定义一个变量来跟踪代码是否已经执行,可以有效避免重复执行。
使用什么工具或库可以帮助我实现只执行一次的代码?
可以使用threading
模块中的Lock
对象,以确保在多线程环境下某段代码只被执行一次。此外,contextlib
模块中的contextmanager
也可以用来创建只执行一次的代码块,提供上下文管理的灵活性。
在Python中,如何在类中实现某个方法只被调用一次?
可以在类的构造函数中设置一个标志属性,指示方法是否已被调用。通过检查这个标志,您可以控制方法的执行,确保它只在第一次调用时运行。这样可以有效地管理类的方法执行逻辑。
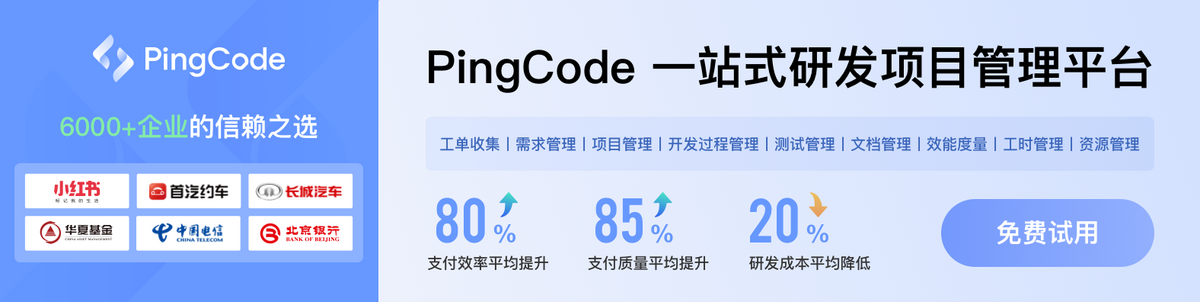