Python获取网页中指定信息的几种方法包括:使用requests库进行网页请求、使用BeautifulSoup进行HTML解析、使用正则表达式进行数据提取、使用Selenium进行动态网页内容获取。 其中,使用requests库和BeautifulSoup进行静态网页解析是最常见的方法。BeautifulSoup是一个功能强大的库,可以轻松地解析HTML和XML文档,并支持多种解析器。下面我们将详细介绍这些方法。
一、使用requests库进行网页请求
requests库是Python中一个非常流行的HTTP库,它可以用来发送HTTP请求并获取响应。使用requests库获取网页内容的步骤如下:
- 安装requests库:
pip install requests
- 发送HTTP请求并获取响应内容:
import requests
url = "http://example.com"
response = requests.get(url)
html_content = response.text
print(html_content)
在上述代码中,我们首先导入requests库,然后使用requests.get()
函数发送HTTP GET请求,并将响应内容存储在html_content
变量中。最后,打印出网页的HTML内容。
二、使用BeautifulSoup进行HTML解析
BeautifulSoup是一个功能强大的HTML和XML解析库,它可以帮助我们轻松地从网页中提取数据。使用BeautifulSoup解析HTML内容的步骤如下:
- 安装BeautifulSoup库:
pip install beautifulsoup4
- 解析HTML内容并提取指定信息:
from bs4 import BeautifulSoup
html_content = "<html><body><h1>Example</h1></body></html>"
soup = BeautifulSoup(html_content, "html.parser")
h1_tag = soup.find("h1")
print(h1_tag.text)
在上述代码中,我们首先导入BeautifulSoup库,然后将HTML内容解析成一个BeautifulSoup对象。接下来,我们使用soup.find()
函数查找指定的HTML标签,并打印出标签的文本内容。
三、使用正则表达式进行数据提取
正则表达式是一种强大的文本匹配工具,它可以用来从字符串中提取特定的模式。使用正则表达式提取网页内容的步骤如下:
- 导入re模块:
import re
- 编写正则表达式并提取数据:
html_content = "<html><body><h1>Example</h1></body></html>"
pattern = re.compile(r"<h1>(.*?)</h1>")
match = pattern.search(html_content)
if match:
print(match.group(1))
在上述代码中,我们首先导入re模块,然后编写一个正则表达式来匹配HTML标签中的文本内容。接下来,我们使用pattern.search()
函数查找匹配的文本,并打印出匹配的结果。
四、使用Selenium进行动态网页内容获取
Selenium是一个功能强大的浏览器自动化工具,它可以用来模拟用户操作并获取动态网页内容。使用Selenium获取网页内容的步骤如下:
- 安装Selenium库和WebDriver:
pip install selenium
- 启动浏览器并获取网页内容:
from selenium import webdriver
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
html_content = driver.page_source
print(html_content)
driver.quit()
在上述代码中,我们首先导入Selenium库,然后启动一个Chrome浏览器实例并访问指定的URL。接下来,我们使用driver.page_source
获取网页的HTML内容,并打印出来。最后,关闭浏览器实例。
详细介绍使用BeautifulSoup进行HTML解析
BeautifulSoup是一个非常强大的HTML解析库,它可以帮助我们轻松地从网页中提取数据。下面我们将详细介绍如何使用BeautifulSoup进行HTML解析。
1. 安装BeautifulSoup库
使用pip命令安装BeautifulSoup库:
pip install beautifulsoup4
2. 解析HTML内容
下面是一个简单的示例,演示如何使用BeautifulSoup解析HTML内容并提取指定的信息:
from bs4 import BeautifulSoup
html_content = """
<html>
<head>
<title>Example</title>
</head>
<body>
<h1>Heading 1</h1>
<p class="description">This is a paragraph.</p>
<a href="http://example.com">Example Link</a>
</body>
</html>
"""
soup = BeautifulSoup(html_content, "html.parser")
提取标题
title = soup.title.text
print("Title:", title)
提取指定标签
h1_tag = soup.find("h1")
print("H1 Tag:", h1_tag.text)
提取带有特定类名的标签
description = soup.find("p", class_="description")
print("Description:", description.text)
提取链接
link = soup.find("a")
print("Link:", link["href"])
在上述代码中,我们首先将HTML内容解析成一个BeautifulSoup对象。接下来,我们使用BeautifulSoup提供的各种方法来提取指定的信息:
soup.title.text
:提取页面标题。soup.find("h1")
:查找指定的HTML标签。soup.find("p", class_="description")
:查找带有特定类名的标签。soup.find("a")["href"]
:提取链接的URL。
3. 遍历和查找多个标签
有时候,我们需要从网页中提取多个相同类型的标签。我们可以使用find_all()
方法来查找多个标签:
html_content = """
<html>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
</body>
</html>
"""
soup = BeautifulSoup(html_content, "html.parser")
提取所有li标签
items = soup.find_all("li")
for item in items:
print("Item:", item.text)
在上述代码中,我们使用soup.find_all("li")
查找所有的<li>
标签,并遍历这些标签,打印出每个标签的文本内容。
4. 使用CSS选择器查找标签
BeautifulSoup还支持使用CSS选择器来查找标签。我们可以使用select()
方法来查找标签:
html_content = """
<html>
<body>
<div class="container">
<p class="text">Paragraph 1</p>
<p class="text">Paragraph 2</p>
</div>
</body>
</html>
"""
soup = BeautifulSoup(html_content, "html.parser")
使用CSS选择器查找标签
paragraphs = soup.select(".container .text")
for paragraph in paragraphs:
print("Paragraph:", paragraph.text)
在上述代码中,我们使用soup.select(".container .text")
查找带有特定类名的标签,并遍历这些标签,打印出每个标签的文本内容。
5. 处理嵌套结构
有时候,网页的HTML结构可能非常复杂,我们需要处理嵌套结构。下面是一个示例,演示如何处理嵌套结构:
html_content = """
<html>
<body>
<div class="parent">
<div class="child">
<p>Nested Paragraph</p>
</div>
</div>
</body>
</html>
"""
soup = BeautifulSoup(html_content, "html.parser")
查找嵌套结构中的标签
parent = soup.find("div", class_="parent")
child = parent.find("div", class_="child")
nested_paragraph = child.find("p")
print("Nested Paragraph:", nested_paragraph.text)
在上述代码中,我们首先查找父元素,然后在父元素中查找子元素,最后在子元素中查找嵌套的标签。
6. 提取属性值
有时候,我们需要从标签中提取属性值。我们可以使用get()
方法来提取属性值:
html_content = """
<html>
<body>
<a href="http://example.com" title="Example">Example Link</a>
</body>
</html>
"""
soup = BeautifulSoup(html_content, "html.parser")
提取链接的属性值
link = soup.find("a")
href = link.get("href")
title = link.get("title")
print("Href:", href)
print("Title:", title)
在上述代码中,我们使用link.get("href")
和link.get("title")
提取链接的URL和标题属性值。
7. 使用正则表达式查找标签
有时候,我们需要使用正则表达式来查找标签。我们可以使用re.compile()
函数编写正则表达式,并将其传递给find_all()
方法:
import re
from bs4 import BeautifulSoup
html_content = """
<html>
<body>
<p class="text">Paragraph 1</p>
<p class="text">Paragraph 2</p>
<p class="description">Description</p>
</body>
</html>
"""
soup = BeautifulSoup(html_content, "html.parser")
使用正则表达式查找标签
pattern = re.compile(r"text")
paragraphs = soup.find_all("p", class_=pattern)
for paragraph in paragraphs:
print("Paragraph:", paragraph.text)
在上述代码中,我们使用正则表达式re.compile(r"text")
来匹配类名包含“text”的标签,并遍历这些标签,打印出每个标签的文本内容。
详细介绍使用Selenium进行动态网页内容获取
Selenium是一个功能强大的浏览器自动化工具,它可以用来模拟用户操作并获取动态网页内容。下面我们将详细介绍如何使用Selenium获取动态网页内容。
1. 安装Selenium库和WebDriver
使用pip命令安装Selenium库:
pip install selenium
下载并安装WebDriver(例如ChromeDriver):
- ChromeDriver下载地址:https://sites.google.com/a/chromium.org/chromedriver/
2. 启动浏览器并获取网页内容
下面是一个简单的示例,演示如何使用Selenium启动浏览器并获取网页内容:
from selenium import webdriver
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
html_content = driver.page_source
print(html_content)
driver.quit()
在上述代码中,我们首先导入Selenium库,然后启动一个Chrome浏览器实例并访问指定的URL。接下来,我们使用driver.page_source
获取网页的HTML内容,并打印出来。最后,关闭浏览器实例。
3. 查找元素并提取信息
Selenium提供了多种方法来查找网页中的元素,并提取指定的信息。下面是一个示例,演示如何查找元素并提取信息:
from selenium import webdriver
from selenium.webdriver.common.by import By
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
查找元素并提取信息
title_element = driver.find_element(By.TAG_NAME, "title")
title = title_element.text
print("Title:", title)
h1_element = driver.find_element(By.TAG_NAME, "h1")
h1_text = h1_element.text
print("H1 Tag:", h1_text)
link_element = driver.find_element(By.TAG_NAME, "a")
link_href = link_element.get_attribute("href")
print("Link:", link_href)
driver.quit()
在上述代码中,我们使用driver.find_element(By.TAG_NAME, "title")
等方法查找网页中的元素,并提取指定的信息。
4. 模拟用户操作
Selenium还可以用来模拟用户操作,例如点击按钮、填写表单等。下面是一个示例,演示如何模拟用户操作:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
查找输入框并输入文本
search_box = driver.find_element(By.NAME, "q")
search_box.send_keys("Selenium")
search_box.send_keys(Keys.RETURN)
等待页面加载完成
driver.implicitly_wait(10)
查找搜索结果并提取信息
results = driver.find_elements(By.CSS_SELECTOR, "h3")
for result in results:
print("Result:", result.text)
driver.quit()
在上述代码中,我们首先查找搜索框元素,并使用search_box.send_keys()
方法输入搜索文本并模拟按下回车键。接下来,我们等待页面加载完成,并查找搜索结果,提取并打印搜索结果的文本内容。
5. 处理弹出框和对话框
有时候,网页中会包含弹出框和对话框,我们需要处理这些元素。下面是一个示例,演示如何处理弹出框和对话框:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.alert import Alert
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
模拟点击按钮,触发弹出框
button = driver.find_element(By.ID, "alertButton")
button.click()
切换到弹出框并处理
alert = Alert(driver)
print("Alert Text:", alert.text)
alert.accept()
driver.quit()
在上述代码中,我们首先查找按钮元素,并模拟点击按钮触发弹出框。接下来,我们切换到弹出框,并获取弹出框的文本内容,最后接受弹出框。
6. 处理多窗口和标签页
有时候,网页操作会打开多个窗口或标签页,我们需要处理这些窗口和标签页。下面是一个示例,演示如何处理多窗口和标签页:
from selenium import webdriver
from selenium.webdriver.common.by import By
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
模拟点击链接,打开新窗口
link = driver.find_element(By.ID, "newWindowLink")
link.click()
获取所有窗口句柄
window_handles = driver.window_handles
切换到新窗口
driver.switch_to.window(window_handles[1])
print("New Window Title:", driver.title)
关闭新窗口并切换回原窗口
driver.close()
driver.switch_to.window(window_handles[0])
print("Original Window Title:", driver.title)
driver.quit()
在上述代码中,我们首先查找链接元素,并模拟点击链接打开新窗口。接下来,我们获取所有窗口句柄,并切换到新窗口,打印新窗口的标题。最后,关闭新窗口并切换回原窗口,打印原窗口的标题。
7. 处理iframe
有时候,网页中会包含iframe,我们需要切换到iframe中处理元素。下面是一个示例,演示如何处理iframe:
from selenium import webdriver
from selenium.webdriver.common.by import By
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
切换到iframe
iframe = driver.find_element(By.ID, "iframe")
driver.switch_to.frame(iframe)
在iframe中查找元素并提取信息
h1_element = driver.find_element(By.TAG_NAME, "h1")
h1_text = h1_element.text
print("H1 Tag in iframe:", h1_text)
切换回主文档
driver.switch_to.default_content()
driver.quit()
在上述代码中,我们首先查找iframe元素,并切换到iframe中。接下来,我们在iframe中查找元素并提取信息。最后,切换回主文档。
8. 截屏和保存网页截图
Selenium还可以用来截屏并保存网页截图。下面是一个示例,演示如何截屏并保存网页截图:
from selenium import webdriver
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
截屏并保存网页截图
screenshot_path = "screenshot.png"
driver.save_screenshot(screenshot_path)
print("Screenshot saved to:", screenshot_path)
driver.quit()
在上述代码中,我们使用driver.save_screenshot()
方法截屏并保存网页截图。
综上所述,使用Python获取网页中指定的信息可以通过多种方法实现,包括使用requests库进行网页请求、使用BeautifulSoup进行HTML解析、使用正则表达式进行数据提取、使用Selenium进行动态网页内容获取。每种方法都有其适用的场景和优缺点,开发者可以根据具体需求选择合适的方法。
相关问答FAQs:
如何使用Python抓取网页数据?
要使用Python抓取网页数据,通常可以使用库如Requests和Beautiful Soup。Requests库用于发送HTTP请求,而Beautiful Soup则用于解析HTML文档。通过结合这两个库,可以轻松获取网页内容并提取所需的信息。
在抓取网页时,如何处理动态加载的内容?
许多网站使用JavaScript动态加载内容,这使得使用传统的Requests库获取信息变得复杂。为了解决这个问题,可以使用Selenium库,它能够模拟浏览器的行为,加载动态内容,从而获取所需的信息。使用Selenium时,需确保安装相应的浏览器驱动程序。
如何确保抓取网页时不违反法律或网站的使用条款?
在抓取网页之前,务必查看该网站的robots.txt文件,以了解其抓取政策。此外,仔细阅读网站的服务条款,确保您的抓取行为不违反相关规定。如果有疑问,可以考虑联系网站管理员请求许可,确保合法合规。
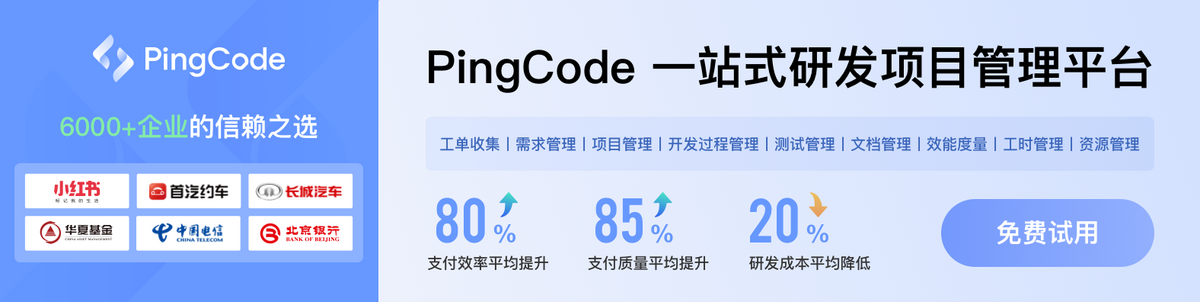