Python可以通过多种方式读取文件的某一行,使用文件对象的readlines()方法、循环遍历文件对象、或是使用第三方库。以下将详细介绍这些方法中的一种。
使用文件对象的readlines()
方法可以方便地读取文件中的所有行,并将其存储在一个列表中。然后,可以通过索引来访问特定的行。例如:
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1]
else:
return "Line number exceeds the total number of lines in the file."
示例调用
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
在这个示例中,read_specific_line
函数接受文件路径和行号作为参数,通过readlines()
方法读取所有行,并返回指定行的内容。若行号超出文件行数,则返回相应提示。
一、使用readlines()
方法
readlines()
方法是最常用的方法之一,尤其在文件内容较少时非常方便。此方法会将文件内容全部读取到内存中,适用于文件较小的情况。
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1]
else:
return "Line number exceeds the total number of lines in the file."
示例调用
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
在这个示例中,read_specific_line
函数接受文件路径和行号作为参数,通过readlines()
方法读取所有行,并返回指定行的内容。若行号超出文件行数,则返回相应提示。
二、使用循环遍历文件对象
在文件较大时,readlines()
方法可能会占用大量内存。此时,可以通过循环遍历文件对象,只读取需要的行。
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line
return "Line number exceeds the total number of lines in the file."
示例调用
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
这个方法通过枚举器enumerate
遍历文件每一行,直到找到所需行号。若行号超出文件行数,则返回相应提示。
三、使用linecache
模块
Python的linecache
模块可以直接读取文件的指定行,且无需手动处理文件打开和关闭,非常简洁。
import linecache
def read_specific_line(file_path, line_number):
line = linecache.getline(file_path, line_number)
if line:
return line
else:
return "Line number exceeds the total number of lines in the file."
示例调用
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
linecache.getline
函数将返回指定行的内容,若行号超出文件行数,则返回空字符串。
四、使用pandas
库
对于结构化数据文件(如CSV),可以使用pandas
库读取特定行。pandas
库提供了强大的数据处理功能,适用于复杂数据操作。
import pandas as pd
def read_specific_line(file_path, line_number):
try:
df = pd.read_csv(file_path, header=None)
if line_number <= len(df):
return df.iloc[line_number - 1]
else:
return "Line number exceeds the total number of lines in the file."
except Exception as e:
return str(e)
示例调用
file_path = 'example.csv'
line_number = 3
print(read_specific_line(file_path, line_number))
在这个示例中,read_specific_line
函数使用pandas
库读取CSV文件,并返回指定行的内容。若行号超出文件行数,则返回相应提示。
五、使用csv
模块
对于CSV文件,csv
模块也是一个常用的选择。此方法较为轻量,适用于较简单的CSV文件操作。
import csv
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
reader = csv.reader(file)
for current_line_number, line in enumerate(reader, start=1):
if current_line_number == line_number:
return line
return "Line number exceeds the total number of lines in the file."
示例调用
file_path = 'example.csv'
line_number = 3
print(read_specific_line(file_path, line_number))
这个方法通过csv.reader
读取CSV文件,并通过枚举器遍历每一行,直到找到所需行号。若行号超出文件行数,则返回相应提示。
六、使用io
模块
在某些情况下,可能需要从内存中的字符串读取特定行。此时,可以使用io
模块将字符串模拟为文件对象,然后进行读取。
import io
def read_specific_line_from_string(data, line_number):
file = io.StringIO(data)
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line
return "Line number exceeds the total number of lines in the file."
示例调用
data = """Line 1
Line 2
Line 3
Line 4"""
line_number = 3
print(read_specific_line_from_string(data, line_number))
在这个示例中,read_specific_line_from_string
函数接受字符串数据和行号作为参数,通过io.StringIO
将字符串模拟为文件对象,并返回指定行的内容。若行号超出数据行数,则返回相应提示。
七、处理大文件的优化方法
在处理大文件时,读取整个文件到内存可能会导致内存占用过高。可以通过分块读取的方式优化内存使用。
def read_specific_line_large_file(file_path, line_number, chunk_size=1024):
with open(file_path, 'r') as file:
current_line_number = 0
while True:
chunk = file.read(chunk_size)
if not chunk:
break
lines = chunk.splitlines(True)
for line in lines:
current_line_number += 1
if current_line_number == line_number:
return line
return "Line number exceeds the total number of lines in the file."
示例调用
file_path = 'large_example.txt'
line_number = 1000
print(read_specific_line_large_file(file_path, line_number))
在这个示例中,read_specific_line_large_file
函数通过分块读取文件,并逐行计数,直到找到指定行号。若行号超出文件行数,则返回相应提示。
八、使用mmap
模块
mmap
模块允许将文件映射到内存中,从而实现高效的文件读取操作。此方法适用于需要频繁访问大文件的情况。
import mmap
def read_specific_line_mmap(file_path, line_number):
with open(file_path, 'r') as file:
with mmap.mmap(file.fileno(), length=0, access=mmap.ACCESS_READ) as mm:
current_line_number = 0
for line in iter(mm.readline, b""):
current_line_number += 1
if current_line_number == line_number:
return line.decode('utf-8')
return "Line number exceeds the total number of lines in the file."
示例调用
file_path = 'large_example.txt'
line_number = 1000
print(read_specific_line_mmap(file_path, line_number))
在这个示例中,read_specific_line_mmap
函数通过mmap
模块将文件映射到内存,并逐行计数,直到找到指定行号。若行号超出文件行数,则返回相应提示。
九、总结
在Python中,读取文件的某一行有多种方法可供选择。根据文件大小、文件格式和内存使用情况,可以选择适当的方法。常用的方法包括使用readlines()
方法、循环遍历文件对象、linecache
模块、pandas
库、csv
模块、io
模块、分块读取和mmap
模块。
在处理较小文件时,readlines()
方法和循环遍历文件对象是较为常用的选择。
在处理较大文件时,分块读取和mmap
模块可以有效优化内存使用。
对于特定格式的文件,如CSV文件,可以使用pandas
库和csv
模块进行读取。
通过合理选择方法,可以高效地读取文件的某一行,并满足不同场景下的需求。
相关问答FAQs:
如何在Python中读取特定行的内容?
在Python中,读取特定行的内容可以通过多种方法实现。最常见的方法是使用文件的readlines()方法,它会将文件的每一行存储在一个列表中。您可以通过索引直接访问所需的行。例如,如果您想读取文件的第三行,可以使用如下代码:
with open('file.txt', 'r') as file:
lines = file.readlines()
specific_line = lines[2] # 索引从0开始,因此2表示第三行
这种方法简单直观,但对于非常大的文件,可能会占用较多内存。
如何使用enumerate函数读取特定行?
使用enumerate函数可以在遍历文件时同时获得行号,这样可以在读取文件时直接输出特定的行。例如,如果您想读取文件的第五行,可以这样做:
with open('file.txt', 'r') as file:
for index, line in enumerate(file):
if index == 4: # 4表示第五行
specific_line = line
break
这种方式在处理大文件时更加高效,因为它只读取到所需的行。
读取文件时如何处理异常情况?
在读取文件的过程中,可能会遇到一些异常情况,比如文件不存在或行号超出范围。为了更安全地处理这些问题,您可以使用try-except语句。例如:
try:
with open('file.txt', 'r') as file:
lines = file.readlines()
specific_line = lines[10] # 假设读取第11行
except FileNotFoundError:
print("文件未找到,请检查文件路径。")
except IndexError:
print("所请求的行超出文件的范围。")
这种方法可以确保您的程序在遇到错误时不会崩溃,而是给出友好的提示。
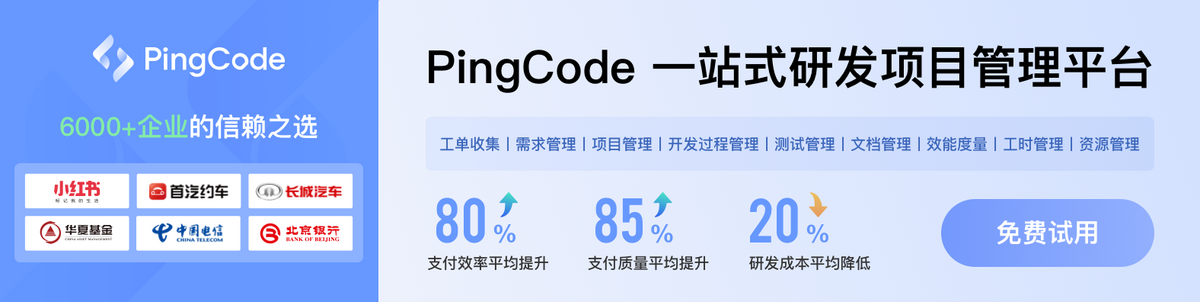