利用Python计算两点坐标间距的方法包括使用欧几里得距离公式、曼哈顿距离公式、以及使用内置库如NumPy、SciPy。其中,欧几里得距离公式是最常用的方法,它依据两点坐标的差值计算直线距离。
欧几里得距离公式的核心思想是基于平面几何中的勾股定理。具体来说,假设有两点A(x1, y1)和B(x2, y2),它们之间的距离d可以通过公式d = √((x2 – x1)^2 + (y2 – y1)^2)计算。这种方法适用于计算平面上任意两点之间的直线距离。
一、使用欧几里得距离公式
欧几里得距离公式是一种广泛应用于计算两点间距离的数学方法。它的计算方法非常简单,适用于各类坐标系。
1、基础计算方法
首先,假设我们有两个点A和B,它们的坐标分别为(x1, y1)和(x2, y2)。我们可以使用以下Python代码来计算它们之间的欧几里得距离:
import math
def euclidean_distance(x1, y1, x2, y2):
return math.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2)
示例
x1, y1 = 1, 2
x2, y2 = 4, 6
distance = euclidean_distance(x1, y1, x2, y2)
print(f"欧几里得距离: {distance}")
2、三维空间中的欧几里得距离
在三维空间中,欧几里得距离的计算方法类似,只需要增加一个维度z即可:
def euclidean_distance_3d(x1, y1, z1, x2, y2, z2):
return math.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2 + (z2 - z1)2)
示例
x1, y1, z1 = 1, 2, 3
x2, y2, z2 = 4, 6, 8
distance = euclidean_distance_3d(x1, y1, z1, x2, y2, z2)
print(f"三维空间中的欧几里得距离: {distance}")
二、使用曼哈顿距离公式
曼哈顿距离是另一种计算两点间距离的方法,它计算的是点与点之间的绝对差值之和。曼哈顿距离的公式为d = |x2 – x1| + |y2 – y1|。
1、基础计算方法
def manhattan_distance(x1, y1, x2, y2):
return abs(x2 - x1) + abs(y2 - y1)
示例
x1, y1 = 1, 2
x2, y2 = 4, 6
distance = manhattan_distance(x1, y1, x2, y2)
print(f"曼哈顿距离: {distance}")
2、三维空间中的曼哈顿距离
在三维空间中,曼哈顿距离的计算方法同样类似,只需增加一个维度z即可:
def manhattan_distance_3d(x1, y1, z1, x2, y2, z2):
return abs(x2 - x1) + abs(y2 - y1) + abs(z2 - z1)
示例
x1, y1, z1 = 1, 2, 3
x2, y2, z2 = 4, 6, 8
distance = manhattan_distance_3d(x1, y1, z1, x2, y2, z2)
print(f"三维空间中的曼哈顿距离: {distance}")
三、使用NumPy库
NumPy是一个强大的数值计算库,提供了许多方便的函数来计算两点间的距离。
1、使用NumPy计算欧几里得距离
NumPy的优势在于它能够处理多维数组和矩阵运算,因此在计算两点间的距离时更加高效:
import numpy as np
def numpy_euclidean_distance(x1, y1, x2, y2):
point1 = np.array([x1, y1])
point2 = np.array([x2, y2])
return np.linalg.norm(point1 - point2)
示例
x1, y1 = 1, 2
x2, y2 = 4, 6
distance = numpy_euclidean_distance(x1, y1, x2, y2)
print(f"使用NumPy计算的欧几里得距离: {distance}")
2、使用NumPy计算曼哈顿距离
同样,NumPy也可以方便地用于计算曼哈顿距离:
def numpy_manhattan_distance(x1, y1, x2, y2):
point1 = np.array([x1, y1])
point2 = np.array([x2, y2])
return np.sum(np.abs(point1 - point2))
示例
x1, y1 = 1, 2
x2, y2 = 4, 6
distance = numpy_manhattan_distance(x1, y1, x2, y2)
print(f"使用NumPy计算的曼哈顿距离: {distance}")
四、使用SciPy库
SciPy库是一个基于NumPy的科学计算库,它提供了更多的高级数学函数和计算工具。SciPy的距离模块(scipy.spatial.distance)提供了多种距离计算方法。
1、使用SciPy计算欧几里得距离
from scipy.spatial import distance
def scipy_euclidean_distance(x1, y1, x2, y2):
return distance.euclidean((x1, y1), (x2, y2))
示例
x1, y1 = 1, 2
x2, y2 = 4, 6
dist = scipy_euclidean_distance(x1, y1, x2, y2)
print(f"使用SciPy计算的欧几里得距离: {dist}")
2、使用SciPy计算曼哈顿距离
def scipy_manhattan_distance(x1, y1, x2, y2):
return distance.cityblock((x1, y1), (x2, y2))
示例
x1, y1 = 1, 2
x2, y2 = 4, 6
dist = scipy_manhattan_distance(x1, y1, x2, y2)
print(f"使用SciPy计算的曼哈顿距离: {dist}")
五、总结
通过以上几种方法,我们可以灵活地计算两点坐标间的距离。无论是使用基础的数学公式,还是借助NumPy和SciPy这样的强大库,Python都提供了丰富的工具来解决这一问题。
- 欧几里得距离公式:适用于计算平面和三维空间中的直线距离,简单易用。
- 曼哈顿距离公式:适用于计算绝对差值之和,适合网格化城市街道的距离计算。
- NumPy库:高效处理多维数组和矩阵运算,适合大规模数据的距离计算。
- SciPy库:提供更多高级数学函数和计算工具,适合科学计算和工程应用。
选择合适的方法和工具取决于具体的应用场景和数据规模。在实际应用中,我们可以根据需求灵活运用这些方法来解决问题。
相关问答FAQs:
如何在Python中计算两个坐标之间的距离?
在Python中,可以使用数学公式来计算两个点之间的距离。最常用的公式是欧几里得距离公式:
[ \text{distance} = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2} ]
可以利用Python的math
库来实现这一计算。以下是一个简单的示例代码:
import math
def calculate_distance(point1, point2):
return math.sqrt((point2[0] - point1[0])<strong>2 + (point2[1] - point1[1])</strong>2)
point_a = (1, 2)
point_b = (4, 6)
distance = calculate_distance(point_a, point_b)
print(f"两点之间的距离是: {distance}")
在Python中有什么库可以简化坐标距离的计算?
使用NumPy库可以简化坐标距离的计算。NumPy提供了高效的数组操作,可以通过内置的函数更快速地计算距离。例如,您可以使用numpy.linalg.norm
函数来计算两点之间的距离:
import numpy as np
point_a = np.array([1, 2])
point_b = np.array([4, 6])
distance = np.linalg.norm(point_b - point_a)
print(f"两点之间的距离是: {distance}")
这种方式不仅简洁,而且在处理大数据集时性能更优。
如何处理三维坐标点之间的距离计算?
对于三维坐标点,计算距离的方法与二维坐标类似,只需在公式中添加第三个维度的坐标。三维欧几里得距离公式为:
[ \text{distance} = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2 + (z_2 – z_1)^2} ]
在Python中,您可以像这样实现:
import math
def calculate_3d_distance(point1, point2):
return math.sqrt((point2[0] - point1[0])<strong>2 + (point2[1] - point1[1])</strong>2 + (point2[2] - point1[2])**2)
point_a = (1, 2, 3)
point_b = (4, 6, 8)
distance = calculate_3d_distance(point_a, point_b)
print(f"三维坐标点之间的距离是: {distance}")
这种方法同样适用于任意维度的坐标计算,只需扩展公式即可。
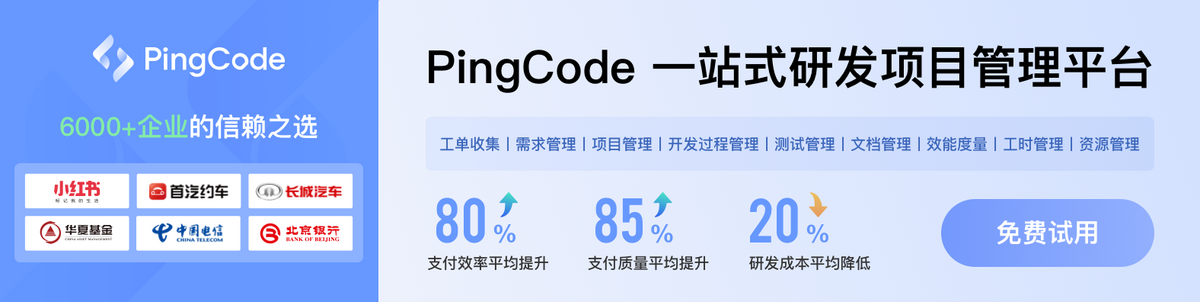