Python如何计算组合贷款计算器
组合贷款计算器在Python中的计算方法包括:计算贷款月供、计算还款总额、计算利息总额。 要详细描述其中的一个方面,我们可以重点讲解如何计算贷款月供。贷款月供的计算是基于贷款总额、贷款年限以及年利率,通过月供公式计算出每月需要偿还的金额,这对于个人财务规划和预算管理非常重要。
一、组合贷款计算器的基本概念
组合贷款通常是指购房者在购房时采用的商业贷款和公积金贷款相结合的贷款方式。这种方式可以有效地降低贷款利率,减轻购房者的还款压力。计算组合贷款月供、总还款额和总利息是购房者在选择组合贷款方式时需要重点考虑的内容。
二、贷款月供的计算
贷款月供的计算是组合贷款计算器的核心部分。贷款月供是指贷款人每月需要偿还的金额,它是基于贷款总额、贷款年限和年利率计算得出的。
1. 商业贷款月供计算
商业贷款的月供计算公式如下:
[ M = \frac{P \cdot \frac{r}{12} \cdot (1 + \frac{r}{12})^{n}}{(1 + \frac{r}{12})^{n} – 1} ]
其中:
- ( M ) 是每月月供
- ( P ) 是贷款本金
- ( r ) 是年利率
- ( n ) 是贷款期数(月)
2. 公积金贷款月供计算
公积金贷款月供计算公式与商业贷款类似:
[ M = \frac{P \cdot \frac{r}{12} \cdot (1 + \frac{r}{12})^{n}}{(1 + \frac{r}{12})^{n} – 1} ]
两个公式的区别仅在于年利率不同。
三、还款总额和利息总额的计算
还款总额是指贷款期内需要偿还的所有本金和利息的总和。利息总额是指贷款期内需要支付的所有利息的总和。
1. 商业贷款还款总额和利息总额
还款总额计算公式:
[ T = M \cdot n ]
利息总额计算公式:
[ I = T – P ]
其中:
- ( T ) 是还款总额
- ( I ) 是利息总额
- ( M ) 是每月月供
- ( n ) 是贷款期数(月)
- ( P ) 是贷款本金
2. 公积金贷款还款总额和利息总额
与商业贷款的计算方法相同,只是年利率不同。
四、Python实现组合贷款计算器
下面是一个Python实现组合贷款计算器的示例代码:
def calculate_monthly_payment(principal, annual_rate, years):
monthly_rate = annual_rate / 12 / 100
months = years * 12
monthly_payment = principal * monthly_rate * (1 + monthly_rate) <strong> months / ((1 + monthly_rate) </strong> months - 1)
return monthly_payment
def calculate_total_payment(monthly_payment, years):
return monthly_payment * years * 12
def calculate_total_interest(total_payment, principal):
return total_payment - principal
def main():
principal_commercial = float(input("请输入商业贷款本金(元):"))
annual_rate_commercial = float(input("请输入商业贷款年利率(%):"))
years_commercial = int(input("请输入商业贷款年限:"))
principal_housing_fund = float(input("请输入公积金贷款本金(元):"))
annual_rate_housing_fund = float(input("请输入公积金贷款年利率(%):"))
years_housing_fund = int(input("请输入公积金贷款年限:"))
monthly_payment_commercial = calculate_monthly_payment(principal_commercial, annual_rate_commercial, years_commercial)
total_payment_commercial = calculate_total_payment(monthly_payment_commercial, years_commercial)
total_interest_commercial = calculate_total_interest(total_payment_commercial, principal_commercial)
monthly_payment_housing_fund = calculate_monthly_payment(principal_housing_fund, annual_rate_housing_fund, years_housing_fund)
total_payment_housing_fund = calculate_total_payment(monthly_payment_housing_fund, years_housing_fund)
total_interest_housing_fund = calculate_total_interest(total_payment_housing_fund, principal_housing_fund)
total_monthly_payment = monthly_payment_commercial + monthly_payment_housing_fund
total_payment = total_payment_commercial + total_payment_housing_fund
total_interest = total_interest_commercial + total_interest_housing_fund
print(f"商业贷款每月月供:{monthly_payment_commercial:.2f} 元")
print(f"商业贷款还款总额:{total_payment_commercial:.2f} 元")
print(f"商业贷款利息总额:{total_interest_commercial:.2f} 元")
print(f"公积金贷款每月月供:{monthly_payment_housing_fund:.2f} 元")
print(f"公积金贷款还款总额:{total_payment_housing_fund:.2f} 元")
print(f"公积金贷款利息总额:{total_interest_housing_fund:.2f} 元")
print(f"组合贷款每月月供:{total_monthly_payment:.2f} 元")
print(f"组合贷款还款总额:{total_payment:.2f} 元")
print(f"组合贷款利息总额:{total_interest:.2f} 元")
if __name__ == "__main__":
main()
五、代码说明
1. 函数定义
在代码中,我们定义了三个主要函数:
calculate_monthly_payment
:计算每月月供calculate_total_payment
:计算还款总额calculate_total_interest
:计算利息总额
这些函数分别接受贷款本金、年利率和贷款年限作为参数,并返回相应的计算结果。
2. 用户输入
在main
函数中,我们通过input
函数获取用户输入的商业贷款和公积金贷款的本金、年利率和年限。这些输入值将用于调用上述函数进行计算。
3. 计算和输出
我们分别计算商业贷款和公积金贷款的每月月供、还款总额和利息总额。然后,将商业贷款和公积金贷款的结果相加,得到组合贷款的结果,并将这些结果输出到控制台。
六、优化和扩展
1. 利用GUI图形界面
为了提高用户体验,可以考虑使用Python的tkinter
或其他GUI库(如PyQt
)创建图形用户界面,使用户更方便地输入数据和查看结果。
2. 贷款提前还款计算
在实际应用中,贷款提前还款是一个常见的需求。可以扩展现有的计算器,增加提前还款的计算功能,包括提前还款的月数、提前还款的金额等。
3. 多种还款方式
除了等额本息还款(即每月月供相同),还有等额本金还款(即每月偿还相同的本金,利息逐月递减)。可以在现有计算器中增加等额本金还款的计算功能,供用户选择。
# 等额本金还款计算
def calculate_monthly_payment_principal(principal, annual_rate, years):
monthly_rate = annual_rate / 12 / 100
months = years * 12
monthly_principal = principal / months
total_payment = 0
for i in range(months):
monthly_payment = monthly_principal + (principal - i * monthly_principal) * monthly_rate
total_payment += monthly_payment
return total_payment / months, total_payment
def main():
principal_commercial = float(input("请输入商业贷款本金(元):"))
annual_rate_commercial = float(input("请输入商业贷款年利率(%):"))
years_commercial = int(input("请输入商业贷款年限:"))
principal_housing_fund = float(input("请输入公积金贷款本金(元):"))
annual_rate_housing_fund = float(input("请输入公积金贷款年利率(%):"))
years_housing_fund = int(input("请输入公积金贷款年限:"))
repayment_method = input("请选择还款方式(等额本息/等额本金):")
if repayment_method == "等额本息":
monthly_payment_commercial = calculate_monthly_payment(principal_commercial, annual_rate_commercial, years_commercial)
total_payment_commercial = calculate_total_payment(monthly_payment_commercial, years_commercial)
total_interest_commercial = calculate_total_interest(total_payment_commercial, principal_commercial)
monthly_payment_housing_fund = calculate_monthly_payment(principal_housing_fund, annual_rate_housing_fund, years_housing_fund)
total_payment_housing_fund = calculate_total_payment(monthly_payment_housing_fund, years_housing_fund)
total_interest_housing_fund = calculate_total_interest(total_payment_housing_fund, principal_housing_fund)
elif repayment_method == "等额本金":
monthly_payment_commercial, total_payment_commercial = calculate_monthly_payment_principal(principal_commercial, annual_rate_commercial, years_commercial)
total_interest_commercial = total_payment_commercial - principal_commercial
monthly_payment_housing_fund, total_payment_housing_fund = calculate_monthly_payment_principal(principal_housing_fund, annual_rate_housing_fund, years_housing_fund)
total_interest_housing_fund = total_payment_housing_fund - principal_housing_fund
else:
print("无效的还款方式")
return
total_monthly_payment = monthly_payment_commercial + monthly_payment_housing_fund
total_payment = total_payment_commercial + total_payment_housing_fund
total_interest = total_interest_commercial + total_interest_housing_fund
print(f"商业贷款每月月供:{monthly_payment_commercial:.2f} 元")
print(f"商业贷款还款总额:{total_payment_commercial:.2f} 元")
print(f"商业贷款利息总额:{total_interest_commercial:.2f} 元")
print(f"公积金贷款每月月供:{monthly_payment_housing_fund:.2f} 元")
print(f"公积金贷款还款总额:{total_payment_housing_fund:.2f} 元")
print(f"公积金贷款利息总额:{total_interest_housing_fund:.2f} 元")
print(f"组合贷款每月月供:{total_monthly_payment:.2f} 元")
print(f"组合贷款还款总额:{total_payment:.2f} 元")
print(f"组合贷款利息总额:{total_interest:.2f} 元")
if __name__ == "__main__":
main()
通过以上优化和扩展,可以使组合贷款计算器更加全面和实用,满足不同用户的需求。希望本文对您理解和实现组合贷款计算器有所帮助。如果有任何问题或建议,欢迎交流与讨论。
相关问答FAQs:
如何使用Python编写组合贷款计算器?
要编写组合贷款计算器,您可以使用Python中的基本数学库。首先,您需要了解组合贷款的基本概念,包括贷款金额、利率、贷款期限等。接下来,可以使用公式计算每种贷款的月供,然后将其合并。使用numpy
库也可以简化一些计算。
组合贷款计算器的主要功能有哪些?
组合贷款计算器的主要功能包括计算每个贷款的月供、总利息、还款总额,以及提供不同贷款组合的比较。用户可以输入贷款金额、利率和期限,程序将返回详细的还款计划和各类贷款的优势和劣势。
如何提高组合贷款计算器的用户体验?
为了提高用户体验,可以考虑提供图形用户界面(GUI)或Web应用程序,使用户可以更加直观地输入数据和查看结果。此外,添加数据验证、错误提示和帮助文档,确保用户在使用过程中有良好的引导和支持。提供多种贷款组合的比较功能也能让用户更轻松地做出明智的决策。
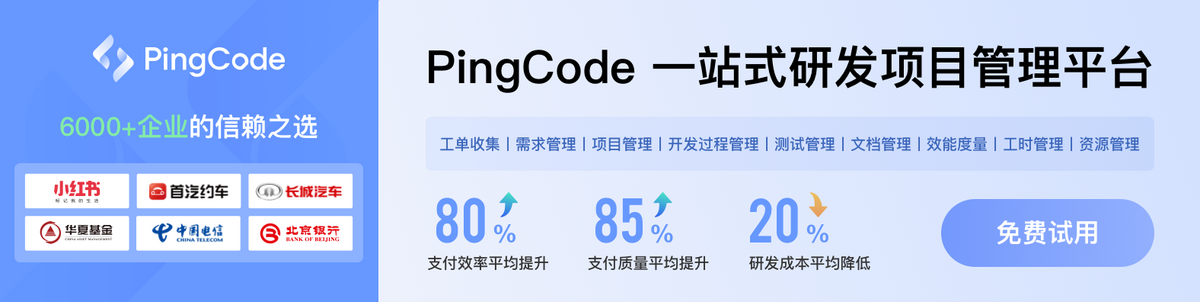