Python将图片序列化的方法有很多种,常见的方式包括使用Pillow库、base64编码、pickle模块等。 在本文中,我们将详细介绍如何使用这些方法将图片序列化,并给出每种方法的具体代码示例。其中,我们将重点介绍使用Pillow库和base64编码的方法。
一、使用Pillow库进行图片序列化
Pillow库是Python中处理图像的常用库,可以方便地读取、处理和保存图像。 使用Pillow库,我们可以将图片转换为字节数组,然后进行序列化。以下是使用Pillow库进行图片序列化的具体步骤:
1.安装Pillow库
在开始之前,我们需要确保已经安装了Pillow库。可以通过以下命令安装:
pip install pillow
2.读取图片并转换为字节数组
首先,我们需要读取图片文件并将其转换为字节数组。以下是具体的代码示例:
from PIL import Image
import io
def image_to_bytes(image_path):
with Image.open(image_path) as img:
byte_arr = io.BytesIO()
img.save(byte_arr, format=img.format)
return byte_arr.getvalue()
image_path = 'example.jpg'
image_bytes = image_to_bytes(image_path)
print(image_bytes)
在上述代码中,我们使用Image.open
方法读取图片文件,并使用io.BytesIO
将其转换为字节数组。最后,使用getvalue
方法获取字节数组。
3.将字节数组进行序列化
接下来,我们可以使用pickle
模块将字节数组进行序列化:
import pickle
def serialize_image(image_bytes):
return pickle.dumps(image_bytes)
serialized_image = serialize_image(image_bytes)
print(serialized_image)
在上述代码中,我们使用pickle.dumps
方法将字节数组进行序列化。
二、使用base64编码进行图片序列化
base64编码是一种常见的编码方式,可以将二进制数据转换为文本数据,便于传输和存储。 以下是使用base64编码进行图片序列化的具体步骤:
1.安装base64库
base64库是Python标准库的一部分,无需额外安装。
2.读取图片并进行base64编码
首先,我们需要读取图片文件并将其进行base64编码。以下是具体的代码示例:
import base64
def image_to_base64(image_path):
with open(image_path, 'rb') as img_file:
image_bytes = img_file.read()
return base64.b64encode(image_bytes).decode('utf-8')
image_base64 = image_to_base64(image_path)
print(image_base64)
在上述代码中,我们使用open
方法以二进制模式读取图片文件,并使用base64.b64encode
方法将其进行base64编码。
3.将base64编码后的数据进行序列化
接下来,我们可以使用pickle
模块将base64编码后的数据进行序列化:
def serialize_image_base64(image_base64):
return pickle.dumps(image_base64)
serialized_image_base64 = serialize_image_base64(image_base64)
print(serialized_image_base64)
在上述代码中,我们使用pickle.dumps
方法将base64编码后的数据进行序列化。
三、使用pickle模块进行图片序列化
pickle模块是Python标准库中的序列化模块,可以将Python对象序列化为字节流,并将字节流反序列化为Python对象。 以下是使用pickle模块进行图片序列化的具体步骤:
1.安装pickle库
pickle库是Python标准库的一部分,无需额外安装。
2.读取图片并进行序列化
首先,我们需要读取图片文件并将其进行序列化。以下是具体的代码示例:
def image_to_pickle(image_path):
with open(image_path, 'rb') as img_file:
image_bytes = img_file.read()
return pickle.dumps(image_bytes)
image_pickle = image_to_pickle(image_path)
print(image_pickle)
在上述代码中,我们使用open
方法以二进制模式读取图片文件,并使用pickle.dumps
方法将其进行序列化。
四、将序列化数据进行反序列化
无论使用哪种方法进行图片序列化,我们最终都需要将序列化的数据进行反序列化,以便恢复原始图片数据。 以下是将序列化数据进行反序列化的具体步骤:
1.使用pickle模块进行反序列化
我们可以使用pickle.loads
方法将序列化的数据进行反序列化:
def deserialize_image(serialized_image):
return pickle.loads(serialized_image)
deserialized_image = deserialize_image(serialized_image)
print(deserialized_image)
在上述代码中,我们使用pickle.loads
方法将序列化的数据进行反序列化。
2.保存反序列化后的图片
最后,我们可以将反序列化后的图片数据保存为图片文件。以下是具体的代码示例:
def save_image(image_bytes, output_path):
with open(output_path, 'wb') as img_file:
img_file.write(image_bytes)
output_path = 'output.jpg'
save_image(deserialized_image, output_path)
在上述代码中,我们使用open
方法以二进制模式打开输出文件,并使用write
方法将图片数据写入文件。
五、综合示例
为了更好地理解上述方法,我们将综合使用Pillow库和base64编码的方法,给出一个完整的示例。
1.序列化图片
首先,我们将图片进行序列化:
from PIL import Image
import io
import base64
import pickle
def image_to_bytes(image_path):
with Image.open(image_path) as img:
byte_arr = io.BytesIO()
img.save(byte_arr, format=img.format)
return byte_arr.getvalue()
def image_to_base64(image_path):
with open(image_path, 'rb') as img_file:
image_bytes = img_file.read()
return base64.b64encode(image_bytes).decode('utf-8')
def serialize_image(image_bytes):
return pickle.dumps(image_bytes)
image_path = 'example.jpg'
image_bytes = image_to_bytes(image_path)
image_base64 = image_to_base64(image_path)
serialized_image = serialize_image(image_bytes)
serialized_image_base64 = serialize_image(image_base64)
print(serialized_image)
print(serialized_image_base64)
2.反序列化图片
接下来,我们将序列化的数据进行反序列化:
def deserialize_image(serialized_image):
return pickle.loads(serialized_image)
deserialized_image = deserialize_image(serialized_image)
print(deserialized_image)
3.保存反序列化后的图片
最后,我们将反序列化后的图片数据保存为图片文件:
def save_image(image_bytes, output_path):
with open(output_path, 'wb') as img_file:
img_file.write(image_bytes)
output_path = 'output.jpg'
save_image(deserialized_image, output_path)
通过上述步骤,我们可以方便地将图片进行序列化和反序列化。
六、总结
通过本文的介绍,我们详细讲解了Python中将图片序列化的多种方法,包括使用Pillow库、base64编码和pickle模块。 每种方法都有其优缺点,具体选择哪种方法取决于具体的应用场景。希望本文对您理解和掌握Python中图片序列化的方法有所帮助。
相关问答FAQs:
如何在Python中将图片转换为字节流?
在Python中,可以使用Pillow库将图片转换为字节流。首先,您需要安装Pillow库,可以通过命令pip install Pillow
来完成。接着,您可以使用以下代码将图片读取为字节流:
from PIL import Image
import io
# 读取图片
image = Image.open('your_image.jpg')
# 创建一个字节流对象
byte_io = io.BytesIO()
# 将图片保存到字节流中
image.save(byte_io, format='JPEG')
# 获取字节流的内容
image_bytes = byte_io.getvalue()
这样,您就可以将图片序列化为字节流,方便进行网络传输或存储。
在Python中序列化图片后,如何将其存储到数据库中?
将图片序列化为字节流后,您可以将其存储到数据库中。许多数据库支持BLOB类型来存储二进制数据。以下是一个使用SQLite的示例:
import sqlite3
# 连接到数据库(或创建数据库)
conn = sqlite3.connect('images.db')
cursor = conn.cursor()
# 创建表
cursor.execute('CREATE TABLE IF NOT EXISTS Images (id INTEGER PRIMARY KEY, image BLOB)')
# 插入序列化的图片
cursor.execute('INSERT INTO Images (image) VALUES (?)', (image_bytes,))
conn.commit()
conn.close()
通过这种方式,您可以将序列化后的图片存储在数据库中,便于后续检索和使用。
如何从序列化的字节流中恢复图片?
恢复图片的过程同样简单。您可以从数据库中提取字节流,然后使用Pillow库将其转换回图片格式。以下是一个示例:
# 从数据库中读取字节流
conn = sqlite3.connect('images.db')
cursor = conn.cursor()
cursor.execute('SELECT image FROM Images WHERE id = ?', (1,))
image_bytes = cursor.fetchone()[0]
conn.close()
# 将字节流转换为图片
byte_io = io.BytesIO(image_bytes)
image = Image.open(byte_io)
image.show() # 或保存为文件
这样,您就可以轻松地从序列化的字节流中恢复出原始图片。
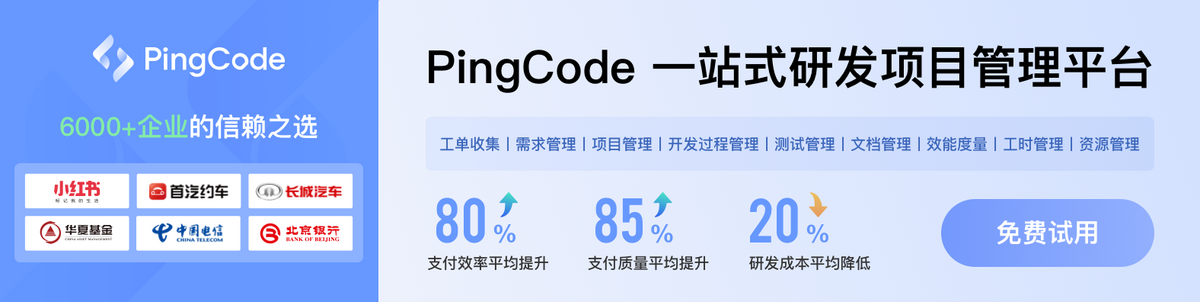