在Python中,输入输出值的方式有多种,包括使用input()函数来获取用户输入、使用print()函数来输出结果、以及使用文件操作进行输入输出。 通过这些方式,可以实现与用户的交互、数据的存储和读取。下面将详细介绍这些方法及其应用。
一、使用 input()
函数获取用户输入
-
基础使用:
input()
函数是Python内置函数,用于从标准输入中读取一行,并将其作为字符串返回。可以通过一个提示字符串来引导用户输入。name = input("Enter your name: ")
print(f"Hello, {name}!")
-
转换数据类型:
用户输入的值默认是字符串类型,若需要其他类型的数据(如整数、浮点数),需进行类型转换。
age = int(input("Enter your age: "))
print(f"Next year, you will be {age + 1} years old.")
-
处理异常输入:
处理用户输入时可能会遇到错误输入,使用try-except块可以捕获异常,确保程序不会因为用户的错误输入而崩溃。
try:
number = int(input("Enter a number: "))
print(f"Twice the number is {number * 2}")
except ValueError:
print("That's not a valid number!")
二、使用 print()
函数输出结果
-
基础使用:
print()
函数用于将指定内容输出到标准输出设备(屏幕)。print("Hello, World!")
-
格式化输出:
可以使用多种方式对输出内容进行格式化,包括使用f-string、
format()
方法和百分号(%)格式化。# Using f-string (Python 3.6+)
name = "Alice"
print(f"Hello, {name}!")
Using format() method
print("Hello, {}!".format(name))
Using % formatting
print("Hello, %s!" % name)
-
控制输出方式:
print()
函数可以通过参数来控制输出方式,如end参数可以指定输出结束时的字符,sep参数可以指定多个值之间的分隔符。print("Hello", "World", sep=", ", end="!\n")
三、文件操作进行输入输出
-
打开文件:
使用
open()
函数可以打开一个文件,指定文件名及操作模式(读取'r'、写入'w'、追加'a'等)。file = open("example.txt", "r")
-
读取文件:
文件对象提供多种方法来读取文件内容,如
read()
、readline()
、readlines()
等。content = file.read()
print(content)
file.close()
-
写入文件:
使用
write()
方法可以将内容写入文件,写入操作完成后要记得关闭文件。file = open("example.txt", "w")
file.write("Hello, World!\n")
file.close()
-
使用 with 语句:
with语句可以自动管理文件的打开和关闭,确保文件操作完成后文件被正确关闭。
with open("example.txt", "r") as file:
content = file.read()
print(content)
四、使用 sys
模块进行输入输出
-
从命令行读取输入:
使用
sys.stdin
可以从标准输入中读取数据。import sys
for line in sys.stdin:
print(line)
-
向标准输出和标准错误输出:
可以使用
sys.stdout
和sys.stderr
分别向标准输出和标准错误输出写入数据。import sys
sys.stdout.write("This is standard output\n")
sys.stderr.write("This is standard error\n")
五、使用 argparse
模块处理命令行参数
-
基础使用:
argparse
模块用于解析命令行参数,便于编写用户友好的命令行接口。import argparse
parser = argparse.ArgumentParser(description="A simple argument parser example")
parser.add_argument("name", type=str, help="Your name")
args = parser.parse_args()
print(f"Hello, {args.name}!")
-
添加可选参数:
可以添加可选参数,使得程序更加灵活。
parser.add_argument("-a", "--age", type=int, help="Your age")
args = parser.parse_args()
if args.age:
print(f"Hello, {args.name}! You are {args.age} years old.")
else:
print(f"Hello, {args.name}!")
六、使用 json
模块进行输入输出
-
读取和写入 JSON 数据:
json
模块用于处理 JSON 数据,提供了简单的接口来解析和生成 JSON 格式的数据。import json
data = {
"name": "Alice",
"age": 25,
"city": "New York"
}
Writing JSON data to a file
with open("data.json", "w") as file:
json.dump(data, file)
Reading JSON data from a file
with open("data.json", "r") as file:
data = json.load(file)
print(data)
七、使用 csv
模块进行输入输出
-
读取和写入 CSV 数据:
csv
模块用于处理 CSV 文件,提供了便捷的接口来读取和写入 CSV 格式的数据。import csv
Writing CSV data to a file
with open("data.csv", "w", newline='') as file:
writer = csv.writer(file)
writer.writerow(["Name", "Age", "City"])
writer.writerow(["Alice", 25, "New York"])
writer.writerow(["Bob", 30, "Los Angeles"])
Reading CSV data from a file
with open("data.csv", "r") as file:
reader = csv.reader(file)
for row in reader:
print(row)
八、使用 pickle
模块进行输入输出
-
序列化和反序列化数据:
pickle
模块用于序列化和反序列化 Python 对象,便于将复杂数据结构保存到文件或通过网络传输。import pickle
data = {
"name": "Alice",
"age": 25,
"city": "New York"
}
Serializing data to a file
with open("data.pkl", "wb") as file:
pickle.dump(data, file)
Deserializing data from a file
with open("data.pkl", "rb") as file:
data = pickle.load(file)
print(data)
九、使用 sqlite3
模块进行输入输出
-
操作 SQLite 数据库:
sqlite3
模块提供了与 SQLite 数据库交互的接口,可以方便地进行数据库操作。import sqlite3
Connecting to a database (or creating it)
conn = sqlite3.connect("example.db")
cursor = conn.cursor()
Creating a table
cursor.execute('''CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)''')
Inserting data into the table
cursor.execute("INSERT INTO users (name, age) VALUES ('Alice', 25)")
conn.commit()
Querying data from the table
cursor.execute("SELECT * FROM users")
rows = cursor.fetchall()
for row in rows:
print(row)
Closing the connection
conn.close()
十、使用 requests
模块进行输入输出
-
发送 HTTP 请求:
requests
模块用于发送 HTTP 请求,可以方便地与 Web 服务进行交互。import requests
Sending a GET request
response = requests.get("https://api.github.com")
print(response.json())
Sending a POST request
data = {"name": "Alice", "age": 25}
response = requests.post("https://httpbin.org/post", json=data)
print(response.json())
十一、使用 subprocess
模块进行输入输出
-
执行系统命令:
subprocess
模块用于执行系统命令,可以捕获命令的输出。import subprocess
Executing a system command
result = subprocess.run(["echo", "Hello, World!"], capture_output=True, text=True)
print(result.stdout)
十二、使用 io
模块进行输入输出
-
操作内存中的文件:
io
模块提供了在内存中操作文件的接口,如StringIO
和BytesIO
。from io import StringIO
Creating an in-memory text stream
text_stream = StringIO()
text_stream.write("Hello, World!\n")
text_stream.write("This is a test.\n")
Reading from the in-memory text stream
text_stream.seek(0)
print(text_stream.read())
通过上述各种方法,可以在Python中灵活地进行输入输出操作,满足不同场景下的需求。无论是与用户交互、文件操作、网络请求还是数据库操作,Python都提供了丰富的工具和模块来实现高效的输入输出。
相关问答FAQs:
在Python中如何读取用户输入的值?
在Python中,您可以使用input()
函数来读取用户输入的值。该函数会暂停程序的执行,等待用户输入,并将输入的内容作为字符串返回。示例代码如下:
user_input = input("请输入一些内容:")
print("您输入的内容是:", user_input)
您可以将输入的字符串转换为其他数据类型,例如整数或浮点数,使用int()
或float()
函数。
如何将输出结果格式化为更易读的形式?
使用print()
函数时,可以通过格式化字符串来提高输出结果的可读性。Python 提供了多种格式化方法,包括使用f-string、format()
方法和百分号(%)格式。以下是使用f-string的示例:
name = "Alice"
age = 30
print(f"{name}的年龄是{age}岁。")
这种方式使得输出内容更加清晰和易于理解。
如何将输出结果保存到文件中?
要将输出结果保存到文件,可以使用Python的文件操作功能。使用open()
函数打开一个文件,并指定写入模式('w'),然后使用write()
方法将内容写入文件。示例代码如下:
with open("output.txt", "w") as file:
file.write("这是要保存的内容。")
使用with
语句确保文件在操作完成后自动关闭,避免资源泄露。
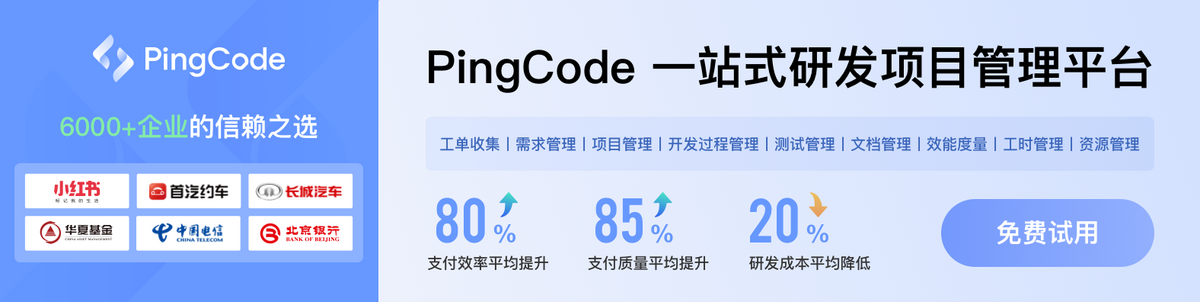