在Python中,你可以使用多种方法来绘制随机粗细的线条。 绘制随机粗细线条的主要方法包括使用Matplotlib库、使用Pygame库、以及使用PIL库。下面将详细介绍如何使用这些方法来绘制随机粗细的线条。
一、使用Matplotlib库
Matplotlib是Python中最常用的绘图库之一,可以非常方便地绘制2D图形。以下是使用Matplotlib库绘制随机粗细线条的步骤:
- 安装Matplotlib库:
pip install matplotlib
- 导入必要的库,并创建一个绘图函数:
import matplotlib.pyplot as plt
import numpy as np
def plot_random_lines(num_lines, xlim, ylim):
for _ in range(num_lines):
x = np.random.uniform(xlim[0], xlim[1], 2)
y = np.random.uniform(ylim[0], ylim[1], 2)
linewidth = np.random.uniform(0.5, 5.0)
plt.plot(x, y, linewidth=linewidth)
plt.xlim(xlim)
plt.ylim(ylim)
plt.show()
调用函数绘制随机线条
plot_random_lines(10, (0, 10), (0, 10))
在这个示例中,plot_random_lines
函数接受三个参数:num_lines
表示要绘制的线条数量,xlim
和 ylim
分别表示x轴和y轴的范围。我们使用 numpy
库生成随机的x和y坐标,并且随机生成线条的粗细。
详细描述:
- 使用
np.random.uniform
函数生成随机的x和y坐标,生成的坐标范围在xlim
和ylim
之间。 - 使用
np.random.uniform
函数生成随机的线条粗细,范围在0.5到5.0之间。 - 使用
plt.plot
函数绘制线条,并设置线条的粗细。 - 最后调用
plt.show
函数显示绘制的图形。
二、使用Pygame库
Pygame是一个跨平台的Python模块,用于编写视频游戏。它包括计算机图形和声音。以下是使用Pygame库绘制随机粗细线条的步骤:
- 安装Pygame库:
pip install pygame
- 导入必要的库,并创建一个绘图函数:
import pygame
import random
def draw_random_lines(screen, num_lines, screen_width, screen_height):
for _ in range(num_lines):
start_pos = (random.randint(0, screen_width), random.randint(0, screen_height))
end_pos = (random.randint(0, screen_width), random.randint(0, screen_height))
width = random.randint(1, 10)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
pygame.draw.line(screen, color, start_pos, end_pos, width)
def main():
pygame.init()
screen_width, screen_height = 800, 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Random Lines")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
draw_random_lines(screen, 10, screen_width, screen_height)
pygame.display.flip()
pygame.quit()
if __name__ == "__main__":
main()
在这个示例中,draw_random_lines
函数接受四个参数:screen
表示Pygame的屏幕对象,num_lines
表示要绘制的线条数量,screen_width
和 screen_height
分别表示屏幕的宽度和高度。我们使用 random
模块生成随机的线条起点和终点坐标,并且随机生成线条的粗细和颜色。
详细描述:
- 使用
random.randint
函数生成随机的起点和终点坐标,生成的坐标范围在屏幕的宽度和高度之间。 - 使用
random.randint
函数生成随机的线条粗细,范围在1到10之间。 - 使用
random.randint
函数生成随机的线条颜色,颜色值在0到255之间。 - 使用
pygame.draw.line
函数绘制线条,并设置线条的颜色、起点、终点和粗细。 - 在主函数中,通过循环不断更新屏幕,并调用
draw_random_lines
函数绘制随机线条。
三、使用PIL库
PIL(Python Imaging Library)是一个强大的图像处理库,可以用于创建和修改图像。以下是使用PIL库绘制随机粗细线条的步骤:
- 安装PIL库:
pip install pillow
- 导入必要的库,并创建一个绘图函数:
from PIL import Image, ImageDraw
import random
def draw_random_lines(image_width, image_height, num_lines):
image = Image.new("RGB", (image_width, image_height), "white")
draw = ImageDraw.Draw(image)
for _ in range(num_lines):
start_pos = (random.randint(0, image_width), random.randint(0, image_height))
end_pos = (random.randint(0, image_width), random.randint(0, image_height))
width = random.randint(1, 10)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
draw.line([start_pos, end_pos], fill=color, width=width)
return image
调用函数绘制随机线条,并保存图像
image = draw_random_lines(800, 600, 10)
image.show()
image.save("random_lines.png")
在这个示例中,draw_random_lines
函数接受三个参数:image_width
和 image_height
分别表示图像的宽度和高度,num_lines
表示要绘制的线条数量。我们使用 random
模块生成随机的线条起点和终点坐标,并且随机生成线条的粗细和颜色。
详细描述:
- 使用
Image.new
函数创建一个新的图像对象,并设置图像的大小和背景颜色。 - 使用
ImageDraw.Draw
函数创建一个绘图对象。 - 使用
random.randint
函数生成随机的起点和终点坐标,生成的坐标范围在图像的宽度和高度之间。 - 使用
random.randint
函数生成随机的线条粗细,范围在1到10之间。 - 使用
random.randint
函数生成随机的线条颜色,颜色值在0到255之间。 - 使用
draw.line
函数绘制线条,并设置线条的颜色、起点、终点和粗细。 - 最后返回生成的图像对象,并使用
image.show
函数显示图像,使用image.save
函数保存图像。
总结
以上三种方法分别使用Matplotlib库、Pygame库和PIL库来绘制随机粗细的线条。每种方法都有其独特的优势和适用场景,选择适合自己需求的方法进行绘制。通过详细的描述和代码示例,相信你已经掌握了如何用Python绘制随机粗细的线条。希望这些内容对你有所帮助!
相关问答FAQs:
如何使用Python绘制随机粗细的线条?
要实现随机粗细的线条,可以使用Python的绘图库,例如Matplotlib。您可以通过设定线条的宽度参数为随机值,来达到这一效果。以下是一个简单的示例代码:
import matplotlib.pyplot as plt
import numpy as np
import random
# 创建一个绘图区域
plt.figure()
# 生成多条随机线条
for _ in range(10):
x = np.linspace(0, 10, 100)
y = np.sin(x) + random.uniform(-1, 1) # 生成随机y值
linewidth = random.uniform(0.5, 5) # 随机线条粗细
plt.plot(x, y, linewidth=linewidth)
plt.show()
这段代码展示了如何生成多条具有不同粗细的正弦波线条。
在绘制随机线条时,有哪些库可以使用?
除了Matplotlib,您还可以使用其他Python绘图库,例如Pygame、Turtle或Seaborn。每个库都有其独特的功能和优势,您可以根据项目的需求选择合适的库。例如,Pygame适合游戏开发,Turtle适合初学者学习编程绘图。
如何控制线条的颜色和样式?
在使用Matplotlib绘制线条时,您可以通过设置颜色和样式参数来改变线条的外观。例如,使用color
参数可以指定线条颜色,linestyle
参数可以设置线条样式(如实线、虚线等)。代码示例如下:
plt.plot(x, y, linewidth=linewidth, color=random.choice(['red', 'blue', 'green']), linestyle=random.choice(['-', '--', ':']))
这样可以让每条线条不仅在粗细上随机,还能在颜色和样式上多样化。
如何在绘图中添加标签和标题?
为了使绘图更具可读性,您可以为每条线条添加标签,并设置整个图形的标题和坐标轴标签。使用plt.title()
、plt.xlabel()
和plt.ylabel()
函数可以实现这些功能。下面是一个示例:
plt.title("随机粗细的线条示例")
plt.xlabel("X 轴")
plt.ylabel("Y 轴")
通过这些操作,您可以使图形更具专业性和易于理解。
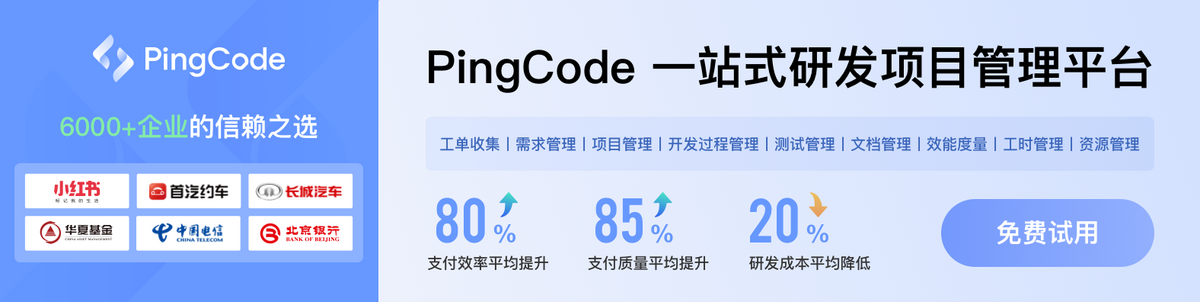