爬取手机通讯录的方法主要包括以下几种:使用Python的ADB(Android Debug Bridge)工具、利用第三方API、编写安卓应用程序、通过Google Contacts API。其中,使用Python的ADB工具是一种比较直接且容易实现的方法。
ADB(Android Debug Bridge)是一个多功能工具,可以帮助开发者与安卓设备进行通信。通过ADB,可以在Python脚本中执行一系列命令,从而获取手机通讯录的数据。下面将详细介绍如何使用Python和ADB工具来爬取手机通讯录。
一、准备工作
-
安装ADB工具:首先需要在计算机上安装ADB工具。可以通过Android SDK来安装ADB工具,也可以直接下载独立的ADB工具包。安装完成后,确保ADB工具的路径已添加到系统的环境变量中。
-
启用安卓设备的开发者模式:在安卓设备上进入设置,找到“关于手机”选项,连续点击“版本号”七次,启用开发者模式。然后进入“开发者选项”,开启“USB调试”功能。
-
连接安卓设备:使用USB数据线将安卓设备连接到计算机,确保设备能够被ADB工具识别。
-
安装Python及相关库:确保计算机上已安装Python环境,并安装ADB的Python库。
二、使用Python和ADB工具获取通讯录
1. 安装ADB的Python库
首先,需要安装adb
库,可以通过pip
进行安装:
pip install adb
2. 编写Python脚本
下面是一个简单的Python脚本,通过ADB工具获取安卓设备上的通讯录数据:
from adb.client import Client as AdbClient
import json
def connect_device():
client = AdbClient(host="127.0.0.1", port=5037)
devices = client.devices()
if len(devices) == 0:
print("No devices connected")
return None
return devices[0]
def get_contacts(device):
# 使用ADB命令获取通讯录数据
result = device.shell("content query --uri content://contacts/phones/ --projection display_name:number")
contacts = result.splitlines()
contacts_list = []
for contact in contacts:
name, number = contact.split("=")[1].split(",", 1)
contacts_list.append({"name": name, "number": number})
return contacts_list
def main():
device = connect_device()
if device:
contacts = get_contacts(device)
with open("contacts.json", "w") as f:
json.dump(contacts, f, indent=4)
print("Contacts saved to contacts.json")
if __name__ == "__main__":
main()
三、详细解析
1. 连接设备
在脚本的connect_device
函数中,通过AdbClient
类连接到本地ADB服务器,并获取已连接的设备列表。如果没有连接设备,脚本会提示“没有设备连接”。
2. 获取通讯录数据
在get_contacts
函数中,使用ADB命令content query
获取通讯录数据。该命令查询安卓设备上的通讯录内容,并使用--projection
选项指定要获取的字段(如display_name
和number
)。通过解析命令的输出,将通讯录数据存储到一个列表中。
3. 保存通讯录数据
在main
函数中,将获取到的通讯录数据保存到一个JSON文件中。这样可以方便地进行后续处理和分析。
四、使用第三方API
除了使用ADB工具外,还可以利用一些第三方API来获取手机通讯录数据。例如,通过Google Contacts API可以访问和管理Google账户中的通讯录数据。
1. 设置Google API项目
首先需要在Google Cloud Platform中创建一个项目,并启用Google Contacts API。然后创建OAuth 2.0凭据,下载客户端密钥文件。
2. 安装Google API客户端库
使用pip
安装Google API客户端库:
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
3. 编写Python脚本
下面是一个使用Google Contacts API获取通讯录数据的示例脚本:
from google.oauth2 import credentials
from google_auth_oauthlib.flow import InstalledAppFlow
from googleapiclient.discovery import build
import json
def get_credentials():
flow = InstalledAppFlow.from_client_secrets_file(
'client_secret.json',
scopes=['https://www.googleapis.com/auth/contacts.readonly']
)
creds = flow.run_local_server(port=0)
return creds
def get_contacts(service):
results = service.people().connections().list(
resourceName='people/me',
pageSize=1000,
personFields='names,phoneNumbers'
).execute()
connections = results.get('connections', [])
contacts_list = []
for person in connections:
names = person.get('names', [])
phone_numbers = person.get('phoneNumbers', [])
if names and phone_numbers:
name = names[0].get('displayName')
number = phone_numbers[0].get('value')
contacts_list.append({"name": name, "number": number})
return contacts_list
def main():
creds = get_credentials()
service = build('people', 'v1', credentials=creds)
contacts = get_contacts(service)
with open("google_contacts.json", "w") as f:
json.dump(contacts, f, indent=4)
print("Contacts saved to google_contacts.json")
if __name__ == "__main__":
main()
五、编写安卓应用程序
另一种方法是编写一个安卓应用程序,获取通讯录数据并将其发送到服务器或存储到文件中。下面是一个简单的安卓应用示例,使用Kotlin语言编写,获取通讯录数据并保存到本地文件。
1. 添加权限
在AndroidManifest.xml
文件中添加读取通讯录和写入存储的权限:
<uses-permission android:name="android.permission.READ_CONTACTS"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
2. 编写代码
在MainActivity.kt
文件中编写代码,获取通讯录数据并保存到文件中:
import android.Manifest
import android.content.pm.PackageManager
import android.os.Bundle
import android.os.Environment
import android.provider.ContactsContract
import androidx.appcompat.app.AppCompatActivity
import androidx.core.app.ActivityCompat
import androidx.core.content.ContextCompat
import org.json.JSONArray
import org.json.JSONObject
import java.io.File
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
if (ContextCompat.checkSelfPermission(this, Manifest.permission.READ_CONTACTS) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, arrayOf(Manifest.permission.READ_CONTACTS), 1)
} else {
getContactsAndSaveToFile()
}
}
override fun onRequestPermissionsResult(requestCode: Int, permissions: Array<out String>, grantResults: IntArray) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults)
if (requestCode == 1 && grantResults.isNotEmpty() && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
getContactsAndSaveToFile()
}
}
private fun getContactsAndSaveToFile() {
val contacts = JSONArray()
val cursor = contentResolver.query(ContactsContract.CommonDataKinds.Phone.CONTENT_URI, null, null, null, null)
while (cursor?.moveToNext() == true) {
val name = cursor.getString(cursor.getColumnIndex(ContactsContract.CommonDataKinds.Phone.DISPLAY_NAME))
val number = cursor.getString(cursor.getColumnIndex(ContactsContract.CommonDataKinds.Phone.NUMBER))
val contact = JSONObject()
contact.put("name", name)
contact.put("number", number)
contacts.put(contact)
}
cursor?.close()
val file = File(Environment.getExternalStorageDirectory(), "contacts.json")
file.writeText(contacts.toString(4))
}
}
六、通过Google Contacts API
Google Contacts API提供了一种访问和管理Google账户中通讯录数据的方式。通过使用Google Contacts API,可以在Python脚本中获取和处理通讯录数据。
1. 设置Google API项目
首先,需要在Google Cloud Platform中创建一个项目,并启用Google Contacts API。然后,创建OAuth 2.0凭据,并下载客户端密钥文件。
2. 安装Google API客户端库
使用pip
安装Google API客户端库:
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
3. 编写Python脚本
下面是一个使用Google Contacts API获取通讯录数据的示例脚本:
from google.oauth2 import credentials
from google_auth_oauthlib.flow import InstalledAppFlow
from googleapiclient.discovery import build
import json
def get_credentials():
flow = InstalledAppFlow.from_client_secrets_file(
'client_secret.json',
scopes=['https://www.googleapis.com/auth/contacts.readonly']
)
creds = flow.run_local_server(port=0)
return creds
def get_contacts(service):
results = service.people().connections().list(
resourceName='people/me',
pageSize=1000,
personFields='names,phoneNumbers'
).execute()
connections = results.get('connections', [])
contacts_list = []
for person in connections:
names = person.get('names', [])
phone_numbers = person.get('phoneNumbers', [])
if names and phone_numbers:
name = names[0].get('displayName')
number = phone_numbers[0].get('value')
contacts_list.append({"name": name, "number": number})
return contacts_list
def main():
creds = get_credentials()
service = build('people', 'v1', credentials=creds)
contacts = get_contacts(service)
with open("google_contacts.json", "w") as f:
json.dump(contacts, f, indent=4)
print("Contacts saved to google_contacts.json")
if __name__ == "__main__":
main()
七、总结
爬取手机通讯录的方法有多种,包括使用Python的ADB工具、利用第三方API、编写安卓应用程序以及通过Google Contacts API等。每种方法都有其优缺点,具体选择哪种方法取决于实际需求和技术背景。
通过以上介绍的方法,可以实现对手机通讯录数据的获取和处理。在实际应用中,需要注意数据隐私和安全问题,确保在合法合规的前提下进行数据操作。同时,定期更新和维护代码,保持与最新技术的同步,也是保障数据获取成功率的重要手段。
相关问答FAQs:
如何使用Python访问手机通讯录中的数据?
要访问手机通讯录数据,您需要使用特定的库和API。对于Android设备,可以考虑使用adb
(Android Debug Bridge)工具,结合Python的subprocess
模块来提取联系人信息。对于iOS设备,则需使用pyobjc
库来调用相关的系统API。确保在使用这些工具之前,手机已开启开发者模式并允许USB调试。
在爬取通讯录时需要注意哪些隐私和安全问题?
在进行通讯录数据爬取时,必须遵循相关法律法规,确保用户隐私得到保护。获取用户同意是非常重要的,未获得同意而爬取数据可能会导致法律责任。此外,存储和使用这些数据时,要采取适当的安全措施,防止数据泄露。
如何处理爬取到的通讯录数据以便进行分析?
一旦成功爬取通讯录数据,可以使用Python的pandas
库进行数据处理和分析。将数据转换为DataFrame格式后,可以方便地进行筛选、排序和统计分析。此外,利用数据可视化工具如matplotlib
或seaborn
,可以将通讯录数据以图表形式呈现,帮助更好地理解数据背后的趋势和模式。
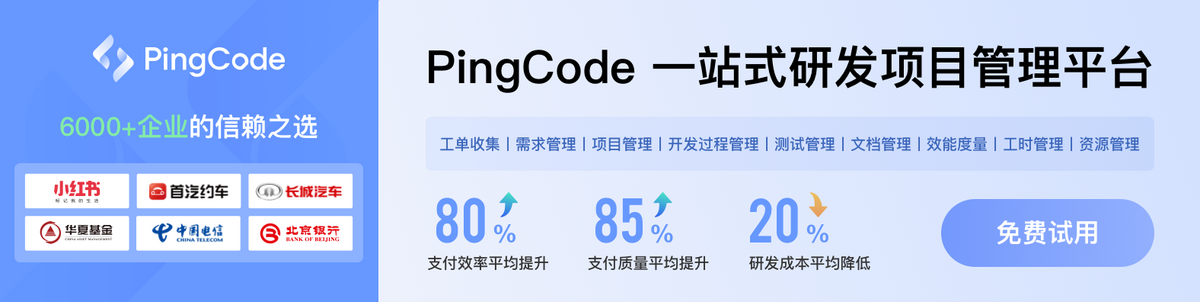