使用Python爬取公众号图片的方法包括:通过微信公众号文章页面的HTML解析、使用第三方库如Selenium和BeautifulSoup、模拟用户行为获取图片链接、使用requests库下载图片。 其中,通过微信公众号文章页面的HTML解析是最常用的方法之一。通过解析HTML页面,可以提取出图片的URL,然后使用requests库进行图片下载。以下是详细介绍。
一、通过微信公众号文章页面的HTML解析
通过微信公众号文章页面的HTML解析,可以提取出图片的URL,然后使用requests库进行图片下载。解析HTML页面通常使用BeautifulSoup库。
1. 安装所需库
首先,确保安装了必要的Python库,如requests和BeautifulSoup:
pip install requests beautifulsoup4
2. 获取微信公众号文章的HTML内容
使用requests库发送HTTP请求获取微信公众号文章的HTML内容:
import requests
url = "微信公众号文章的URL"
response = requests.get(url)
html_content = response.text
3. 解析HTML内容
使用BeautifulSoup解析HTML内容,并提取图片的URL:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, "html.parser")
images = soup.find_all("img")
image_urls = [img["src"] for img in images if "src" in img.attrs]
二、使用Selenium模拟用户行为
有些微信公众号文章需要通过模拟用户行为才能获取到图片,比如需要点击“阅读全文”按钮。Selenium是一个强大的工具,可以模拟浏览器行为。
1. 安装Selenium
首先,安装Selenium库和浏览器驱动(如ChromeDriver):
pip install selenium
下载ChromeDriver并将其添加到系统PATH中。
2. 使用Selenium获取HTML内容
通过Selenium模拟浏览器行为,获取HTML内容:
from selenium import webdriver
url = "微信公众号文章的URL"
driver = webdriver.Chrome()
driver.get(url)
如果有“阅读全文”按钮,需要点击
read_more_button = driver.find_element_by_xpath("button_xpath")
read_more_button.click()
html_content = driver.page_source
driver.quit()
3. 解析HTML内容
同样使用BeautifulSoup解析HTML内容,并提取图片的URL:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, "html.parser")
images = soup.find_all("img")
image_urls = [img["src"] for img in images if "src" in img.attrs]
三、模拟用户行为获取图片链接
有些微信公众号文章需要通过模拟用户行为才能获取到图片,比如需要点击“阅读全文”按钮。可以使用Selenium来模拟这些行为,获取完整的HTML内容。
1. 使用Selenium模拟点击
通过Selenium模拟点击“阅读全文”按钮,获取完整的HTML内容:
from selenium import webdriver
url = "微信公众号文章的URL"
driver = webdriver.Chrome()
driver.get(url)
模拟点击“阅读全文”按钮
read_more_button = driver.find_element_by_xpath("//button[@id='read-more-button']")
read_more_button.click()
获取完整的HTML内容
html_content = driver.page_source
driver.quit()
2. 解析HTML内容
同样使用BeautifulSoup解析HTML内容,并提取图片的URL:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, "html.parser")
images = soup.find_all("img")
image_urls = [img["src"] for img in images if "src" in img.attrs]
四、使用requests库下载图片
获取图片的URL后,可以使用requests库下载图片并保存到本地。
1. 下载图片
使用requests库下载图片,并保存到本地:
import os
import requests
def download_image(url, save_path):
response = requests.get(url, stream=True)
if response.status_code == 200:
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
else:
print(f"Failed to download image from {url}")
创建保存图片的目录
os.makedirs("images", exist_ok=True)
下载所有图片
for index, img_url in enumerate(image_urls):
download_image(img_url, f"images/image_{index}.jpg")
2. 确保下载成功
确保所有图片都能成功下载,并处理下载失败的情况:
import os
import requests
def download_image(url, save_path):
try:
response = requests.get(url, stream=True)
response.raise_for_status()
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
print(f"Image downloaded from {url}")
except Exception as e:
print(f"Failed to download image from {url}: {e}")
创建保存图片的目录
os.makedirs("images", exist_ok=True)
下载所有图片
for index, img_url in enumerate(image_urls):
download_image(img_url, f"images/image_{index}.jpg")
五、处理图片的防盗链问题
有些微信公众号的图片服务器可能会启用防盗链机制,即只有通过特定的Referer才能访问图片。对于这种情况,需要在请求头中加入Referer信息。
1. 设置请求头
在下载图片时,设置请求头中的Referer:
import os
import requests
def download_image(url, save_path, referer):
headers = {
"Referer": referer
}
try:
response = requests.get(url, stream=True, headers=headers)
response.raise_for_status()
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
print(f"Image downloaded from {url}")
except Exception as e:
print(f"Failed to download image from {url}: {e}")
创建保存图片的目录
os.makedirs("images", exist_ok=True)
下载所有图片
for index, img_url in enumerate(image_urls):
download_image(img_url, f"images/image_{index}.jpg", url)
六、使用多线程加快下载速度
如果有大量图片需要下载,可以使用多线程来加快下载速度。
1. 使用ThreadPoolExecutor
使用ThreadPoolExecutor来并行下载图片:
import os
import requests
from concurrent.futures import ThreadPoolExecutor
def download_image(url, save_path, referer):
headers = {
"Referer": referer
}
try:
response = requests.get(url, stream=True, headers=headers)
response.raise_for_status()
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
print(f"Image downloaded from {url}")
except Exception as e:
print(f"Failed to download image from {url}: {e}")
创建保存图片的目录
os.makedirs("images", exist_ok=True)
定义下载任务
def download_task(index, img_url):
download_image(img_url, f"images/image_{index}.jpg", url)
使用ThreadPoolExecutor并行下载图片
with ThreadPoolExecutor(max_workers=10) as executor:
for index, img_url in enumerate(image_urls):
executor.submit(download_task, index, img_url)
七、总结
通过以上方法,可以使用Python爬取微信公众号图片。通过微信公众号文章页面的HTML解析是最常用的方法之一,结合使用requests、BeautifulSoup和Selenium库,可以高效地获取图片链接并下载图片。在处理图片的防盗链问题时,可以通过设置请求头中的Referer来解决。如果有大量图片需要下载,可以使用多线程来加快下载速度。希望这些方法能够帮助到需要爬取微信公众号图片的开发者。
相关问答FAQs:
如何选择合适的Python库进行公众号图片爬取?
在进行公众号图片爬取时,常用的Python库有Requests和BeautifulSoup。Requests用于发送HTTP请求,获取网页内容;而BeautifulSoup则能帮助解析HTML页面,提取所需的图片链接。此外,Scrapy也是一个强大的爬虫框架,适合处理大型数据抓取任务。
爬取公众号图片时需要注意哪些法律和道德问题?
在爬取公众号图片时,务必遵循相关法律法规,尊重版权。未经授权的内容使用可能会侵犯他人权益。在进行爬虫时,可以考虑仅限于个人学习和研究,避免公开分享或商业利用所爬取的内容。同时,合理设置爬虫频率,避免对目标网站造成负担。
如何处理爬取到的图片数据以便于后续使用?
爬取到的图片可以通过Python的PIL库进行处理,比如调整图片大小、格式转换等。也可以将图片保存到本地,或上传到云存储服务以便于后续访问。使用Pandas等数据处理库可以将图片链接与其他数据集成,便于数据分析和可视化。
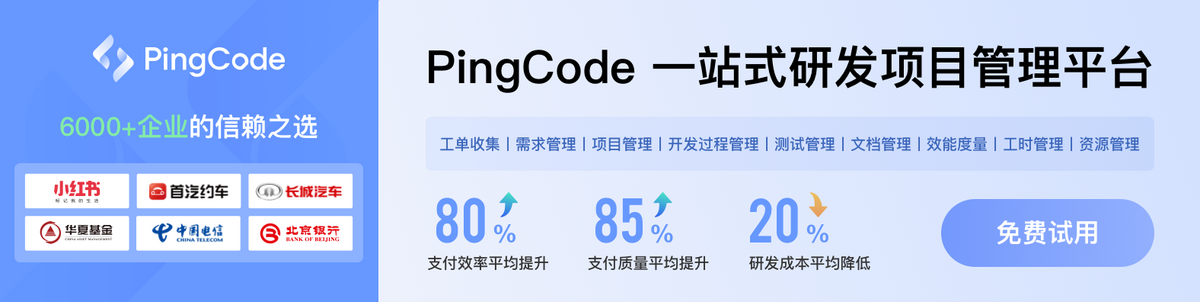