Python循环爬取多页图片的方法包括使用请求库、解析库和循环结构来访问多个网页,解析网页内容并下载图片。 下面详细介绍一种常见的方法,使用requests库进行HTTP请求,BeautifulSoup库解析HTML内容以及os库保存图片。
一、准备工作
在开始之前,你需要确保已经安装了必要的Python库:
pip install requests
pip install beautifulsoup4
二、基础步骤概述
- 发送HTTP请求:使用requests库发送HTTP请求,获取网页的HTML内容。
- 解析HTML内容:使用BeautifulSoup库解析HTML内容,找到图片的URL。
- 保存图片:使用os库保存图片到本地。
- 循环访问多页:通过循环结构访问多个网页。
三、详细步骤讲解
1、发送HTTP请求
首先,我们需要发送HTTP请求,获取网页的HTML内容。以下是一个示例代码:
import requests
url = "http://example.com/page1"
response = requests.get(url)
html_content = response.text
2、解析HTML内容
使用BeautifulSoup库解析HTML内容,找到图片的URL:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
image_tags = soup.find_all('img')
for img_tag in image_tags:
img_url = img_tag['src']
print(img_url)
3、保存图片
将图片保存到本地,使用os库创建目录:
import os
def save_image(img_url, folder_path):
response = requests.get(img_url)
if response.status_code == 200:
with open(os.path.join(folder_path, img_url.split('/')[-1]), 'wb') as file:
file.write(response.content)
创建文件夹
folder_path = 'images'
if not os.path.exists(folder_path):
os.makedirs(folder_path)
保存图片
for img_tag in image_tags:
img_url = img_tag['src']
save_image(img_url, folder_path)
4、循环访问多页
通过循环结构访问多个网页,并重复上述步骤:
base_url = "http://example.com/page"
num_pages = 10
for i in range(1, num_pages + 1):
url = f"{base_url}{i}"
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
image_tags = soup.find_all('img')
for img_tag in image_tags:
img_url = img_tag['src']
save_image(img_url, folder_path)
四、完整示例代码
结合上述步骤,以下是一个完整的示例代码:
import requests
from bs4 import BeautifulSoup
import os
def save_image(img_url, folder_path):
response = requests.get(img_url)
if response.status_code == 200:
with open(os.path.join(folder_path, img_url.split('/')[-1]), 'wb') as file:
file.write(response.content)
def scrape_images(base_url, num_pages, folder_path):
if not os.path.exists(folder_path):
os.makedirs(folder_path)
for i in range(1, num_pages + 1):
url = f"{base_url}{i}"
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
image_tags = soup.find_all('img')
for img_tag in image_tags:
img_url = img_tag['src']
save_image(img_url, folder_path)
使用示例
base_url = "http://example.com/page"
num_pages = 10
folder_path = 'images'
scrape_images(base_url, num_pages, folder_path)
五、注意事项
- 反爬策略:一些网站有反爬策略,可能会封禁频繁的请求。可以使用请求头(headers)模拟浏览器访问。
- URL格式:确保URL格式正确,特别是分页部分。
- 异常处理:添加异常处理,避免程序因网络或其他问题中断。
import requests
from bs4 import BeautifulSoup
import os
import time
def save_image(img_url, folder_path):
response = requests.get(img_url)
if response.status_code == 200:
with open(os.path.join(folder_path, img_url.split('/')[-1]), 'wb') as file:
file.write(response.content)
def scrape_images(base_url, num_pages, folder_path):
if not os.path.exists(folder_path):
os.makedirs(folder_path)
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
for i in range(1, num_pages + 1):
url = f"{base_url}{i}"
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
image_tags = soup.find_all('img')
for img_tag in image_tags:
img_url = img_tag['src']
save_image(img_url, folder_path)
# 延迟一段时间,防止被封禁
time.sleep(2)
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
continue
使用示例
base_url = "http://example.com/page"
num_pages = 10
folder_path = 'images'
scrape_images(base_url, num_pages, folder_path)
通过以上步骤和示例代码,你可以使用Python循环爬取多页图片。根据实际需求,可能需要进行调整和优化。
相关问答FAQs:
如何使用Python实现多页图片的循环爬取?
要实现多页图片的循环爬取,首先需要选择合适的库,例如requests
用于发送网络请求,BeautifulSoup
用于解析HTML内容。可以通过循环构造URL,逐页获取图片链接,并使用requests
下载图片。确保遵循网站的爬虫协议,避免对服务器造成负担。
在爬取图片时,如何处理反爬虫机制?
许多网站会设置反爬虫机制来防止自动化爬取。可以通过设置请求头(如User-Agent
)伪装成浏览器访问,使用随机延时控制请求频率,或者通过代理IP来隐藏真实IP。此外,使用time.sleep()
函数可以有效降低被检测到的风险。
爬取图片后,如何保存和管理下载的文件?
下载的图片可以使用Python的os
库创建文件夹来组织存储。建议根据图片的来源或下载日期命名文件,方便后续管理。还可以使用PIL库对图片进行处理,比如调整大小或格式转换,确保文件符合需要。这样做不仅有助于文件的整理,还能提高使用时的便利性。
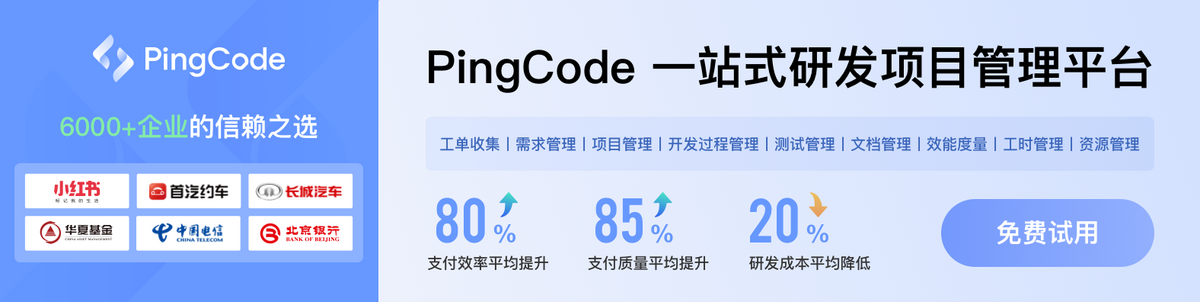