在Python中,可以使用Matplotlib库来绘制柱状图并在柱状图上标注数值。你可以通过调用plt.bar()
函数绘制柱状图,并使用plt.text()
函数在每个柱子上添加数值标注。下面我将详细介绍如何实现这一点,并提供一个完整的示例代码。
一、安装和导入必要的库
在绘制柱状图之前,你需要确保已经安装了Matplotlib库。如果你还没有安装它,可以使用以下命令进行安装:
pip install matplotlib
然后,在你的Python脚本或Jupyter Notebook中导入必要的库:
import matplotlib.pyplot as plt
import numpy as np
二、绘制柱状图
首先,你需要准备数据并绘制柱状图。假设我们有以下数据:
categories = ['A', 'B', 'C', 'D', 'E']
values = [23, 45, 56, 78, 89]
你可以使用plt.bar()
函数来绘制柱状图:
plt.figure(figsize=(10, 6))
bars = plt.bar(categories, values, color='skyblue')
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Chart with Value Labels')
三、在柱状图上标注数值
为了在柱状图上标注数值,可以使用plt.text()
函数。在每个柱子上方添加数值标注。我们可以遍历bars
对象,并使用bar.get_height()
获取每个柱子的高度,然后在适当的位置添加数值标注。
for bar in bars:
height = bar.get_height()
plt.text(bar.get_x() + bar.get_width() / 2.0, height, f'{height}', ha='center', va='bottom')
完整的示例代码如下:
import matplotlib.pyplot as plt
import numpy as np
数据准备
categories = ['A', 'B', 'C', 'D', 'E']
values = [23, 45, 56, 78, 89]
绘制柱状图
plt.figure(figsize=(10, 6))
bars = plt.bar(categories, values, color='skyblue')
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Chart with Value Labels')
在柱状图上标注数值
for bar in bars:
height = bar.get_height()
plt.text(bar.get_x() + bar.get_width() / 2.0, height, f'{height}', ha='center', va='bottom')
显示图表
plt.show()
四、调整标注样式
有时,你可能需要调整标注的样式,以便更好地与图表匹配。例如,你可以更改字体大小、颜色、旋转角度等。可以在plt.text()
函数中添加其他参数:
for bar in bars:
height = bar.get_height()
plt.text(bar.get_x() + bar.get_width() / 2.0, height, f'{height}', ha='center', va='bottom', fontsize=12, color='black', fontweight='bold')
五、处理负值和零值
在处理负值和零值时,添加标注的位置可能需要调整。例如,对于负值,可以将标注放在柱子的下方:
for bar in bars:
height = bar.get_height()
if height < 0:
plt.text(bar.get_x() + bar.get_width() / 2.0, height, f'{height}', ha='center', va='top', fontsize=12, color='red', fontweight='bold')
else:
plt.text(bar.get_x() + bar.get_width() / 2.0, height, f'{height}', ha='center', va='bottom', fontsize=12, color='black', fontweight='bold')
六、自定义柱状图的样式
你还可以进一步自定义柱状图的样式,使其更加美观。例如,可以更改柱子的颜色、添加网格线、调整图表的边距等:
plt.figure(figsize=(12, 8))
bars = plt.bar(categories, values, color=['#FF6347', '#4682B4', '#FFD700', '#ADFF2F', '#FF69B4'])
plt.xlabel('Categories', fontsize=14)
plt.ylabel('Values', fontsize=14)
plt.title('Customized Bar Chart with Value Labels', fontsize=16)
plt.grid(True, linestyle='--', alpha=0.6)
for bar in bars:
height = bar.get_height()
plt.text(bar.get_x() + bar.get_width() / 2.0, height, f'{height}', ha='center', va='bottom', fontsize=12, color='black', fontweight='bold')
plt.tight_layout()
plt.show()
通过上述步骤和示例代码,你应该能够在Python中使用Matplotlib库绘制柱状图并在柱状图上标注数值。根据具体需求,你可以进一步调整和自定义图表的样式。
相关问答FAQs:
如何在Python中使用Matplotlib为柱状图添加数值标签?
在使用Matplotlib绘制柱状图时,可以通过text()
函数在每个柱子上方添加数值标签。首先,绘制柱状图后,遍历每个柱子的高度,使用text()
函数指定位置和显示内容。例如,可以使用以下代码实现:
import matplotlib.pyplot as plt
data = [3, 7, 5, 10]
plt.bar(range(len(data)), data)
for i, v in enumerate(data):
plt.text(i, v + 0.2, str(v), ha='center')
plt.show()
这样,每个柱子上方都会显示对应的数值。
在使用Seaborn绘制的柱状图中如何添加数值标签?
Seaborn是构建在Matplotlib之上的一个可视化库,您可以同样使用text()
函数来添加标签。在绘制完Seaborn的柱状图后,可以通过类似的方式遍历数据并添加数值。例如:
import seaborn as sns
import matplotlib.pyplot as plt
data = [3, 7, 5, 10]
sns.barplot(x=list(range(len(data))), y=data)
for i, v in enumerate(data):
plt.text(i, v + 0.2, str(v), ha='center')
plt.show()
这将为每个柱子添加相应的数值标签。
如何自定义柱状图中数值标签的样式?
自定义数值标签的样式可以通过text()
函数的参数来实现。您可以调整字体大小、颜色和对齐方式等。例如,可以使用以下代码:
for i, v in enumerate(data):
plt.text(i, v + 0.2, str(v), ha='center', fontsize=12, color='blue', fontweight='bold')
通过这些参数,您可以让数值标签更具吸引力和可读性。
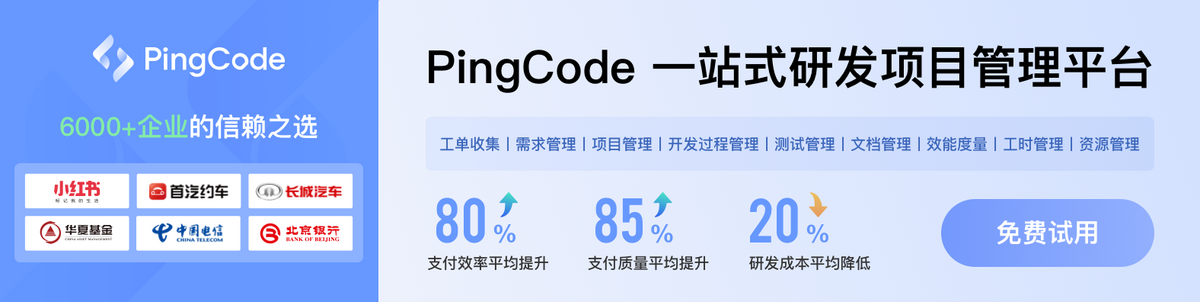