Python开发软件界面窗口的方法有多种,最常见的是使用Tkinter、PyQt、Kivy。这些库各有优缺点,选择合适的库需要根据具体需求。Tkinter简单易用、适合小型应用、PyQt功能强大、适合大型项目、Kivy适合开发跨平台应用。下面详细介绍如何使用这些库来开发软件界面窗口。
一、TKINTER
1、Tkinter概述
Tkinter是Python的标准GUI库,具有轻量级、易用、跨平台等特点。它提供了常见的窗口控件,如按钮、标签、文本框等,适合快速开发小型应用。
2、安装与基本使用
Tkinter是Python自带库,无需额外安装。可以通过以下代码创建一个简单的窗口:
import tkinter as tk
创建主窗口
root = tk.Tk()
root.title("Tkinter 窗口")
创建标签
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
运行主循环
root.mainloop()
3、窗口布局
Tkinter提供了多种布局管理器,如pack、grid、place。pack是最简单的一种,适合基本布局;grid适合网格布局;place可以自由定位控件。
# 使用grid布局
label1 = tk.Label(root, text="Label 1")
label2 = tk.Label(root, text="Label 2")
label1.grid(row=0, column=0)
label2.grid(row=1, column=1)
4、事件处理
Tkinter支持事件驱动,可以通过绑定事件来响应用户操作。例如,绑定按钮点击事件:
def on_button_click():
print("Button clicked!")
button = tk.Button(root, text="Click Me", command=on_button_click)
button.pack()
二、PYQT
1、PyQt概述
PyQt是一个功能强大的Python GUI库,基于Qt库,适合开发复杂的桌面应用。它提供了丰富的控件和强大的功能,如信号与槽机制、样式表等。
2、安装与基本使用
可以使用pip安装PyQt:
pip install PyQt5
创建一个简单的窗口:
from PyQt5.QtWidgets import QApplication, QWidget, QLabel
app = QApplication([])
window = QWidget()
window.setWindowTitle("PyQt 窗口")
label = QLabel("Hello, PyQt!", parent=window)
window.show()
app.exec_()
3、窗口布局
PyQt提供了多种布局管理器,如QVBoxLayout、QHBoxLayout、QGridLayout。可以通过以下代码实现布局管理:
from PyQt5.QtWidgets import QVBoxLayout, QPushButton
layout = QVBoxLayout()
button1 = QPushButton("Button 1")
button2 = QPushButton("Button 2")
layout.addWidget(button1)
layout.addWidget(button2)
window.setLayout(layout)
4、信号与槽
PyQt的信号与槽机制是其强大功能之一,可以方便地处理事件。例如,绑定按钮点击事件:
def on_button_click():
print("Button clicked!")
button = QPushButton("Click Me")
button.clicked.connect(on_button_click)
layout.addWidget(button)
三、KIVY
1、Kivy概述
Kivy是一个开源的Python库,适合开发多点触控应用和跨平台应用。它具有高度定制化的用户界面,支持多种输入设备。
2、安装与基本使用
可以使用pip安装Kivy:
pip install kivy
创建一个简单的窗口:
from kivy.app import App
from kivy.uix.label import Label
class MyApp(App):
def build(self):
return Label(text="Hello, Kivy!")
if __name__ == "__main__":
MyApp().run()
3、窗口布局
Kivy提供了多种布局控件,如BoxLayout、GridLayout、FloatLayout。可以通过以下代码实现布局管理:
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.button import Button
class MyApp(App):
def build(self):
layout = BoxLayout(orientation='vertical')
button1 = Button(text="Button 1")
button2 = Button(text="Button 2")
layout.add_widget(button1)
layout.add_widget(button2)
return layout
4、事件处理
Kivy使用事件调度器来处理事件。例如,绑定按钮点击事件:
def on_button_click(instance):
print("Button clicked!")
button = Button(text="Click Me")
button.bind(on_press=on_button_click)
layout.add_widget(button)
四、选择适合的库
1、根据项目需求选择
选择适合的库需要根据具体需求。如果需要快速开发小型应用,可以选择Tkinter;如果需要开发功能复杂的桌面应用,可以选择PyQt;如果需要开发跨平台应用,可以选择Kivy。
2、考虑学习成本
学习成本也是选择库的重要因素。Tkinter入门简单,适合初学者;PyQt功能强大,但学习曲线较陡;Kivy适合有一定编程经验的开发者。
3、社区与文档支持
社区与文档支持也是选择库的重要因素。Tkinter、PyQt、Kivy都有较好的社区和文档,可以提供丰富的学习资源和技术支持。
五、综合示例
1、Tkinter示例
下面是一个综合示例,展示如何使用Tkinter创建一个简单的记事本应用:
import tkinter as tk
from tkinter import filedialog
def new_file():
text.delete(1.0, tk.END)
def open_file():
file_path = filedialog.askopenfilename()
with open(file_path, 'r') as file:
content = file.read()
text.delete(1.0, tk.END)
text.insert(tk.END, content)
def save_file():
file_path = filedialog.asksaveasfilename()
with open(file_path, 'w') as file:
content = text.get(1.0, tk.END)
file.write(content)
root = tk.Tk()
root.title("记事本")
text = tk.Text(root)
text.pack()
menu = tk.Menu(root)
root.config(menu=menu)
file_menu = tk.Menu(menu)
menu.add_cascade(label="文件", menu=file_menu)
file_menu.add_command(label="新建", command=new_file)
file_menu.add_command(label="打开", command=open_file)
file_menu.add_command(label="保存", command=save_file)
root.mainloop()
2、PyQt示例
下面是一个综合示例,展示如何使用PyQt创建一个简单的记事本应用:
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit, QAction, QFileDialog
class Notepad(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.text = QTextEdit(self)
self.setCentralWidget(self.text)
new_action = QAction('新建', self)
new_action.triggered.connect(self.new_file)
open_action = QAction('打开', self)
open_action.triggered.connect(self.open_file)
save_action = QAction('保存', self)
save_action.triggered.connect(self.save_file)
menubar = self.menuBar()
file_menu = menubar.addMenu('文件')
file_menu.addAction(new_action)
file_menu.addAction(open_action)
file_menu.addAction(save_action)
self.setGeometry(300, 300, 800, 600)
self.setWindowTitle('记事本')
self.show()
def new_file(self):
self.text.clear()
def open_file(self):
file_path, _ = QFileDialog.getOpenFileName(self, '打开文件')
with open(file_path, 'r') as file:
content = file.read()
self.text.setText(content)
def save_file(self):
file_path, _ = QFileDialog.getSaveFileName(self, '保存文件')
with open(file_path, 'w') as file:
content = self.text.toPlainText()
file.write(content)
if __name__ == '__main__':
app = QApplication([])
notepad = Notepad()
app.exec_()
3、Kivy示例
下面是一个综合示例,展示如何使用Kivy创建一个简单的记事本应用:
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.textinput import TextInput
from kivy.uix.button import Button
from kivy.uix.filechooser import FileChooserListView
from kivy.uix.popup import Popup
class Notepad(App):
def build(self):
self.layout = BoxLayout(orientation='vertical')
self.text = TextInput()
self.layout.add_widget(self.text)
button_layout = BoxLayout(size_hint_y=None, height=50)
new_button = Button(text='新建')
new_button.bind(on_press=self.new_file)
open_button = Button(text='打开')
open_button.bind(on_press=self.open_file)
save_button = Button(text='保存')
save_button.bind(on_press=self.save_file)
button_layout.add_widget(new_button)
button_layout.add_widget(open_button)
button_layout.add_widget(save_button)
self.layout.add_widget(button_layout)
return self.layout
def new_file(self, instance):
self.text.text = ''
def open_file(self, instance):
content = FileChooserListView(on_submit=self.load_file)
self.popup = Popup(title='打开文件', content=content, size_hint=(0.9, 0.9))
self.popup.open()
def load_file(self, chooser, selection, touch):
with open(selection[0], 'r') as file:
self.text.text = file.read()
self.popup.dismiss()
def save_file(self, instance):
content = FileChooserListView(on_submit=self.write_file)
self.popup = Popup(title='保存文件', content=content, size_hint=(0.9, 0.9))
self.popup.open()
def write_file(self, chooser, selection, touch):
with open(selection[0], 'w') as file:
file.write(self.text.text)
self.popup.dismiss()
if __name__ == '__main__':
Notepad().run()
六、总结
Python提供了多种开发软件界面窗口的库,每种库都有其独特的优势和适用场景。Tkinter简单易用、适合小型应用、PyQt功能强大、适合大型项目、Kivy适合开发跨平台应用。根据项目需求选择合适的库,能够提高开发效率,满足不同的开发需求。
相关问答FAQs:
如何使用Python创建图形用户界面(GUI)?
Python提供了多种库来开发图形用户界面,最常用的是Tkinter、PyQt和wxPython。Tkinter是Python内置的库,适合初学者使用。PyQt则功能强大,适合需要复杂界面的应用,而wxPython则提供了原生的外观和感觉。根据项目需求选择合适的库,可以利用这些库的文档和示例代码加速开发过程。
Python开发软件界面需要哪些基础知识?
在开始开发GUI之前,掌握Python编程的基本概念是非常重要的。此外,了解事件驱动编程、布局管理、组件使用和用户交互等概念也至关重要。熟悉相关库的API文档和示例代码能帮助你更快上手,设计出用户友好的界面。
如何调试和优化Python GUI应用程序的性能?
调试Python GUI应用程序可以通过使用Python内置的调试工具(如pdb)和IDE的调试功能进行。在性能优化方面,关注界面响应速度和资源使用情况是关键。使用线程或异步编程可以改善界面的响应能力,避免在主线程中执行耗时操作。同时,定期检查和更新库版本,以利用最新的性能优化和功能增强。
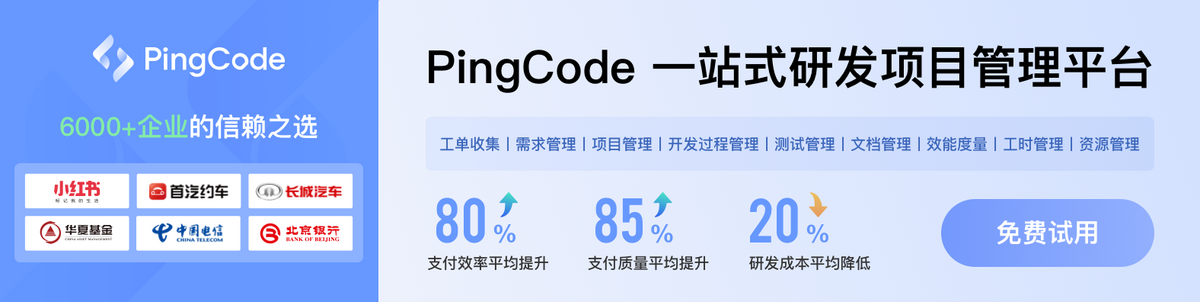